Sometimes as an ASP.NET developer, you may want to create your web application using a specific html structure or specific html elements. ASP.NET Web Controls only allow so much customization over the interface and the actual markup that gets displayed in the browser. Well, I will explain to you how to use hand coded html elements known in the framework as HTML Controls. These HTML Controls allow us to have full control over our interface markup yet still allows us to use our own HTML element as a ASP.NET Server Control. Let’s get started!
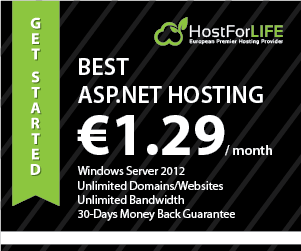
First, add your element to your aspx file. In order to use the element as a Server Control, we need to specify an element ID and the runat="server" properties. Try to add the following code within the <body> tag in Default.aspx:
<h1 id="the_heading" runat="server"></h1>
To demonstrate the abilities of HTML Controls, we’re going to leave this heading tag blank, and populate it via server side code in the code-behind. This single HTML element is enough for a demonstration, but in a larger production application, you will have many elements that need to be dynamically populated throughout your project.
In order to use an HTML Control we’re going to have to import the HTML Controls namespace in Default.aspx.cs like so:
using System.Web.UI.HtmlControls;
Now that we have the required namespace referenced in the top of the code-behind, we can continue by instantiating and manipulating our HTML Control.
Within the standard Page_Load method in Default.aspx.cs, please type the following:
var a = " World!"
the_heading.InnerHtml = "Hello" + a;
In the code above, we are instantiating a variable named a that contains the string " World!". On the next line we reference our HTML Control via it’s ID attribute the_heading.
We call this method as InnerHtml on the HTML element which replaces the content within the elements tags.
Finally, we specify the content to populate the element with as the string "Hello" concatenated with our variable a to create the message "Hello World!". Now when we compile our program, our empty heading tag should contain the friendly message “Hello World!”.
The entire code-behind should look similar to the following:
using System;
using System.Web;
using System.Web.UI;
using System.Web.UI.HtmlControls;
namespace html_controls
{
public partial class _Default : Page
{
protected void Page_Load(object sender, EventArgs e)
{
var a = " World";
the_heading.InnerHtml = "Hello" + a;
}
}
}
That's all about creating an HTML Control from any HTML Elements in ASP.NET. Easy right? Good luck!
HostForLIFE.eu ASP.NET 5 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
