Once you finish developing an ASP.NET Core web application, you need to deploy it on a server so that end users can start using it. When it comes to deployment to IIS, ASP.NET Core offers two hosting models namely InProcess and OutOfProcess. In this article you learn about these hosting models and how to configure them.
When you deploy your web application to IIS, various requests to the application are handled by what is known as ASP.NET Core Module. Under default settings the hosting model for your application is InProcess. This means ASP.NET Core Module forwards the requests to IIS HTTP Server (IISHttpServer). The IIS HTTP Server is a server that runs in-process with IIS. This results in great performance as compared to Out Of Process model. In-process models bypasses built-in Kestrel web server of ASP.NET Core.
If you decide to use Out-Of-Process hosting model then IIS HTTP Server won't be used. Instead Kestrel web server is used to process your requests. So, ASP.NET Core Module forwards your requests to Kestrel web server. This communication is out-of-process communication and is therefore slower than the in-process model.
Now that you have some basic idea about the in-process and out-of-process hosting models let's see how to configure them.
In order to understand the hosting models discussed in this article you first need to create an ASP.NET Core web application using Visual Studio. So, go ahead and do so based on any of the web application project templates. Make sure to use ASP.NET Core version 2.2 or later.
Once you create the project, click on the Build > Publish menu and Web Deploy the output (or manually copy to IIS) to IIS. Once you finish deploying the application locate the web.config file generated during the deployment process. In this web.config you will find a section like this:
<system.webServer>
<handlers>
<add name="aspNetCore" path="*" verb="*"
modules="AspNetCoreModuleV2" />
</handlers>
<aspNetCore processPath="dotnet"
arguments=".\MyWebApp.dll"
stdoutLogEnabled="false"
stdoutLogFile=".\logs\stdout"
hostingModel="inprocess" />
</system.webServer>
As you can see the <aspNetCore> tag has hostingModel attribute that is set to inprocess by default.
Now, run the application from the browser with this default setting in place and observe the HTTP headers. The following figure shows one such sample run of the application.
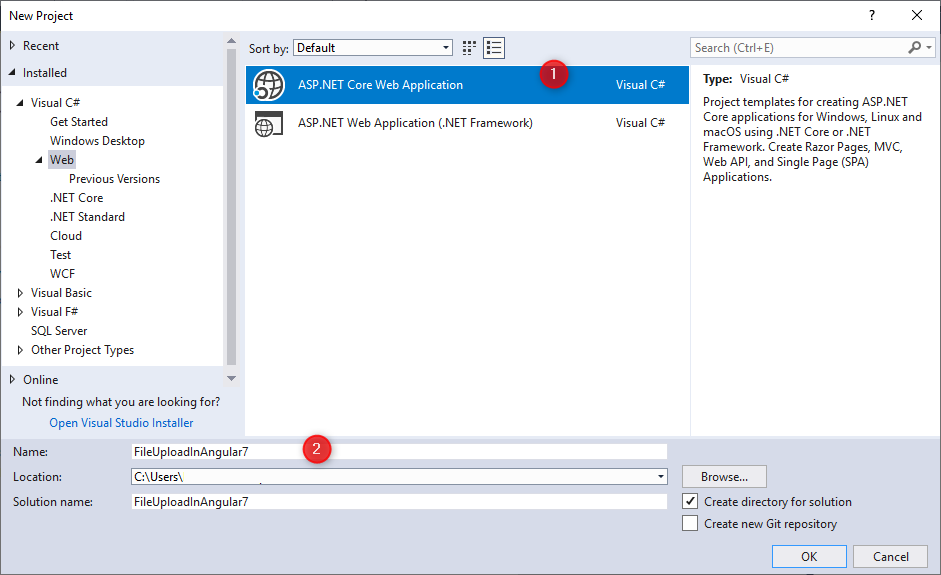
As you can see the Server is Microsoft IIS.
Now, change the hostingModel attribute from inprocess to outofprocess. Run the application again. This time you will get the following output:
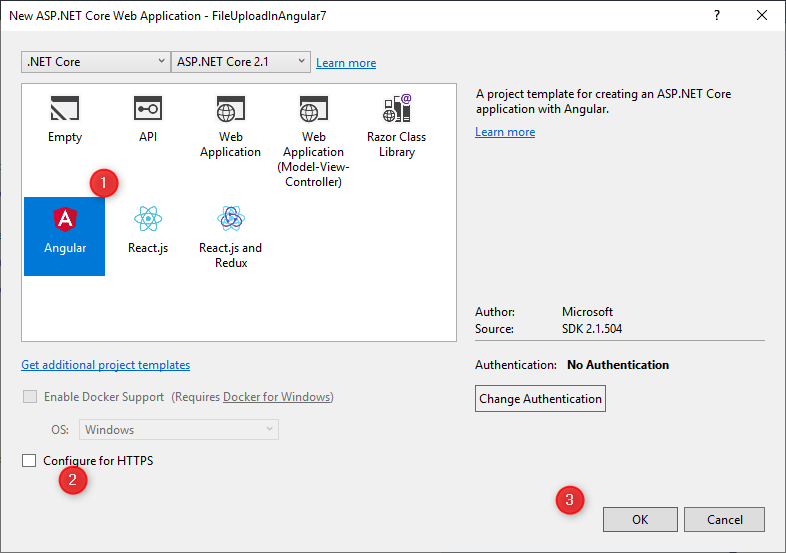
As you can see the Server is now Kestrel indicating that Out-Of-Process model is active.
In the preceding example you change the hosting model in the web.config generated during the publish operation. You can also specify the hosting model in project's .csproj file. Consider the following markup from .csproj file that does that.
<PropertyGroup>
<TargetFramework>netcoreapp2.2</TargetFramework>
<AspNetCoreHostingModel>InProcess</AspNetCoreHostingModel>
</PropertyGroup>
The <AspNetCoreHostingModel> element sets the hosting model to InProcess. To set it to Out-Of-Process you need to set it like this:
<AspNetCoreHostingModel>OutOfProcess</AspNetCoreHostingModel>
Run the application with these settings and confirm whether you get the outcome as before.
That's it for now! Keep coding!!