.NET client libraries that integrate with third-party APIs occasionally need to compromise on how enum values are represented in model classes. For example, an API that requires values to be expressed in all uppercase letters force the creation of an enum similar to:
public enum YesNoMaybeEnum
{
YES,
NO,
MAYBE
}
While this will compile, it violates .NET naming conventions. In other cases, the third party may include names that include invalid characters like dashes or periods. For example, Amazon's Alexa messages include a list of potential request types that include a period in the names. These values cannot be expressed as enumation names. While this could be addressed by changing the data type of the serialized property from an enumeration to a string, the property values are no longer constrained and any suggestions from Intellisense are lost.
This article demonstrates how to eat your cake and have it, too. Using attributes and reflection, values can be serialized to and deserialized from JSON.
Serialization with EnumDescription
Let's say we need to serialize values that include periods. Creating an enum like the following generates compile time errors,
public enum RequestTypeEnum
{
LaunchRequest,
IntentRequest,
SessionEndedRequest,
CanFulfillIntentRequest,
AlexaSkillEvent.SkillPermissionAccepted,
AlexaSkillEvent.SkillAccountLinked,
AlexaSkillEvent.SkillEnabled,
AlexaSkillEvent.SkillDisabled,
AlexaSkillEvent.SkillPermissionChanged,
Messaging.MessageReceived
}
The EnumMember attribute defines the value to serialize when dealing with data contracts. Samples on Stack Overflow that show enumeration serialization tend to use the Description attribute. Either attribute can be used or you can create your own. The EnumMember attribute is more tightly bound to data contract serialization while the Description attribute is for use with design time and runtime environments, the serialization approach in this article opts for the EnumMember. After applying the EnumMember and Data Contract attributes, the enum now looks like,
[DataContract(Name = "RequestType")]
public enum RequestTypeEnum
{
[EnumMember(Value = "LaunchRequest")]
LaunchRequest,
[EnumMember(Value = "IntentRequest")]
IntentRequest,
[EnumMember(Value = "SessionEndedRequest")]
SessionEndedRequest,
[EnumMember(Value = "CanFulfillIntentRequest")]
CanFulfillIntentRequest,
[EnumMember(Value = "AlexaSkillEvent.SkillPermissionAccepted")]
SkillPermissionAccepted,
[EnumMember(Value = "AlexaSkillEvent.SkillAccountLinked")]
SkillAccountLinked,
[EnumMember(Value = "AlexaSkillEvent.SkillEnabled")]
SkillEnabled,
[EnumMember(Value = "AlexaSkillEvent.SkillDisabled")]
SkillDisabled,
[EnumMember(Value = "AlexaSkillEvent.SkillPermissionChanged")]
SkillPermissionChanged,
[EnumMember(Value = "Messaging.MessageReceived")]
MessageReceived
}
The EnumMember attribute is also applied to enum members without periods. Otherwise, the DataContractSerilizer would serializes the numeric representation of the enumeration value. Now we can define a DataContract with,
[DataContract]
public class SamplePoco
{
[DataMember]
public RequestTypeEnum RequestType { get; set; }
}
And serialize it to XML with,
SamplePoco enumPoco = new SamplePoco();
enumPoco.RequestType = RequestTypeEnum.SkillDisabled;
DataContractSerializer serializer = new DataContractSerializer(typeof(SamplePoco));
var output = new StringBuilder();
using (var xmlWriter = XmlWriter.Create(output))
{
serializer.WriteObject(xmlWriter, enumPoco);
xmlWriter.Close();
}
string xmlOut = output.ToString();
This generates the following XML,
<?xml version="1.0" encoding="utf-16"?><SamplePoco xmlns:i="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://schemas.datacontract.org/2004/07/EnumSerializationSample"><RequestType>AlexaSkillEvent.SkillDisabled</RequestType>
</SamplePoco>
DataContract serialization is sorted out, but doesn't yet address JSON serialization.
JSON Serialization
If you need to work with any REST API endpoints, then you'll need to support JSON. The NewtonSoft JSON has its own serialization strategy, and so the EnumMember attribute needs to be leveraged to integrate with it using a custom JsonConverter, but before taking that step, the enumation value must be read from the attribute.
This method accepts an enum value and returns the string value in the EnumMember attribute.
private string GetDescriptionFromEnumValue(Enum value)
{
#if NETSTANDARD2_0
EnumMemberAttribute attribute = value.GetType()
.GetField(value.ToString())
.GetCustomAttributes(typeof(EnumMemberAttribute), false)
.SingleOrDefault() as EnumMemberAttribute;
return attribute == null ? value.ToString() : attribute.Value;
#endif
#if NETSTANDARD1_6 || NETSTANDARD1_3 || NET45 || NET47
EnumMemberAttribute attribute = value.GetType()
.GetRuntimeField(value.ToString())
.GetCustomAttributes(typeof(EnumMemberAttribute), false)
.SingleOrDefault() as EnumMemberAttribute;
return attribute == null ? value.ToString() : attribute.Value;
#endif
throw new NotImplementedException("Unsupported version of .NET in use");
}
There's a subtle difference between the .NET Standard 2.0 implementation and the others. In .NET Standard 1.6 and prior versions, use the GetRuntimeField method to get a property from a type. In .NET Standard 2.0, use the GetField method to return the property of a type. The compile-time constants and checks in the GetDescriptionFromEnumValue abstract away that complexity.
Coming the other way, a method needs to take a string and convert it to the associated enumeration.
public T GetEnumValue(string enumMemberText)
{
T retVal = default(T);
if (Enum.TryParse<T>(enumMemberText, out retVal))
return retVal;
var enumVals = Enum.GetValues(typeof(T)).Cast<T>();
Dictionary<string, T> enumMemberNameMappings = new Dictionary<string, T>();
foreach (T enumVal in enumVals)
{
string enumMember = enumVal.GetDescriptionFromEnumValue();
enumMemberNameMappings.Add(enumMember, enumVal);
}
if (enumMemberNameMappings.ContainsKey(enumMemberText))
{
retVal = enumMemberNameMappings[enumMemberText];
}
else
throw new SerializationException($"Could not resolve value {enumMemberText} in enum {typeof(T).FullName}");
return retVal;
}
The values expressed in the EnumMember attributes are loaded into a dictionary. The value of the attribute serves as the key and the associated enum is the value. The dictionary keys are compared to the string value passed to the parameter and if a matching EnumMember value is found, then the related enum is returned and so "AlexaSkillEvent.Enabled" returns RequestTypeEnum.SkillEnabled.
Using this method in a JSON Converter, the WriteJson method looks like the following,
public class JsonEnumConverter<T> : JsonConverter where T : struct, Enum, IComparable, IConvertible, IFormattable
{
public override void WriteJson(JsonWriter writer, object value, JsonSerializer serializer)
{
if (value != null)
{
Enum sourceEnum = value as Enum;
if (sourceEnum != null)
{
string enumText = GetDescriptionFromEnumValue(sourceEnum);
writer.WriteValue(enumText);
}
}
}
Please note that an enum constraint is applied to the generic type class declaration. This wasn't possible until C# version 7.3. If you cannot upgrade to use C# version 7.3, just remove this constraint.
The corresponding ReadJson method is,
public override object ReadJson(JsonReader reader, Type objectType, object existingValue, JsonSerializer serializer)
{
object val = reader.Value;
if (val != null)
{
var enumString = (string)reader.Value;
return GetEnumValue(enumString);
}
return null;
}
Now the class definition needs to apply the JsonConverter class to the RequestType property,
[DataContract]
public class SamplePoco
{
[DataMember]
[JsonConverter(typeof(JsonEnumConverter<RequestTypeEnum>))]
public RequestTypeEnum RequestType { get; set; }
}
Finally, the SamplePoco class is serialized to JSON,
SamplePoco enumPoco = new SamplePoco();
enumPoco.RequestType = RequestTypeEnum.SkillEnabled;
string samplePocoText = JsonConvert.SerializeObject(enumPoco);
This generates the following JSON,
{
"RequestType":"AlexaSkillEvent.SkillEnabled"
}
And deserialing the JSON yields the RequestTypeEnum.SkillEnabled value on the sample class.
string jsonText = "{\"RequestType\":\"AlexaSkillEvent.SkillEnabled\"}";
SamplePoco sample = JsonConvert.DeserializeObject<SamplePoco>(jsonText);
HostForLIFE.eu ASP.NET Core 2.2.1 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
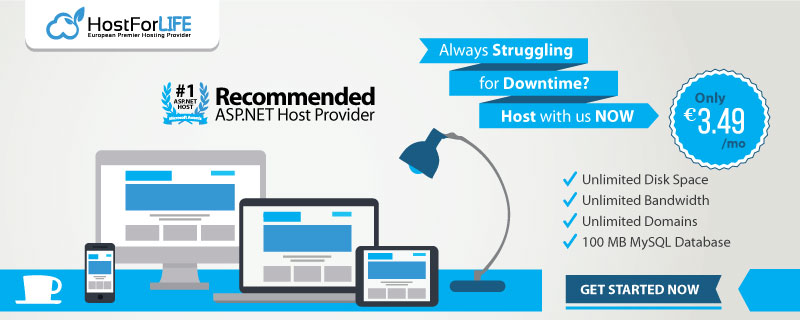