I'll go over three widely used approaches to handle JSON in.NET in this post. JavaScript Object Notation, or JSON, is a text-based, lightweight format that is frequently used for data interchange. There are various ways to work with JSON in.NET, each with advantages of its own and appropriate for particular situations. You can select from a variety of libraries, each designed to meet specific needs. Here are three well-liked choices: NetJSON, System.Text.Json, and Newtonsoft.Json, as well as code samples to get you started.
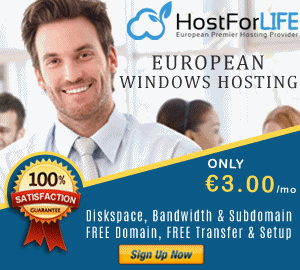
Working With Json in ASP.NET
- Using Newtonsoft.Json (Json.NET)
- Using System.Text.Json (Built-in .NET Core Library)
- Using NetJSON (High-Performance JSON Library)
Let's create an employee class and prepare data to work with different JSON parsing libraries.
public class Employee
{
public int Id { get; set; }
public string? Name { get; set; }
public string? Department { get; set; }
}
Now, prepare the data with a list of employees.
static List<Employee> Getemployees()
{
var employees = new List<Employee>
{
new Employee { Id = 1, Name = "John Doe", Department = "HR" },
new Employee { Id = 2, Name = "Jane Smith", Department = "IT" },
new Employee { Id = 3, Name = "Mike Johnson", Department = "Finance" }
};
return employees;
}
Using Newtonsoft.Json (Json.NET)
How do you install the Newtonsoft.Json package using the Manage NuGet Packages feature?

Install Newtonsoft.Json package
Search for the Newtonsoft.Json package in the NuGet package list as shown below.

Search package and install package
Let's look at an example where we serialize a list of employees into a JSON string and then deserialize the JSON string back into a list of employees.
static void JsonUsingNewtonsoft(List<Employee> employees)
{
// Serialize to JSON
string json = JsonConvert.SerializeObject(employees);
Console.WriteLine("Serialized JSON (Newtonsoft.Json):");
Console.WriteLine(json);
// Deserialize back to Employee object
List<Employee> employeeList = JsonConvert.DeserializeObject<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee:");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
Console.ReadLine();
}

The snippet above shows the output of the code using the Newtonsoft.Json package.
Using System.Text.Json (Built-in .NET Core Library)
System.Text.Json, introduced in .NET Core 3.0, is the default and highly efficient library for processing JSON in .NET. It offers powerful features for serialization, deserialization, and parsing JSON documents.
static void JsonUsingSystemTextJson(List<Employee> employees)
{
// Serialize to JSON using System.Text.Json
string json = JsonSerializer.Serialize(employees);
Console.WriteLine("Serialized JSON (System.Text.Json):");
Console.WriteLine(Environment.NewLine);
Console.WriteLine(json);
// Deserialize back to Employee object using System.Text.Json
List<Employee> employeeList = JsonSerializer.Deserialize<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee (System.Text.Json):");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
}
The snippet above shows the output of the code using the
Using NetJSON (High-Performance JSON Library)
NetJSON is a high-performance, lightweight JSON library for .NET, designed for speed and minimal memory usage. It is ideal for performance-critical scenarios, such as processing large datasets, high-throughput applications, or real-time systems. While it lacks the extensive customization options of libraries like Newtonsoft.Json, it stands out for its simplicity and exceptional performance.

Install NetJSON Package from NuGet Package Manager
Here’s an example demonstrating the use of the NetJSON package to serialize a list of employees into a JSON string and then deserialize the JSON string back into a list of employees.
static void JsonUsingNetJSON(List<Employee> employees)
{
// Serialize to JSON using NetJSON
string json = NetJSON.NetJSON.Serialize(employees);
Console.WriteLine("Serialized JSON (NetJSON):");
Console.WriteLine(Environment.NewLine);
Console.WriteLine(json);
// Deserialize back to Employee object using NetJSON
List<Employee> employeeList = NetJSON.NetJSON.Deserialize<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee (NetJSON):");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
}

The snippet above shows the output of the code using the NetJSON package.
Let's put all methods together in a single class.
using JsonExample;
using Newtonsoft.Json;
using JsonSerializer = System.Text.Json.JsonSerializer;
var employeeList = Getemployees();
// Using Newtonsoft.Json
JsonUsingNewtonsoft(employeeList);
// Using System.Text.Json .Net Core built-in library
JsonUsingSystemTextJson(employeeList);
// Using NetJSON
JsonUsingNetJSON(employeeList);
Console.ReadLine();
static List<Employee> Getemployees()
{
var employees = new List<Employee>
{
new Employee { Id = 1, Name = "John Doe", Department = "HR" },
new Employee { Id = 2, Name = "Jane Smith", Department = "IT" },
new Employee { Id = 3, Name = "Mike Johnson", Department = "Finance" }
};
return employees;
}
static void JsonUsingNewtonsoft(List<Employee> employees)
{
// Serialize to JSON
string json = JsonConvert.SerializeObject(employees);
Console.WriteLine("Serialized JSON (Newtonsoft.Json):");
Console.WriteLine(Environment.NewLine);
Console.WriteLine(json);
// Deserialize back to Employee object
List<Employee> employeeList = JsonConvert.DeserializeObject<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee:");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
}
static void JsonUsingSystemTextJson(List<Employee> employees)
{
// Serialize to JSON using System.Text.Json
string json = JsonSerializer.Serialize(employees);
Console.WriteLine("Serialized JSON (System.Text.Json):");
Console.WriteLine(Environment.NewLine);
Console.WriteLine(json);
// Deserialize back to Employee object using System.Text.Json
List<Employee> employeeList = JsonSerializer.Deserialize<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee (System.Text.Json):");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
}
static void JsonUsingNetJSON(List<Employee> employees)
{
// Serialize to JSON using NetJSON
string json = NetJSON.NetJSON.Serialize(employees);
Console.WriteLine("Serialized JSON (NetJSON):");
Console.WriteLine(Environment.NewLine);
Console.WriteLine(json);
// Deserialize back to Employee object using NetJSON
List<Employee> employeeList = NetJSON.NetJSON.Deserialize<List<Employee>>(json);
Console.WriteLine("\nDeserialized Employee (NetJSON):");
foreach (Employee employee in employeeList)
{
Console.WriteLine($"Id: {employee.Id}, Name: {employee.Name}, Department: {employee.Department}");
}
}

Recommendation. Choosing the Right JSON Library in the Real-time Project.
- System.Text.Json: Best suited for modern .NET projects due to its performance and native integration.
- Newtonsoft.Json: Ideal for projects requiring compatibility or advanced customization features.
- NetJSON: Recommended for performance-critical scenarios such as real-time systems or large-scale data processing.
Note. Each approach serves different needs, and your choice depends on project requirements and performance considerations
Summary
This article covers three popular methods for working with JSON in .NET, highlighting their unique advantages and ideal use cases. JSON is a lightweight, text-based format commonly used for data exchange, and .NET provides versatile tools to handle it effectively. Note. I have provided a working solution compatible with Visual Studio 2022, which you can download from the top left corner of the article. Simply click the download icon to get the .zip file.