Most of the newbies and few administrators handling the deployment of their company’s ASP.Net applications on the Windows Server must be knowing about the basics of how ASP.Net is associated with the IIS web server. Here’s a quick tip for you to quickly check whether the exact version of .NET framework has been installed on the Server and also to check whether it has been registered with the IIS or not.
In this scenario, I'm going to consider a fresh installation of the Windows Server (2008 R2/ 2012 R2). SO make sure you have the below mentioned configuration done accordingly.
How to Find The Existence of the .NET Framework and Its Files
Navigate to this location, to make sure the .Net Framework that you are wanting/looking for is installed.
File location: (32-bit): C:\Windows\Microsoft.NET\Framework\ (By default)
For 64-bit: C:\Windows\Microsoft.NET\Framework64\ (By default)
There are several .NET Framework versions available. Some are included in some Windows OS by default and all are available to download at Microsoft website as well.
Following is a list of all released versions of .NET Framework:
- .NET Framework 1.0 (Obsolete)
- .NET Framework 1.1 (Obsolete, comes installed in Windows Server 2003)
- .NET Framework 2.0* (Obsolete, comes as a standalone installer)
- .NET Framework 3.0* (comes pre-installed in Windows Vista and Server 2008)
- .NET Framework 3.5* (comes pre-installed in Windows 7 and Server 2008 R2)
- .NET Framework 4.0*
- .NET Framework 4.5* (comes pre-installed in Windows 8/8.1 and Server 2012/2012 R2)
Note: The framework marked with (*) have their later versions which is available as service packs, that can be downloaded from Microsoft’s Download website.
To check the ASP.Net application version compatibility, refer this MSDN article. Which is also shown below
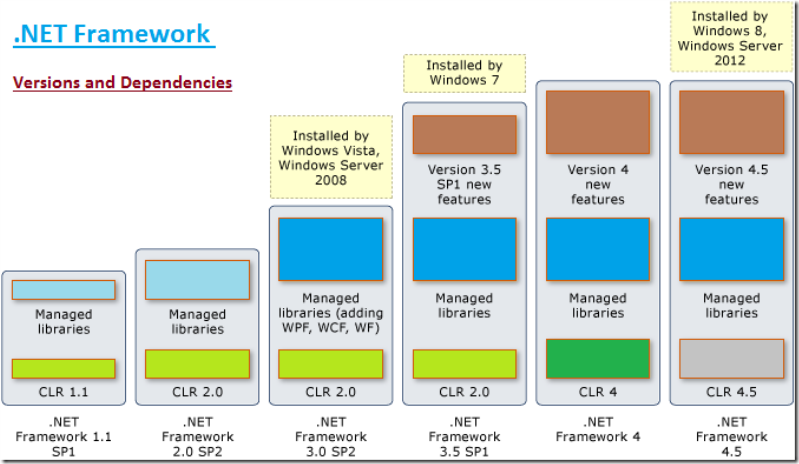
If you find the respective folder, say v4.0.xxx, then it means that the Framework 4.0 or above has been installed. X:\Windows\Microsoft.NET\Framework\v4.0.30319 (The X:\ is replaced by the OS drive)
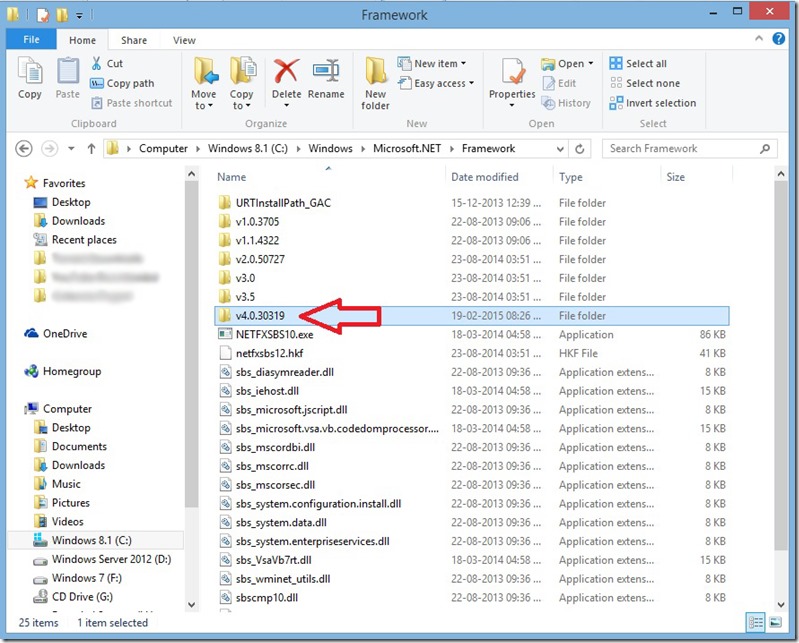
Also you will find a similar folder for the x64 bit .Net Framework and its files associated in this location X:\Windows\Microsoft.NET\Framework64\v4.0.30319 (The X:\ is replaced by the OS drive)
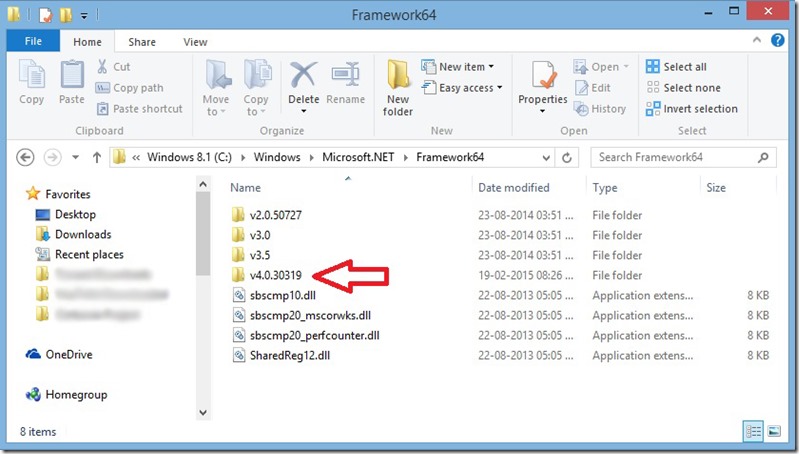
This can also be confirmed using another method by navigating into the Windows Registry to find a key and its existence confirms the same.
How to Find the .NET Framework Versions by Viewing the Registry
1. On the Start menu, choose Run.
2. In the Open box, enter regedit.exe.
You must have administrative credentials to run regedit.exe.
In the Registry Editor, open the following subkey:
HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\NET Framework Setup\NDP\v4\Full
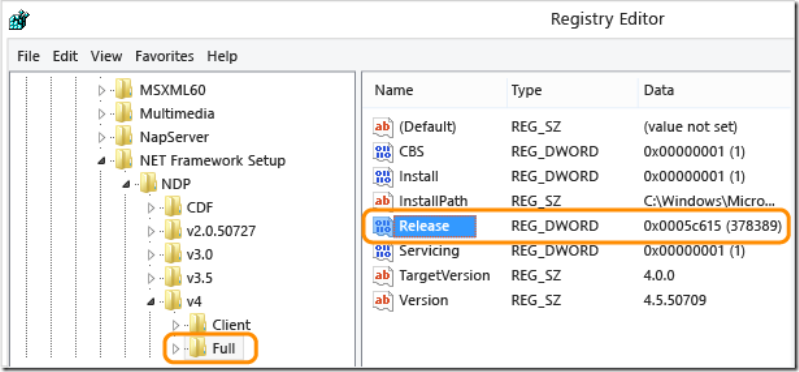
This confirms the existence of .Net framework 4 and above(4.5 / 4.5.1 / 4.5.2 Only).
In most of the cases people might ignore this, assuming they have already configured ASP.Net by choosing from the server roles or any other version of .Net application that was working earlier.
This step will guide you to check whether your new application (with respect to the .Net Framework compatibility) has its asp.net component registered or not.
Simply Check ASP.NET 4.5 has been Installed via IIS
To check that .net 4.5 has been installed on the server, please just simply create a website in IIS and hit the “Select” button to check the .Net framework versions available to create Application Pool.
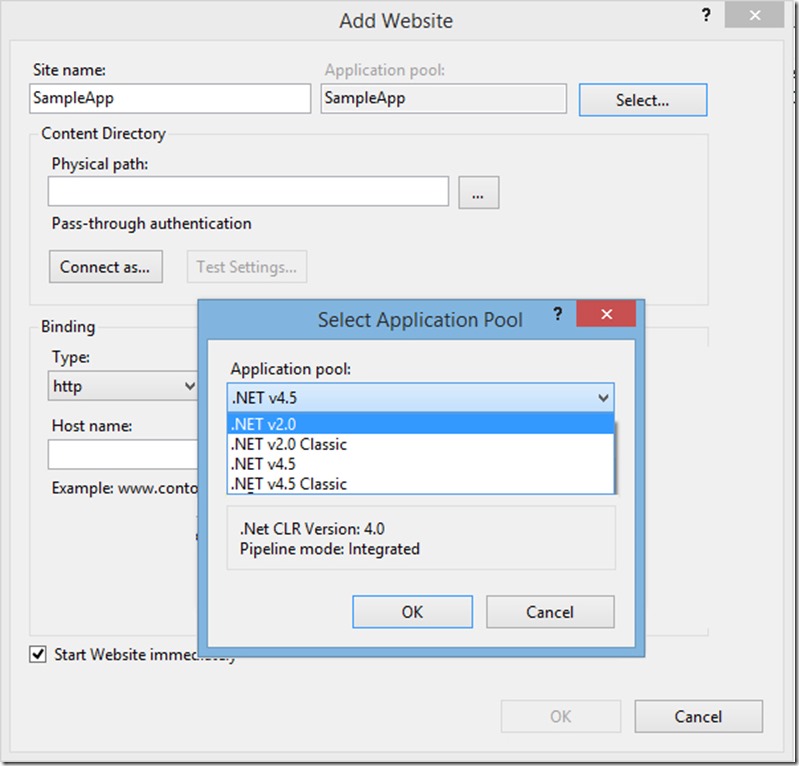
If you find v4.5 instead of v4.0, then it clearly justifies that .Net framework version 4.5 or above (4.5 / 4.5.1 / 4.5.2 only) has been installed and made available to run any website that requires this framework’s version.
If you don't find it, then navigate to the framework’s root folder (refer pic 2) and run “aspnet_regiis.exe” which will in turn register the asp.net component to the IIS.
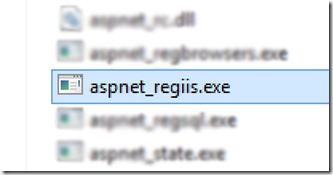
Once you have registered the ASP.Net component, restart the IIS from the command prompt by typing “iisreset”, then launch your IIS Manager and follow the Step 3, to check the version listed in the available frameworks in the “Add Website” windows as shown above.
Now you are all set to go and start deploying your web application through Web Deploy / FTP or any other.