For this blog, my agenda is to provide a step-by-step solution to read and write CSV files in ASP.NET Core 3.0, and CSVHelper. The Same Logic will work for Web Application, Windows Application, and Console Application. In this post, I'm going to create a sample Console Application to show you the process.
Steps:
- Create a Console Application project
- Create a student class inside the project
- Install the CSVHelper from NuGet Package Manager
- Add Mappers folder and inside add a mapper Class for Student
- Add Services Folder in the project and Add StudentService Class inside it
- Write the Logic inside the main method of Program file as its starting point of the application
Create a Console Application project
Give the Name to The Project as "ReadWriteCSVFile". You can give any name.
Create a student class inside the project
Write the below code inside the Student class:
namespace ReadWriteCSVFile {
public class Student {
public int RollNo {
get;
set;
}
public string Name {
get;
set;
}
public string Course {
get;
set;
}
public decimal Fees {
get;
set;
}
public string Mobile {
get;
set;
}
public string City {
get;
set;
}
}
}
In the next step, we are going to install the CSVHelper package so that it will help us to read and write the CSV file in an easy way.
Now Install the CSVHelper from NuGet Package Manager --Version (12.2.1),
- Step 1 - Right Click on the Project
- Step 2 - Go To "Manage NuGet Packages..."
- Step 3 - Go To Browse Tab then move to the Search area
- Step 4 - Type "CSVHelper" in the search box
Here you will see a list of packages. Choose the first one and move it to the right-side panel. You will see one option as Version: (If not installed, if you already installed, then you will see both Installed and Version, two options). Select Version 12.2.1 and click on the install button and follow the steps to install successfully.
Add "Mappers" folder and inside it add mapper Class for Student
- Here you can give any name to the folder and class
- Give the proper name to the class as "StudentMap"
- Make this class as sealed
Write the below code inside the StudentMap Class:
namespace ReadWriteCSVFile.Mappers {
public sealed class StudentMap: ClassMap < Student > {
public StudentMap() {
Map(x => x.RollNo).Name("RollNo");
Map(x => x.Name).Name("Name");
Map(x => x.Course).Name("Course");
Map(x => x.Fees).Name("Fees");
Map(x => x.Mobile).Name("Mobile");
Map(x => x.City).Name("City");
}
}
}
Add "Services" Folder in the project and Add StudentService Class inside it:
- Here, you can give any name to the folder and class
- Give the proper name to the class as "StudentService"
Write the below Code inside the StudentService Class
namespace ReadWriteCSVFile.Services {
public class StudentService {
public List < Student > ReadCSVFile(string location) {
try {
using(var reader = new StreamReader(location, Encoding.Default))
using(var csv = new CsvReader(reader)) {
csv.Configuration.RegisterClassMap < StudentMap > ();
var records = csv.GetRecords < Student > ().ToList();
return records;
}
} catch (Exception e) {
throw new Exception(e.Message);
}
}
public void WriteCSVFile(string path, List < Student > student) {
using(StreamWriter sw = new StreamWriter(path, false, new UTF8Encoding(true)))
using(CsvWriter cw = new CsvWriter(sw)) {
cw.WriteHeader < Student > ();
cw.NextRecord();
foreach(Student stu in student) {
cw.WriteRecord < Student > (stu);
cw.NextRecord();
}
}
}
}
}
Write the Logic inside the main method of Program file as its starting point of the application.
Change the code inside the Main method of Program class as shown below:
namespace ReadWriteCSVFile {
class Program {
static void Main(string[] args) {
Console.WriteLine("Start CSV File Reading...");
var _studentService = new StudentService();
var path = @ "D:\Tutorials\Student.csv";
//Here We are calling function to read CSV file
var resultData = _studentService.ReadCSVFile(path);
//Create an object of the Student class
Student student = new Student();
student.RollNo = 5;
student.Name = "Lucy";
student.Course = "B.Tech";
student.Fees = 75000;
student.Mobile = "7788990099";
student.City = "Pune";
resultData.Add(student);
//Here We are calling function to write file
_studentService.WriteCSVFile(@ "D:\Tutorials\NewStudentFile.csv", resultData);
//Here D: Drive and Tutorials is the Folder name, and CSV File name will be "NewStudentFile.csv"
Console.WriteLine("New File Created Successfully.");
}
}
}
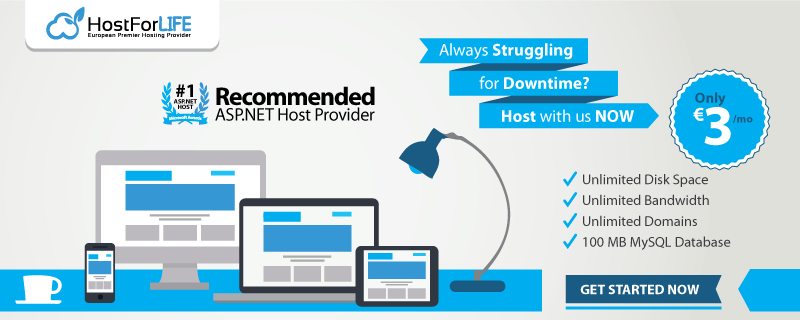