Performance is very important; it is a major factor for the success of any web application. ASP.NET Core 3.0 includes several enhancements that scale back memory usage and improve turnout. In this blog post, I provide 10 tips to help you improve the performance of ASP.NET Core 3.0 applications by doing the following:
Avoid synchronous and use asynchronous
Try to avoid synchronous calling when developing ASP.NET Core 3.0 applications. Synchronous calling blocks the next execution until the current execution is completed. While fetching data from an API or performing operations like I/O operations or independent calling, execute the call in an asynchronous manner.
Avoid using Task.Wait and Task.Result, and try to use await. The following code shows how to do this.
public class WebHost
{
public virtual async Task StartAsync(CancellationToken cancellationToken = default)
{
// Fire IHostedService.Start
await _hostedServiceExecutor.StartAsync(cancellationToken).ConfigureAwait(false);
// More setup
await Server.StartAsync(hostingApp, cancellationToken).ConfigureAwait(false);
// Fire IApplicationLifetime.Started
_applicationLifetime?.NotifyStarted();
// Remaining setup
}
}
Entity Framework 3.0 Core also provides a set of async extension methods, similar to LINQ methods, that execute a query and return results.
Asynchronous querying
Asynchronous queries avoid blocking a thread while the query is executed in the database. Async queries are important for quick, responsive client applications.
Examples:
-
ToListAsync()
-
ToArrayAsync()
-
SingleAsync()
public async Task<List> GetBlogsAsync()
{
using (var context = new BloggingContext())
{
return await context.Blogs.ToListAsync();
}
}
Asynchronous saving
Asynchronous saving avoids a thread block while changes are written to the database. It provides DbContext.SaveChangesAsync() as an asynchronous alternative to DbContext.SaveChanges().
public static async Task AddBlogAsync(string url)
{
using (var context = new BloggingContext())
{
var blogContent = new BlogContent { Url = url };
context.Blogs.Add(blogContent);
await context.SaveChangesAsync();
}
}
Optimize data access
Improve the performance of an application by optimizing its data access logic. Most applications are totally dependent on a database. They have to fetch data from the database, process the data, and then display it. If it is time-consuming, then the application will take much more time to load.
Recommendations:
-
Call all data access APIs asynchronously.
-
Don’t try to get data that is not required in advance.
-
Try to use no-tracking queries in Entity Framework Core when accessing data for read-only purposes.
-
Use filter and aggregate LINQ queries (with .Where, .Select, or .Sum statements), so filtering can be performed by the database.
You can find approaches that may improve performance of your high-scale apps in the new features of EF Core 3.0.
Use response caching middleware
Middleware controls when responses are cacheable. It stores responses and serves them from the cache. It is available in the Microsoft.AspNetCore.ResponseCaching package, which was implicitly added to ASP.NET Core.
In Startup.ConfigureServices, add the Response Caching Middleware to the service collection.
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCaching();
services.AddRazorPages();
}
Use JSON serialization
ASP.NET Core 3.0 uses System.Text.Json for JSON serialization by default. Now, you can read and write JSON asynchronously. This improves performance better than Newtonsoft.Json. The System.Text.Json namespace provides the following features for processing JSON:
Reduce HTTP requests
Reducing the number of HTTP requests is one of the major optimizations. Cache the webpages and avoid client-side redirects to reduce the number of connections made to the web server.
Use the following techniques to reduce the HTTP requests:
-
Use minification.
-
Use bundling.
-
Use sprite images.
By reducing HTTP requests, these techniques help pages load faster.
Use exceptions only when necessary
Exceptions should be rare. Throwing and catching exceptions will consume more time relative to other code flow patterns.
Use response compression
Response compression, which compresses the size of a file, is another factor in improving performance. In ASP.NET Core, response compression is available as a middleware component.
Usually, responses are not natively compressed. This typically includes CSS, JavaScript, HTML, XML, and JSON.
-
Don’t compress natively compressed assets, such as PNG files.
-
Don’t compress files with a size of 150-1,000 bytes.
-
Don’t compress small files; it may produce a compressed file larger than the uncompressed file.
Package: Microsoft.AspNetCore.ResponseCompression is implicitly included in ASP.NET Core apps.
The following sample code shows how to enable Response Compression Middleware for the default MIME types and compression providers.
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseResponseCompression();
}
}
These are the providers:
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.Providers.Add<BrotliCompressionProvider>();
options.Providers.Add<GzipCompressionProvider>();
options.Providers.Add<CustomCompressionProvider>();
options.MimeTypes =
ResponseCompressionDefaults.MimeTypes.Concat(
new[] { "image/svg+xml" });
});
}
HttpContext accessibility improvements
HttpContext accessibility is only valid as long as there is an active HTTP request in ASP.NET Core. Here are some suggestions for accessing HttpContext from Microsoft’s documentation:
Client-side improvements
Client-side optimization is one important aspect of improving performance. When creating a website using ASP.Net Core, consider the following tips:
Bundling
Bundling combines multiple files into a single file, reducing the number of server requests. You can use multiple individual bundles in a webpage.
Minification
Minification removes unnecessary characters from code without changing any functionality, also reducing file size. After applying minification, variable names are shortened to one character and comments and unnecessary whitespace are removed.
Loading JavaScript at last
Load JavaScript files at the end. If you do that, static content will show faster, so users won’t have to wait to see the content.
Use a content delivery network
Use a content delivery network (CDN) to load static files such as images, JS, CSS, etc. This keeps your data close to your consumers, serving it from the nearest local server.
Conclusion
Now you know 10 tips to help improve the performance of ASP.NET Core 3.0 applications. I hope you can implement most of them in your development.
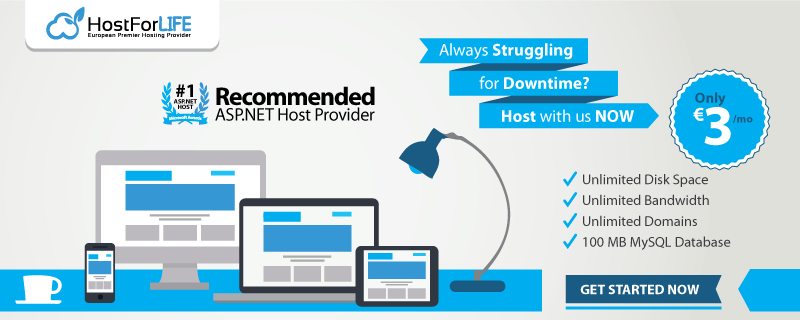