
February 24, 2016 19:43 by
Peter
This code for how to Make all Child Check Boxes checked on Master Check Box Checked Event. Checkboxes allow the user to select one or more options from a set. Typically, you should present each checkbox option in a vertical list. Now write the following code:
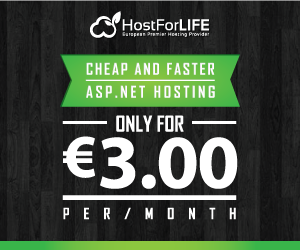
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div style="height:70%;width:70%">
<asp:GridView ID="gridview1" runat="server" AutoGenerateColumns="False"
DataKeyNames="ID" DataSourceID="SqlDataSource1" BackColor="White"
BorderColor="#E7E7FF" BorderStyle="None" BorderWidth="1px" CellPadding="3"
GridLines="Horizontal" Width="100%">
<AlternatingRowStyle BackColor="#F7F7F7" />
<Columns>
<asp:TemplateField>
<HeaderTemplate>
<asp:CheckBox ID="CheckBox2" onclick="headercheckbox(this);" runat="server" />
</HeaderTemplate>
<ItemTemplate>
<asp:CheckBox ID="CheckBox1" onclick="childcheckboxes(this);" runat="server" />
</ItemTemplate>
</asp:TemplateField>
<asp:TemplateField>
<HeaderTemplate>
<asp:LinkButton ID="LinkButton1" runat="server">Delete</asp:LinkButton>
</HeaderTemplate>
<ItemTemplate>
<asp:LinkButton ID="LinkButton2" runat="server">Delete</asp:LinkButton>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="ID" HeaderText="ID" InsertVisible="False"
ReadOnly="True" SortExpression="ID" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
<asp:BoundField DataField="Class" HeaderText="Class" SortExpression="Class" />
<asp:BoundField DataField="Lastname" HeaderText="Lastname"
SortExpression="Lastname" />
</Columns>
<FooterStyle BackColor="#B5C7DE" ForeColor="#4A3C8C" />
<HeaderStyle BackColor="#4A3C8C" Font-Bold="True" ForeColor="#F7F7F7" />
<PagerStyle BackColor="#E7E7FF" ForeColor="#4A3C8C" HorizontalAlign="Right" />
<RowStyle BackColor="#E7E7FF" ForeColor="#4A3C8C" />
<SelectedRowStyle BackColor="#738A9C" Font-Bold="True" ForeColor="#F7F7F7" />
<SortedAscendingCellStyle BackColor="#F4F4FD" />
<SortedAscendingHeaderStyle BackColor="#5A4C9D" />
<SortedDescendingCellStyle BackColor="#D8D8F0" />
<SortedDescendingHeaderStyle BackColor="#3E3277" />
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:akConnectionString %>"
SelectCommand="SELECT [ID], [Name], [Class], [Lastname] FROM [nametb]">
</asp:SqlDataSource>
<script type="text/javascript" language="javascript">
var gridview = document.getElementById("gridview1");
function headercheckbox(checkbox) {
for (var i = 1; i < gridview.rows.length; i++)
{
gridview.rows[i].cells[0].getElementsByTagName("INPUT")[0].checked = checkbox.checked;
}
}
function childcheckboxes(checkbox) {
var atleastekcheck = false;
for (var i = 1; i < gridview.rows.length; i++)
{
if (gridview.rows[i].cells[0].getElementsByTagName("INPUT")[0].checked == false)
{
atleastekcheck = true;
break;
}
}
gridview.rows[0].cells[0].getElementsByTagName("INPUT")[0].checked = !atleastekcheck ;
}
</script>
HostForLIFE.eu ASP.NET Core 1.0 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
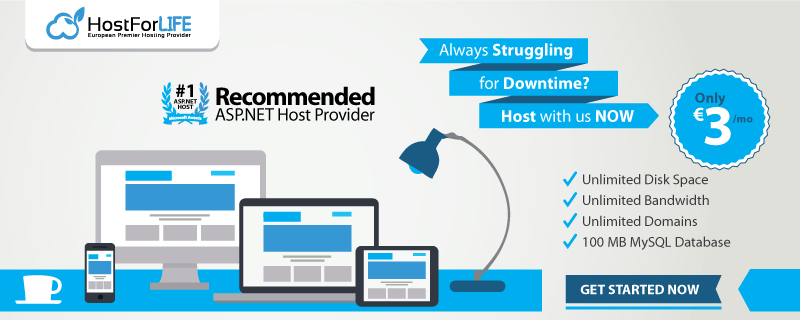

February 17, 2016 21:20 by
Peter
Here's a really short and easy little bit of code that has the potential to be slightly of a time-saver. The FindControl method of the control class is used to find a specific child control of a given parent, searching by ID. This method, however, does not search the control hierarchy recursively: it searches the direct children of the specified parent control only. While writing a recursive version of this method is trivial, a rather nice way to make the technique reusable is to implement it as an extension method. Here's how it can be done:
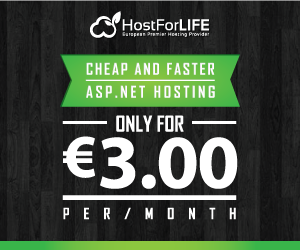
//search the children
foreach (Control child in control.Controls)
{
ctrl = FindControlRecursive(child, id);
if (ctrl != null) break;
}
}
return ctrl;
}
}
}
And to call it:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using Boo.Web.UI.Extensions;
namespace MyWebApp
{
public partial class WebForm1 : System.Web.UI.Page
{
public void Page_Load(object sender, EventArgs e)
{
//call the recursive FindControl method
Control ctrl = this.FindControlRecursive("my_control_id");
}
}
}
Now, don't forget to import the namespace within which the extensions method class is declared- or a compilation error will not long follow. Now, all you need to do is import this same namespace for any pages/user controls/custom controls which need to use the recursive control search and you're good to go.
Doing it this way is of course logically no different to creating a static method in a util class, and passing both the parent control and the ID of the child control you want to find to it, but considering how commonly this functionality is required, it's nice to be able to tack it onto the control class itself, and extension methods provide an elegant way to accomplish this.
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
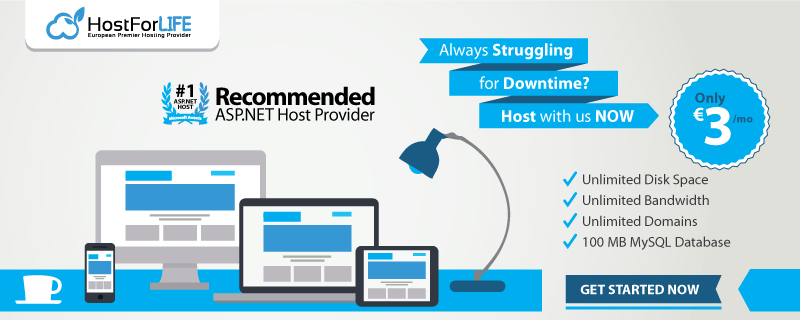

February 3, 2016 19:24 by
Peter
First we craete a table UserImage using database test:
use test
create table UserImage(SrNo int primary key,Name nvarchar(50),UserImg nvarchar(max))
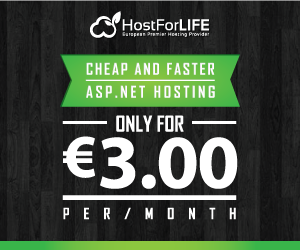
After than we create a Web from like this and take a Folder Image in our WebSite for saving image which are upload by user.
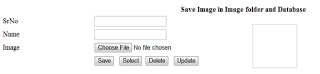
Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<style type="text/css">
.style1 {
width: 100%;
}
.style2
{
width: 351px;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<table class="style1">
<tr>
<td colspan="3"><center><asp:Label ID="Label1" runat="server" Text="Save Image in Image folder and Database" Font-Bold="true"></asp:Label></center>
</td>
</tr>
<tr>
<td>
<asp:Label ID="SrNo" runat="server" Text="SrNo"></asp:Label>
</td>
<td class="style2">
<asp:TextBox ID="txtsrno" runat="server"></asp:TextBox>
</td>
<td rowspan="5">
<asp:Image ID="Image1" runat="server" Height="91px" Width="99px" />
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label4" runat="server" Text="Name"></asp:Label>
</td>
<td class="style2">
<asp:TextBox ID="txtname" runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Label ID="Label5" runat="server" Text="Image"></asp:Label>
</td>
<td class="style2">
<asp:FileUpload ID="FileUpload1" runat="server" />
</td>
</tr>
<tr>
<td>
</td>
<td class="style2">
<asp:Button ID="BSave" runat="server" onclick="BSave_Click" Text="Save" />
<asp:Button ID="BSelect" runat="server" onclick="BSelect_Click" Text="Select" />
<asp:Button ID="BDelete" runat="server" onclick="BDelete_Click" Text="Delete" />
<asp:Button ID="BUpdate" runat="server" onclick="BUpdate_Click" Text="Update" />
</td>
</tr>
<tr>
<td>
</td>
<td class="style2">
<asp:Label ID="Message" runat="server" Text="Label" Visible="False"></asp:Label>
</td>
</tr>
</table>
</div>
</form>
</body>
</html>
Now we create Connection global for Website in web.config
<configuration>
<connectionStrings>
<add name="kush" connectionString="Data Source=KUSH-PC; Initial Catalog=test;Integrated Security=True" providerName="System.Data.SqlClient"/>
</connectionStrings>
<system.web>
<compilation debug="true" targetFramework="4.0"/>
</system.web>
</configuration>
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data.SqlClient;
using System.Configuration;
//Using this namespace for DirectoryInfo and FileInfo
using System.IO;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
con = new SqlConnection(ConfigurationManager.ConnectionStrings["kush"].ConnectionString);
}
SqlConnection con;
SqlCommand cmd;
SqlDataReader dr;
//Saving image in Database and Image Folder which are exist in Website
protected void BSave_Click(object sender, EventArgs e)
{
con.Open();
cmd = new SqlCommand("insert into UserImage (SrNo,Name,UserImg) values (@SrNo,@Name,@UserImg)", con);
cmd.Parameters.AddWithValue("@SrNo", Convert.ToInt32(txtsrno.Text));
cmd.Parameters.AddWithValue("@Name", txtname.Text);
cmd.Parameters.AddWithValue("@UserImg", FileUpload1.FileName);
FileUpload1.SaveAs(Server.MapPath("~/Image/") + FileUpload1.FileName);
cmd.ExecuteNonQuery();
Message.Visible=true;
Message.Text = "Add Successfully ";
con.Close();
txtname.Text = "";
}
//retrive image from Database and Image Folder which are exist in Website
protected void BSelect_Click(object sender, EventArgs e)
{
con.Open();
cmd = new SqlCommand("select * from UserImage where SrNo=@SrNo", con);
cmd.Parameters.AddWithValue("@SrNo", Convert.ToInt32(txtsrno.Text));
dr=cmd.ExecuteReader();
if (dr.Read())
{
txtname.Text = dr["Name"].ToString();
Image1.ImageUrl = "~/Image/" + dr["UserImg"].ToString();
}
con.Close();
}
//The following code is for delete image from Database and Image Folder which are exist in Website
protected void BDelete_Click(object sender, EventArgs e)
{
DirectoryInfo dd = new DirectoryInfo(Server.MapPath("~/Image"));
foreach (FileInfo ff in dd.GetFiles())
{
if (ff.Name == Path.GetFileName(Image1.ImageUrl))
ff.Delete();
}
con.Open();
cmd = new SqlCommand("delete from UserImage where SrNo=@SrNo", con);
cmd.Parameters.AddWithValue("@SrNo", Convert.ToInt32(txtsrno.Text));
cmd.ExecuteNonQuery();
con.Close();
txtname.Text = "";
Message.Visible=true;
Message.Text="Delete Successfully Image "+"For SrNo"+"-"+txtsrno.Text+"";
}
//this code for Update image in Database and Image Folder and old image deleted from Image Folder
protected void BUpdate_Click(object sender, EventArgs e)
{
try
{
DirectoryInfo dd = new DirectoryInfo(Server.MapPath("~/Image"));
foreach (FileInfo ff in dd.GetFiles())
{
if (ff.Name == Path.GetFileName(Image1.ImageUrl))
ff.Delete();
}
con.Open();
cmd = new SqlCommand("update UserImage set Name=@Name, UserImg=@UserImg where SrNo=@SrNo", con);
cmd.Parameters.AddWithValue("@SrNo", Convert.ToInt32(txtsrno.Text));
cmd.Parameters.AddWithValue("@Name", txtname.Text);
if (FileUpload1.HasFile)
{
cmd.Parameters.AddWithValue("@UserImg", FileUpload1.FileName);
}
else
{
cmd.Parameters.AddWithValue("@UserImg", Image1.ImageUrl);
}
FileUpload1.SaveAs(Server.MapPath("~/Image/") + FileUpload1.FileName);
cmd.ExecuteNonQuery();
con.Close();
txtname.Text = "";
Message.Visible = true;
Message.Text = "update Successfully Image " + "For SrNo" + "-" + txtsrno.Text + "";
}
catch (Exception ex)
{
Message.Visible = true;
Message.Text = ex.Message;
}
}
}
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
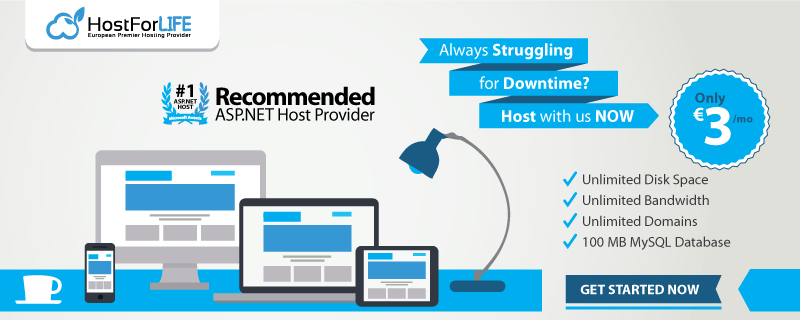