This article overview action result which are used in ASP.NET Core and Core API. We will understand both, which are available in two different assemblies of ASP.NET Core Microsoft.AspNetCore.Mvc and System.Web.Http.
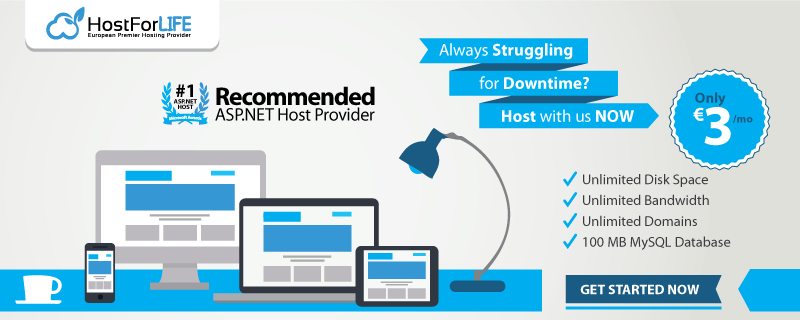
ObjectResult
ObjectResult primary role is content negotiation. It has some variation of a method called SelectFormatter on its ObjectResultExecutor. You can return an object with it, and it formats the response based on what the user requested in the Accept header. If the header didn’t exist, it returns the default format configured for the app. It’s important to note that if the request is issued through a browser, the Accept header will be ignored, unless we set the RespectBrowserAcceptHeader to true when we configure the MVC options in Startup.cs. Also, it doesn’t set the status code, which causes the status code to be null. ObjectResult is the super type of following:
AcceptedResult
AcceptedAtActionResult
AcceptedAtRouteResult
BadRequestObjectResult
CreatedResult
CreatedAtActionResult
CreatedAtRouteResult
NotFoundObjectResult
OkObjectResult
AcceptedResult
An AcceptedResultthat returns an Accepted (202) response with a Location header. It indicates that the request is successfully accepted for processing, but it might or might not acted upon. In this case, we should redirect the user to a location that provides some kind of monitor on the current state of the process. For this purpose, we pass a URI.
public AcceptedResult AcceptedActionResult()
{
return Accepted(new Uri("/Home/Index", UriKind.Relative), new { FirstName = "Peter",LastName="Scott" });
}
AcceptedAtActionResult
An AcceptedAtActionResult action result returns an accepted 202 response with a location header.
public AcceptedAtActionResult AcceptedAtActionActionResult()
{
return AcceptedAtAction("IndexWithId", "Home", new { Id = 2, area = "" }, new { FirstName = "Peter",LastName="Scott" });
}
AcceptedAtRouteResult
An AcceptedAtRouteResult returns an Accepted (202) response with a Location header. It's the same as AcceptedResult, with the only difference being that it takes a route name and route value instead of URI.
public AcceptedAtRouteResult AcceptedAtRouteActionResult()
{
return AcceptedAtRoute("default", new { Id = 2, area = "" }, new { FirstName = "Peter", LastName = "Scott" });
}
BadRequestResult
An ObjectResult, when executed. will produce a Bad Request (400) response. It indicates a bad request by user. It does not take any argument.
public BadRequestResult BadRequestActionResult()
{
return BadRequest();
}
BadRequestObjectResult
This is similar to BadRequestResult, with the difference that it can pass an object or a ModelStateDictionary containing the details regarding the error.
public BadRequestObjectResult BadRequestObjectActionResult()
{
var modelState = new ModelStateDictionary();
modelState.AddModelError("Name", "Name is required.");
return BadRequest(modelState);
}
CreatedResult
CreatedResult returns a Created (201) response with a Location header. This indicates the request has been fulfilled and has resulted in one or more new resources being created.
public CreatedResult CreatedActionResult()
{
return Created(new Uri("/Home/Index", UriKind.Relative), new { FirstName = "Peter", LastName = "Scott" });
}
CreatedAtActionResult
CreatedAtActionResult that returns a Created (201) response with a Location header.
public CreatedAtActionResult CreatedAtActionActionResult()
{
return CreatedAtAction("IndexWithId", "Home", new { id = 2, area = "" }, new { FirstName = "Peter", LastName = "Scott" });
}
CreatedAtRouteResult
CreatedAtRouteResult that returns a Created (201) response with a Location header.
public CreatedAtRouteResult CreatedAtRouteActionResult()
{
return CreatedAtRoute("default", new { Id = 2, area = "" }, new { FirstName = "Peter", LastName = "Scott" });
}
NotFoundResult
This represents a StatusCodeResult that when executed, will produce a Not Found (404) response.
public NotFoundResult NotFoundActionResult()
{
return NotFound();
}
NotFoundObjectResult
This is similar to NotFoundResult, with the difference being that you can pass an object with the 404 response.
public NotFoundObjectResult NotFoundObjectActionResult()
{
return NotFound(new { Id = 1, error = "There was no customer with an id of 1." });
}
OkResult
This is a StatusCodeResult. When executed, it will produce an empty Status200OK response.
public OkResult OkEmptyWithoutObject()
{
return Ok();
}
OkObjectResult
An ObjectResult, when executed, performs content negotiation, formats the entity body, and will produce a Status200OK response if negotiation and formatting succeed.
public OkObjectResult OkObjectResult()
{
return new OkObjectResult(new { Message="Hello World !"});
}
NoContentResult
The action result returns 204 status code. It’s different from EmptyResult in that EmptyResult returns an empty 200 status code, but NoContentResult returns 204. Use EmptyResult in normal controllers and NoContentResult in API controllers.
public NoContentResult NoContentActionResult()
{
return NoContent();
}
StatusCodeResult
StatusCodeResult accepts a status code number and sets that status code for the current request. One thing to point is that you can return an ObjectResult with and status code and object. There is a method on ControllerBase called StatusCode (404, new {Name = "Peter Scott”}), which can take a status code and an object and return an ObjectResult.
public StatusCodeResult StatusCodeActionResult()
{
return StatusCode(404);
}