The new feature that appeals to me is the new “Web API” which eases the development of REST and AJAX APIs (API is the new Microsoft jargon for a web service!)
Wanting to have a play with this new feature, I fired up Visual Studio Express 2012 and created a standard web application in vb.net. Although many examples on the web are showing the Web API with MVC, it can be used in any .net application.
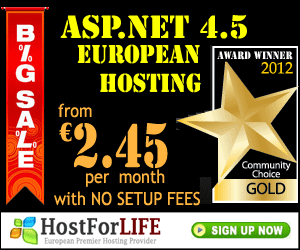
Using the new “Web API” requires two parts.
1. Setup the controller class that will perform the logic.
2. Setup up route tables in your global.asax.vb file, to correctly “route” the client request to the correct controller class.
So down to code. The examples below assume a GET request to the Web API Server.
1. Create a standard web application, remembering to specify .net 4.5 as the target framework.
2. Create a new folder within the application called “Controllers”. See Picture below.
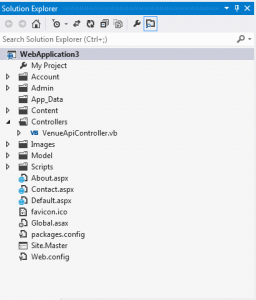
3. Within this folder, create a new “Web API Controller Class”, by right clicking on the Controllers folder and clicking Add. Then choose “Web API Controller Class” from the list as shown below
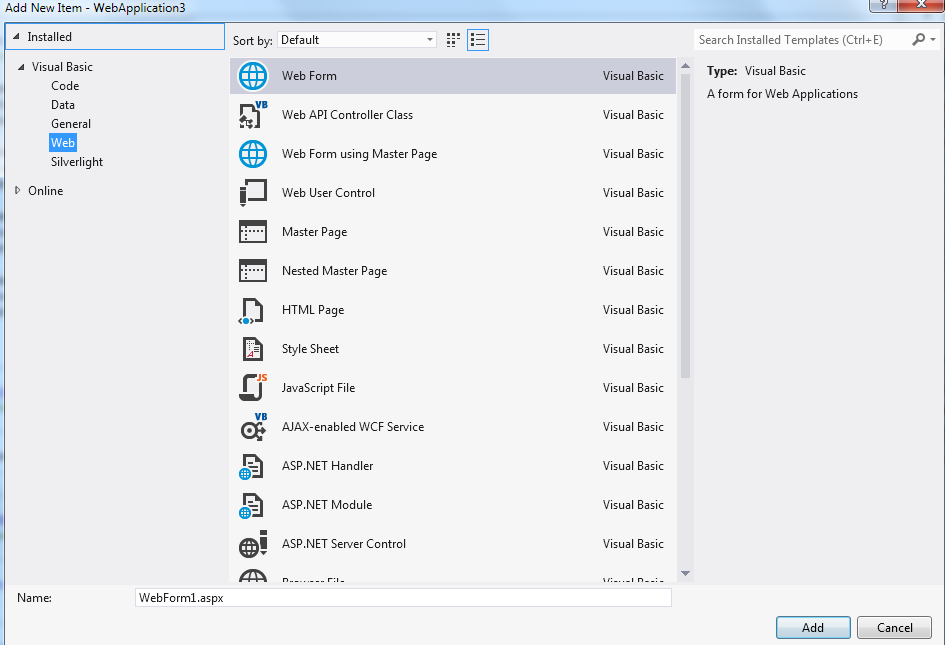
4. When naming this new controller class, remember to leave the word controller at the end of the name, otherwise the routing won’t work! As you can see below, I called my class VenueApiController.vb
5. When you open this new class, you will see some get, post,delete functions already filled in. I just created new functions to suit my client data requirements.
The sample data that I am using is for a project we have been working on call nejola.com. This allows local businesses to “push” their deals to Android smartphone users in the locality.
We have some test data in the SQL Server, so used this to try the Web API out.
The first function that I created returned a full list of the test venues available. I named this function GetVenues. No search data was needed to be passed to the function and I wanted the result fetched as a dataset to the client. The beauty of the “Web API” is the data is then presented to the client depending upon the headers of the calling client. For example an ajax call will return the data in a json format
Public Function GetVenues() As DataSet
Try
Using sqlConn As New
SqlConnection(ConfigurationManager.ConnectionStrings("DefaultConnection").ConnectionString)
Dim sqlComm As SqlCommand = New SqlCommand
sqlComm.Connection = sqlConn
sqlComm.CommandText = "SELECT * From df_Locations"
Dim sqlDataAdapt As SqlDataAdapter = New SqlDataAdapter(sqlComm)
Dim ds As DataSet = New DataSet
sqlDataAdapt.Fill(ds)
sqlDataAdapt.Dispose() : sqlComm.Dispose() : sqlConn.Close() : sqlConn.Dispose()
ds.DataSetName = "VenueData"
ds.Tables(0).TableName = "exgVenuesData"
Return ds
End Using
Catch ex As Exception
Return Nothing
End Try
And that’s it for the data logic ! Nothing too fancy here, just perform some SQL on the SQL server and return the dataset. I would normally call a stored procedure for but this purpose used the commandtext
The function below requires a parameter to be passed, in this case the town name as a string.
Public Function GetVenueByTown(ByVal town As String) As DataSet
Dim sqlConn As SqlConnection = New SqlConnection("Connection info here")
sqlConn.Open()
Dim sqlComm As SqlCommand = New SqlCommand()
sqlComm.Connection = sqlConn
sqlComm.CommandText = "SELECT * From df_Locations WHERE VenueTown = '" & town & "'"
Dim sqlDataAdapt As SqlDataAdapter = New SqlDataAdapter(sqlComm)
Dim ds As DataSet = New DataSet
sqlDataAdapt.Fill(ds)
sqlDataAdapt.Dispose() : sqlComm.Dispose() : sqlConn.Close() : sqlConn.Dispose()
ds.DataSetName = "VenueTownData"
ds.Tables(0).TableName = "EXGTownData"
Return ds
End Function
Now that we have two working functions within the API Class, we need to add routing to call the functions. Users Of Microsoft MVC should be aware of routing and controllers, however for VB.net users this is new with .net 4.5
What the routing and controllers allows us to do is present the URL’s called by the clients in a nice format. For example to call the GetVenues function above, the client can call :
http://domain.com/venues
To call the GetVenueByTown function and pass the town parameter the client can use :
http://domain.com/venues/town/townname
Ok, so now we need to setup the routing for this to work !
1. Open the global.asax.vb file and look for the sub
Sub Application_Start(ByVal sender As Object, ByVal e As EventArgs)
End sub
2. At the top of the page add :
Imports System.Web.Routing
Imports System.Web.Http
3. Here is the code for the GetVenues Function which is the default function called. Add this to the Application_Start Sub.
RouteTable.Routes.MapHttpRoute(name:="Venue", _
routeTemplate:="venues", _
defaults:=New With {.symbol = RouteParameter.Optional, .controller = "VenueApi"})
To break down the above, here is what is happening. This example is the default call, with no parameters being passed to the function.
name:=”Venue” –> This is the name given to this routetable. Each routetable has a different name.
routeTemplate:=”venues” –> This is the actual name that the client will call. In this example http://domain.com/venues.
.controller = “VenueApi” –> This MUST match the name that you gave your controller class without the controller part.
The route code to call the GetVenueByTown function that requires a town parameter to be passed is as follows :
RouteTable.Routes.MapHttpRoute(name:="VenueTowns", _
routeTemplate:="venues/town/{town}", _
defaults:=New With {.symbol = RouteParameter.Optional, .controller = "VenueApi"})
Again a break down of the code is as follows :
name:=”VenueTowns” –> Unique name of the routetable.
routeTemplate:=”venues/town/{town}” –> This is the path that will be entered by the client to get to the correct function. Note we have added the /town/ path and the parameter {town}. This parameter name must match that of the function otherwise the routing won’t work correctly.
controller = “VenueApi” –> This is the same as before and is the controller class without the controller part.