Because it enables the development of applications that expand and adapt to the requirements of various services and platforms, web API development is a crucial part of the current development scenario. CRUD actions, which enable the fundamental interface with the application's data, are one of the cornerstone procedures in the development of APIs. Starting with CRUD operations makes perfect sense if you are "green" with.NET Core because it teaches you the basics of creating a Web API and handling data.
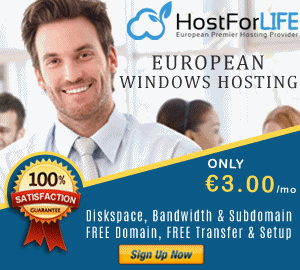
The creation of a.NET Core 8 Web API project and the specification of the endpoints for each CRUD operation will be covered in this tutorial. Effective data handling techniques are outlined by the Entity Framework Core, an ORM system made to communicate with.NET databases. By the end of this article, you will have mastered the fundamentals of RESTful design in.NET Core and developed a functional Web API that can manipulate data.
This tutorial is intended for both a novice front-end developer looking to hone their skills and an aspiring backend developer with experience in.NET Core 8 API development. Therefore, you will undoubtedly learn how to begin creating APIs in.NET Core 8 if you fit into either of the two categories. Let's go over this initial phase.
Open Visual Studio 2022 and Choose "Create a new project".

On the Create a new project page, search for "Web API" on the search bar, select the project template and press the "next" button.

On the configuration of the project, enter the Project Name and choose the check box to keep the solution file and project in the same directory.

To configure the Framework version, tick the boxes according to the screenshot, and click "create" on the project's additional details page. To get things started, it will generate the project using the default files.

Initially, the project folder structure looked like this.

First, we need to install the packages required for the ORM to interact with the Database. To install the packages, Right click on the solution and choose "Manage NuGet Packages... "
On the NuGet page, search for the below two packages and install the versions above 8.
Microsoft.EntityFrameworkCore.Tools
Microsoft.EntityFrameworkCore.SqlServer
Then, Right-click on the solution. Create a new Class file named Employee.cs and paste the code.
namespace EmployeePortal.Models.Entities
{
public class Employee
{
public Guid Id { get; set; }
public required string Name { get; set; }
public required string Email { get; set; }
public required string PhoneNumber { get; set; }
public decimal Salary { get; set; }
}
}
Then, we need to create a DB Context file for the application that holds the configuration for the ORM and its Entities. Create a file named ApplicationDbContext.cs and paste the below code.
using EmployeePortal.Models.Entities;
using Microsoft.EntityFrameworkCore;
namespace EmployeePortal
{
public class ApplicationDbContext : DbContext
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options)
: base(options)
{
}
public DbSet<Employee> Employees { get; set; }
}
}
Then, we need to add a DB connection string to the appsettings.json file.
"ConnectionStrings": {
"DefaultConnection": "Server=your_server_name;Database=your_database_name;User Id=your_username;Password=your_password;TrustServerCertificate=True;"
}
Then, we need to add the SQL Server services to our Program.cs file and add the below code to the program file under the services, which tells the application to use the SQL server from the connection string.
builder.Services.AddDbContext<ApplicationDbContext>(options => options.UseSqlServer(
builder.Configuration.GetConnectionString("DefaultConnection")));
Now, we add a migration to create a snapshot of our entities from the application because we are using the Code First Approach in EF. So, we need to specify the entities and their relationships, and then we run the migration which will create a Database and tables based on the relationship of the entities.
Open the package manager console and run the following commands.
- add-migration "initial one": generates a migration file based on the current state of your data models compared to the database schema.
- update-database: applies the migration to your database, creating or altering tables, columns, or relationships as defined in the migration file.
Now, If you open the SQL server, you can see the database and the tables.

We need to create a Web API controller to fetch the data from the database through the endpoints. Right-click on the controller's folder and choose to add a new Web API Controller.

You can request the endpoints using the necessary information.
Endpoints and their use cases with return types
- Get All Employees: it is GET api/employees. Method: GetEmployees(). Purpose: Fetches all the employees from the store. Response: Returns an Ok (HTTP 200) status with the body, which contains the list of employees.
- Get Employee by ID: it is GET api/employees/{id}. Method: GetEmployeeById(Guid id). Purpose: Use unique IDs to retrieve an employee. Response: Returns an Ok (HTTP 200) status and data about an employee when such is available, however, there is a risk that if no such employees can be traced then one will be returned as NotFound (HTTP 404).
- Add a New Employee: it is POST api/employees. Method: AddEmployee(EmployeeDto employees). Purpose: Whenever an employee Dto record is transmitted to the application, the application updates the defined employees in the store. Response: Returned 201 Created status whenever an employer is successfully added.
- Update Employee: it is PUT api/employees/{id}. Method: UpdateEmployee(Guid id, UpdateEmployeeDto employeeDto). Purpose: One has to search the employee by the unique ID and change his information. Response: If the updates work effectively, they return an Ok (HTTP 200) status, while the NotFound (HTTP 404) will be resolved in situations where no suitable culprit persons are available.
- Delete Employee: it is DELETE api/employees/{id}. Method: DeleteEmployee(Guid id). Purpose: When databases are directed to delete certain profiles, the corresponding unique ID numbers are used. Response: If it works, returns NoContent (HTTP 204) in situations where the deletion was successful, whereas NotFound (HTTP 404) if no matching employee is found.
Conclusion
In this article, we explored a basic implementation of CRUD operations for managing employee data in a .NET Core Web API. By following these steps, we created endpoints to add, retrieve, update, and delete employees, using DTOs to encapsulate and simplify data transfer. This approach helps establish a solid foundation for building RESTful APIs and managing data flow in a secure and organized manner.