A CAPTCHA (an acronym for "Completely Automated Public Turing test to tell Computers and Humans Apart") is a type of challenge-response test used in computing to determine whether or not the user is human. The term was coined in 2003 by Luis von Ahn, Manuel Blum, Nicholas J. Hopper, and John Langford.
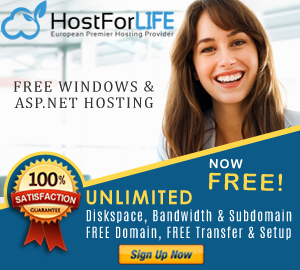
The technology is used mostly to block spammers and bots that try to automatically harvest email addresses or try to automatically sign up for or make use of Web sites, blogs or forums. CAPTCHA, whose users include Yahoo and Google, blocks automated systems, which can't read the distorted letters in the graphic.
The algorithm for CAPTCHA is public, as the "P" in the name implies. The test was developed in various forms around 1996, but it got its distinctive name in 2000 from researchers at Carnegie Mellon University and IBM. Cracking the algorithm won't make the CAPTCHA vulnerable, since the algorithm is only used for generating the random series of letters and numbers in the image. The system works because humans and computers process strings of characters differently.
One of the problems with CAPTCHA is that sometimes the characters are so distorted that they can't even be recognized by people with good vision, let alone visually handicapped individuals. Depending on local regulations for handicapped access to Web sites, this can also be a compliance issue for some Web-based businesses.
CAPTCHA technology is easy to implement, but requires some knowledge of hypertext preprocessor (PHP) or other Web scripting languages. For more information and links to extensive resources, check the How to use CAPTCHA Web site and The CAPTCHA Project. Both sites also have examples of CAPTCHAs and in-depth tutorials on how to develop and implement CAPTCHA for your Web site.
So, in this article, I will explain how to make basic CAPTCHA.
You can set the height and width of the CAPTCHA image while initializing the CAPTCHA class. The CAPTCHA image generated from the class can be set to the image control directly or it can be save to the local path and then set to the image control. For this example I am saving it to the local path and then set to the image control. And the CAPTCHA image code generated by the class is automatically set to the session variable named CaptchaCode.
Time to get some hand on it
On the page get an image control to display CAPTCHA image, a textbox where user will enter the CAPTCHA text and a button when clicked, we can check and validate if the code entered is correct or not. Plug the DLL in your ASP.NET project and go to the Page_Load event. Here we will generate the image of the height and width we wish to have for out CAPTCHA image and then save the image to the CaptchaImages folder with the random image code generated. The code on the Page_Load event goes like this:
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
CaptchaImage cImage = new CaptchaImage(CaptchaImage.generateRandomCode(), 140, 40);
cImage.Image.Save(Server.MapPath("~\\CaptchaImages\\" + Convert.ToString(Session["CaptchaCode"]) + ".jpg"), ImageFormat.Jpeg);
CaptachaImage.ImageUrl = "~\\CaptchaImages\\" + Convert.ToString(Session["CaptchaCode"]) + ".jpg";
cImage.Dispose();
}
CaptchaCode = Convert.ToString(Session["CaptchaCode"]);
}
Before you can use the above code, declare a public variable which I have used to hold the value of the CAPTCHA code. I named it CaptchaCode. You can name it whatever you like it. We will be using this variable later to check it against the user input. Hit F5 to start and test your application. If everything is in place and you will be able to see the below output.

To check if the user enters the correct CAPTCHA code, we must have an event on button click which will validate the user input and prompt the user if CAPTCHA code is correct or incorrect. The code on the button click is:
protected void btn_Validate(object sender, EventArgs e)
{
if (CaptchaCode == txt_ccode.Text)
{
ClientScript.RegisterClientScriptBlock(typeof(Page), "ValidateMsg", "<script>alert('You entered Correct CAPTCHA Code!');</script>");
}
else
{
ClientScript.RegisterClientScriptBlock(typeof(Page), "ValidateMsg", "<script>alert('You entered INCORRECT CAPTCHA Code!');</script>");
}
}
The above code will check the CAPTCHA code entered by the user and check against the CAPTCHA code we previously saved in the session variable (CaptchaCode). If the validation is a success, user will get the message notifying him that he enters correct CAPTCHA code or the error message otherwise.
And that's it, we have successfully implement a CAPTCHA in our ASP.NET application. So, here are few things we can do with this CAPTCHA library:
Set image height and width.
Saves the image to the local path.
Automatically sets the generated CAPTCHA code to the application session.
HostForLIFE.eu ASP.NET 4.5 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
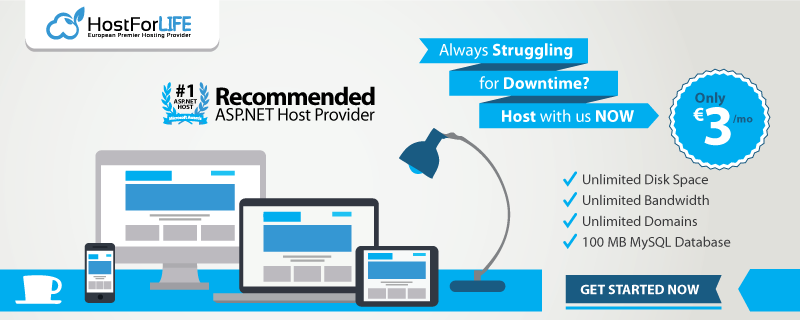