In this post, I will tell you about how to handling JSON Arrays returned from ASP.NET Web Services with jQuery. JSON (JavaScript Object Notation) is a lightweight data-interchange format. It is easy for humans to read and write. It is easy for machines to parse and generate. It is based on a subset of the JavaScript Programming Language, Standard ECMA-262 3rd Edition - December 1999. JSON is a text format that is completely language independent but uses conventions that are familiar to programmers of the C-family of languages, including C, C++, C#, Java, JavaScript, Perl, Python, and many others. These properties make JSON an ideal data-interchange language.
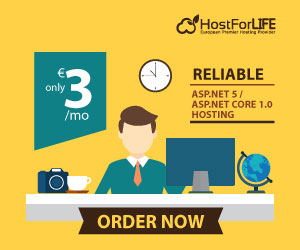
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Data;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
using System.Web;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.Services;
namespace VIS {
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
[System.Web.Script.Services.ScriptService]
public class WebMyService: System.Web.Services.WebService {
string connetionString = null;
SqlConnection sqlCnn;
SqlCommand sqlCmd;
SqlDataAdapter adapter = new SqlDataAdapter();
DataSet dsbind = new DataSet();
int i = 0;
string sql = null;
public class Gender {
public string employeeid {
get;
set;
}
public string male {
get;
set;
}
public string female {
get;
set;
}
}
public string JSONConversion(DataTable dt) {
DataSet ds = new DataSet();
ds.Merge(dt);
StringBuilder JsonString = new StringBuilder();
JsonString.Append("{");
JsonString.Append("\"Data\"");
JsonString.Append(":");
if (ds != null && ds.Tables[0].Rows.Count > 0) {
JsonString.Append("[");
for (int i = 0; i < ds.Tables[0].Rows.Count; i++) {
JsonString.Append("{");
for (int j = 0; j < ds.Tables[0].Columns.Count; j++) {
if (j < ds.Tables[0].Columns.Count - 1) {
JsonString.Append("\"" + ds.Tables[0].Columns[j].ColumnName.ToString() + "\":" + "\"" + ds.Tables[0].Rows[i][j].ToString() + "\",");
} else if (j == ds.Tables[0].Columns.Count - 1) {
JsonString.Append("\"" + ds.Tables[0].Columns[j].ColumnName.ToString() + "\":" + "\"" + ds.Tables[0].Rows[i][j].ToString() + "\"");
}
}
if (i == ds.Tables[0].Rows.Count - 1) {
JsonString.Append("}");
} else {
JsonString.Append("},");
}
}
JsonString.Append("]");
JsonString.Append("}");
return JsonString.ToString();
} else {
return null;
}
}
[WebMethod]
[ScriptMethod(ResponseFormat = ResponseFormat.Json)]
public Gender[] GenderWise() {
connetionString = "Data Source=ServerName;Initial Catalog=DatabaseName;User ID=UserName;Password=Password";
sql = "select distinct(empid) as employeeid, count(case when gender='M' then 1 end) as Male, count(case when gender='F' then 1 end) as Female from V_CountOnGender";
sqlCnn = new SqlConnection(connetionString);
try {
sqlCnn.Open();
sqlCmd = new SqlCommand(sql, sqlCnn);
adapter.SelectCommand = sqlCmd;
adapter.Fill(dsbind);
JavaScriptSerializer obj = new JavaScriptSerializer();
string result = string.Empty;
Gender[] arrlst = new Gender[dsbind.Tables[0].Rows.Count];
if (dsbind.Tables[0].Rows.Count > 0) {
for (int i = 0; i < dsbind.Tables[0].Rows.Count; i++) {
Gender objgender = new Gender();
objgender.employeeid = dsbind.Tables[0].Rows[i]["employeeid"].ToString();
objgender.male = dsbind.Tables[0].Rows[i]["Male"].ToString();
objgender.female = dsbind.Tables[0].Rows[i]["Female"].ToString();
arrlst.SetValue(objgender, i);
}
} else {
result = "No Record Found";
}
} catch (Exception ex) {}
return arrlst;;
}
}
}
This will go into the < head > section of the page:
<
script type = "text/javascript"
src = "script/jquery-1.2.6.min.js" > < /script> <
script type = "text/javascript" >
$(document).ready(function() {
$.ajax({
type: "POST",
contentType: "application/json; charset=utf-8",
dataType: "json",
url: "/WebMyVoterService.asmx/GenderWise",
processData: false,
success: OnSuccess,
failure: function(response) {
alert("Can't be able to bind graph");
},
error: function(response) {
alert("Can't be able to bind graph");
}
});
function OnSuccess(response) {
var dpmale = [];
var dpfemale = [];
for (var i = 0; i < response.d.length; i++) {
var obj = response.d[i];
var datamale = {
y: parseInt(obj.male),
label: obj.employeeid,
};
var datafemale = {
y: parseInt(obj.female),
label: obj.employeeid,
};
dpmale.push(datamale);
dpfemale.push(datafemale);
}
var chart = new CanvasJS.Chart("chartContainerbar", {
animationEnabled: true,
axisX: {
interval: 1,
labelFontSize: 10,
lineThickness: 0,
},
axisY2: {
valueFormatString: "0",
lineThickness: 0,
labelFontSize: 10,
},
toolTip: {
shared: true
},
legend: {
verticalAlign: "top",
horizontalAlign: "center",
fontSize: 10,
},
data: [{
type: "stackedBar",
showInLegend: true,
name: "Male",
axisYType: "secondary",
color: "#f8d347",
dataPoints: dpmale
}, {
type: "stackedBar",
showInLegend: true,
name: "Female",
axisYType: "secondary",
color: "#6ccac9",
dataPoints: dpfemale
}]
});
chart.render();
}
}); < /script>
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
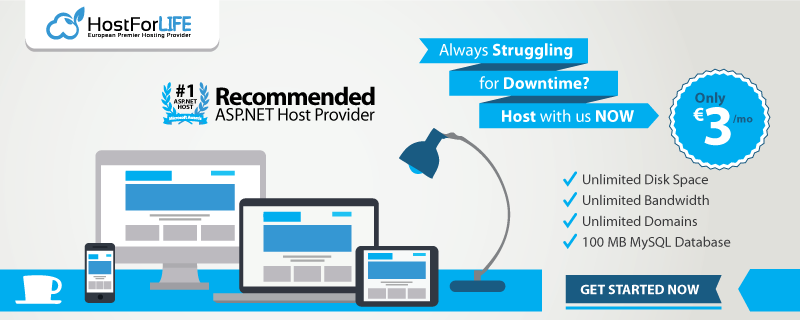