Many applications demand the ability to generate PDF documents programmatically. GrapeCity's GcPdf is a comprehensive library in the.NET ecosystem that allows developers to easily generate, change, and manipulate PDF documents. This blog post will show you how to utilize GcPdf to generate PDF documents programmatically in.NET C#, with actual examples to back it up.
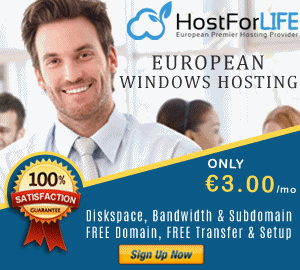
What exactly is GcPdf?
GcPdf is a.NET package that offers extensive PDF document production and manipulation features. It has a variety of features, including:
- Creating PDF documents from the ground up.
- Text, photos, and shapes can be added to PDF pages.
- Changing fonts and styles.
- Making tables and graphs.
- Inserting links and bookmarks.
- Exporting PDFs to various formats.
- Password protection and encryption are being added as security measures.
Let's get started with GcPdf by creating a simple PDF document.
How to Begin with GcPdf?
Make sure you have Visual Studio or your favourite C# development environment installed before we begin. In addition, you must include the GrapeCity Documents for PDF NuGet package (GcPdf) in your project.
Making a Basic PDF Document
In this example, we'll make a simple PDF file with text and a rectangle shape.
using System;
using GrapeCity.Documents.Pdf;
using GrapeCity.Documents.Text;
class Program
{
static void Main(string[] args)
{
// Create a new PDF document
var doc = new GcPdfDocument();
// Add a page to the document
var page = doc.NewPage();
// Create a graphics object for drawing on the page
var g = page.Graphics;
// Add content to the page
var text = "Hello, World!";
var font = StandardFonts.Helvetica;
var fontSize = 24;
var textFormat = new TextFormat()
{
Font = font,
FontSize = fontSize,
};
g.DrawString(text, textFormat, new PointF(100, 100));
// Create a rectangle
var rect = new RectangleF(100, 200, 200, 150);
g.DrawRectangle(rect, Color.Red);
// Specify the file path where you want to save the PDF
var filePath = "example.pdf";
// Save the document to a PDF file
doc.Save(filePath);
Console.WriteLine($"PDF created at {filePath}");
}
}
Explanation of the code
- To represent the PDF document, we construct a new GcPdfDocument object.
- Using doc, add a page to the document.NewPage().
- Make a graphics object (g) to doodle on the page.
- Using g, add text and a rectangle to the page.DrawString() and g.DrawRectangle() are two functions.
- Enter the location to the file where you wish to save the PDF.
- doc Save the document as a PDF file.Save().
After running this code, a PDF file named "example.pdf" will be created in your project directory.
GcPdf Advanced Features
GcPdf has a wide range of tools for creating advanced PDF documents. Here are a few advanced features to look into:
Including Images
Using the g.DrawImage() method, you can add images to your PDF document. This enables you to insert logos, images, or photographs in your documents.
var image = Image.FromFile("logo.png");
g.DrawImage(image, new RectangleF(50, 50, 100, 100));
Making Tables
Tables are widely used to present tabular data in PDF documents. GcPdf includes a Table class that may be used to create tables with a variety of formatting choices.
var table = new Table();
table.DataSource = GetTableData(); // Replace with your data source
page.Elements.Add(table);
Adding Hyperlinks
You can include hyperlinks in your PDFs using the g.DrawString() method with a link destination.
var hyperlinkText = "Visit our website";
var linkDestination = new LinkDestinationURI("https://example.com");
g.DrawString(hyperlinkText, textFormat, new PointF(100, 300), linkDestination);
PDF Security
GcPdf allows you to secure your PDFs by adding passwords or encrypting them. You can set document permissions and control who can view or edit the document.
var options = new PdfSaveOptions
{
Security = new PdfSecuritySettings
{
OwnerPassword = "owner_password",
UserPassword = "user_password",
Permissions = PdfPermissions.Print | PdfPermissions.Copy,
},
};
doc.Save("secure.pdf", options);
Creating customized PDFs for various applications in .NET C# by using GcPdf programmatically is a potent and versatile method. GcPdf offers all the necessary features and flexibility to generate reports, invoices, or other types of documents quickly and efficiently. To enhance your PDF generation capabilities with more in-depth information and examples, please refer to the GcPdf documentation. We wish you happy coding!