As I have previously written, ASP.NET 4.5 has introduced many new features. One of them is model binding in ASP.NET 4.5. Now, I will give implementation how to use model binding with ASP.NET Forms 4.5.
Sample data
Before going on with examples let’s create some test data too. Let’s define class Product as follows.
1.public class Product
2.{
3. public int Id { get; set; }
4. public string Name { get; set; }
5. public decimal Price { get; set; }
6.}
We need more than one product and to avoid creating database or doing other complex things we define simple method that returns us list of products.
01.private IList<Product> GetProductList()
02.{
03. return new[]{
04. new Product { Id=1, Name="Heineken", Price=0.88m },
05. new Product { Id=2, Name="Skopsko", Price=0.97m },
06. new Product { Id=3, Name="Gambrinus", Price=0.63m },
07. new Product { Id=4, Name="Lapin Kulta", Price=0.92m },
08. new Product { Id=5, Name="Rock", Price=1.1m }
09. };
10.}
That’s enough for preparation work. You can use this method also as static method of Product class.
Strongly typed controls
Previous versions of ASP.NET Forms used reflection on data source objects to read values from objects they were bound to. Web is full of examples about how to bind DataTable to GridView or Repeater and so on. With strongly typed controls ASP.NET Forms makes step closer to object orientation in presentation layer.
Let’s define strongly typed Repeater that shows us list of products.
01.<asp:Repeater
02. runat="server" ID="Repeater1"
03. ModelType="WebApplication45.Product"
04. SelectMethod="GetProducts">
05. <HeaderTemplate>
06. <table>
07. <tr>
08. <th>ID</th>
09. <th>Name</th>
10. <th>Price</th>
11. </tr>
12. </HeaderTemplate>
13. <ItemTemplate>
14. <tr>
15. <td><%# Item.Id %></td>
16. <td><%# Item.Name %></td>
17. <td><%# Item.Price %></td>
18. </tr>
19. </ItemTemplate>
20. <FooterTemplate>
21. </table>
22. </FooterTemplate>
23.</asp:Repeater>
Note the following things:
1. There is new ModelType property we can use to specify type of data item.
2. There is new SelectMethod property that specifies the method to call when repeater wants to load data.
3. To show data in different templates we can use Item property that is strongly typed and – of course – IntelliSense is also supported. You can see IntelliSense in action on screenshot on below.
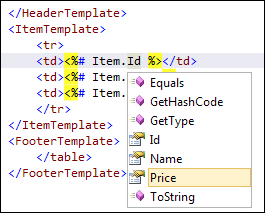
Now let’s see how SelectMethod works.
Select method
Select method is expected to return us result of type IQueryable<ModelType>. If our data source returns something else we can usually use LINQ extension methods to convert our source data to IQueryable. In our case GetProducts() method is defined as follows.
1.public IQueryable<Product> GetProducts()
2.{
3. return GetProductList().AsQueryable();
4.}
SelectMethod allows us to do much more but for this example it is enough.
After running our ASP.NET Forms solution we will see product table like this.
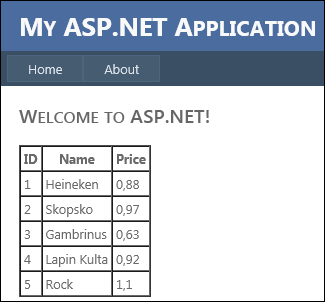
Conclusion
Repeater is not the only strongly typed control available in ASP.NET 4.5 – there are many other familiar controls that have support for strongly typed data. In this posting we saw that defining strongly typed web controls is easy. It is also easy to provide data to these controls using data selecting method. SelectMethod is more powerful than you can see here but this is the topic of some other (hopefully interesting) posting.