
September 4, 2023 08:18 by
Peter
Using tools like Swagger/OpenAPI or NSwag to create thorough API documentation for an ASP.NET Core Web API is a critical step in ensuring that your API is well-documented and easy for other developers to understand and utilize. I'll show you how to build API documentation in an ASP.NET Core Web API project using Swagger/OpenAPI in the steps below.
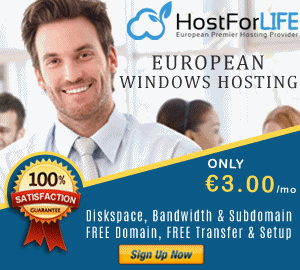
Step 1: Begin by creating an ASP.NET Core Web API Project.
If you do not already have an ASP.NET Core Web API project, you can build one by following the instructions below.
Start Visual Studio or your favorite code editor.
Make a new project and select "ASP.NET Core Web Application."
Choose the "API" template and press "Create."
Step 2: Set up Swashbuckle.AspNetCore
Swashbuckle.AspNetCore is a library that makes integrating Swagger/OpenAPI into your ASP.NET Core Web API project easier. It may be installed using NuGet Package Manager or the.NET CLI.
dotnet add package Swashbuckle.AspNetCore
Step 3. Configure Swagger/OpenAPI
In your Startup.cs file, configure Swagger/OpenAPI in the ConfigureServices and Configure methods.
using Microsoft.OpenApi.Models;
using Swashbuckle.AspNetCore.SwaggerGen;
using Swashbuckle.AspNetCore.SwaggerUI;
Author: Sardar Mudassar Ali Khan
public void ConfigureServices(IServiceCollection services)
{
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo
{
Title = "Auth API",
Version = "v1",
Description = "Description of your API",
});
var xmlFile = $"{Assembly.GetExecutingAssembly().GetName().Name}.xml";
var xmlPath = Path.Combine(AppContext.BaseDirectory, xmlFile);
c.IncludeXmlComments(xmlPath);
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseSwagger();
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "Auth API");
c.RoutePrefix = "api-docs"; // You can change the URL path as needed.
});
}
Step 4. Add XML Comments
For Swagger to provide descriptions and summaries for your API endpoints, you should add XML comments to your controller methods. To enable XML documentation, go to your project's properties and enable the "Generate XML documentation file" option.
Then, add comments to your controller methods like this:
using Microsoft.AspNetCore.Mvc;
using System.Collections.Generic;
using YourNamespace.Models; // Replace with your model namespace
using Microsoft.EntityFrameworkCore;
Author: Peter
[ApiController]
[Route("api/items")]
public class ItemsController : ControllerBase
{
private readonly YourDbContext _context; // Replace with your DbContext type
public ItemsController(YourDbContext context)
{
_context = context;
}
[HttpGet]
public IActionResult GetItems()
{
try
{
var items = _context.Items.ToList(); // Assuming "Items" is your DbSet
if (items == null || items.Count == 0)
{
return NoContent(); // Return 204 No Content if no items are found.
}
return Ok(items); // Return 200 OK with the list of items.
}
catch (Exception ex)
{
// Log the exception or handle it accordingly.
return StatusCode(500, "Internal Server Error"); // Return a 500 Internal Server Error status.
}
}
}
Step 5. Run Your API and Access Swagger UI
Build and run your ASP.NET Core Web API project. You can access the Swagger UI by navigating to /api-docs/index.html (or the path you configured in Startup.cs) in your web browser. You should see the API documentation generated by Swagger/OpenAPI.
Now, your ASP.NET Core Web API has comprehensive API documentation generated using Swagger/OpenAPI. Developers can use this documentation to understand and interact with your API effectively.