
October 20, 2020 08:37 by
Peter
In this article we'll have a look at different lifetime options we have registering service via built-in IoC container provided in .net core. As an example we'll use code provided in one of my previous articles.
To quiclky recap we have a Quartz.Net job which depends on a service.
private readonly IDemoService _demoService;
public DemoJob(IDemoService demoService)
{
_demoService = demoService;
}
Instead of injecting DemoService directly we provide IDemoService abstraction which DemoJob depends upon.
Understanding service lifetimes
In the abovementioned article, we have registered our services with scoped lifetime.
var serviceCollection = new ServiceCollection();
serviceCollection.AddScoped<DemoJob>();
serviceCollection.AddScoped<IDemoService, DemoService>();
var serviceProvider = serviceCollection.BuildServiceProvider();
However, there is no actual thinking presented here as to why we have chosen it over other options such as transient or singleton lifetime.
Let’s examine the other options. In order to achieve this, we’ll add some trace statements to our class constructors.
public DemoService()
{
Console.WriteLine("DemoService started");
}
And the job constructor:
public DemoJob(IDemoService demoService, IOptions<DemoJobOptions> options)
{
_demoService = demoService;
_options = options.Value;
Console.WriteLine("Job started");
}
The service registration is as follows,
serviceCollection.AddTransient<DemoJob>();
serviceCollection.AddTransient<IDemoService, DemoService>();
After we run the program we’ll observe the following output,
DemoService started
Job started
calling http://i.ua
DemoService started
Job started
calling http://i.ua
DemoService started
Job started
calling http://i.ua
The output is pretty self-explanatory: We create a new instance each time we call service. Changing both registrations to AddScoped or AddSingleton produces the same result,
DemoService started
Job started
calling http://i.ua
calling http://i.ua
calling http://i.ua
Both instances are constructed just once at application startup. Let’s consult with the documentation to see what are the difference between those lifetimes and why the produce the same result for a given example.
Scoped lifetime services are created once per client request (connection).
Here is what singleton does.
Singleton lifetime services are created the first time they’re requested.
So in our case, we have a single request because we use console application. This is the reason why both service lifetimes act the same.
The last topic most of DI-related articles do not cover is a composition of services with different lifetimes. Although there is something worth mentioning. Here is the example of registration.
serviceCollection.AddSingleton<DemoJob>();
serviceCollection.AddTransient<IDemoService, DemoService>();
This means that we inject transient dependency into singleton service. One might expect that since we declared IDemoService as transient it will be constructed each time.
The output, however, is quite different,
DemoService started
Job started
calling http://i.ua
calling http://i.ua
calling http://i.ua
So again both services are constructed at the application startup. Here we see that lifetime of transient service gets promoted by the service that uses it. This leads to an important application. The service we’ve registered as transient might be not be designed to be used as a singleton because it is not written in thread-safe fashion or for some other reasons. However, it becomes singleton in this case which may lead to some subtle bugs. This brings us to the conclusion that we shouldn’t register services as singletons unless we have some good reason for it; i.e., service that manages global state. It’s preferable to register services as transient.
The opposite, however, yields no surprises.
serviceCollection.AddTransient<DemoJob>();
serviceCollection.AddSingleton<IDemoService, DemoService>();
produces
DemoService started
Job started
calling http://i.ua
Job started
calling http://i.ua
Job started
calling http://i.ua
Here each new instance of a job reuses the same singleton DemoService.
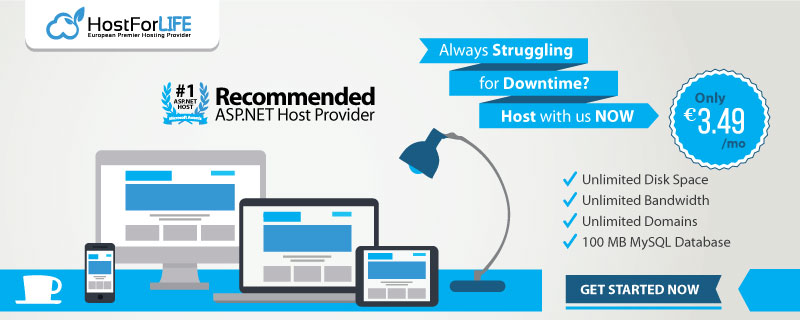

October 16, 2020 09:45 by
Peter
In ASP.NET Core, whenever we inject a service as a dependency, we must register this service to ASP.NET Core Dependency Injection container. However, registering services one by one is not only tedious and time-consuming, but it is also error-prone. So here, we will discuss how we can register all the services at once dynamically. To register all of the services dynamically, we will use TanvirArjel.Extensions.Microsoft.DependencyInjection library. This is a small but extremely useful library that enables you to register all your services into ASP.NET Core Dependency Injection container at once without exposing the service implementation.
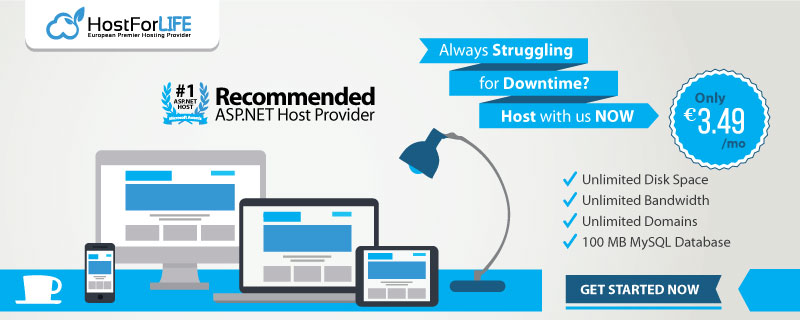
First, install the latest version of TanvirArjel.Extensions.Microsoft.DependencyInjection NuGet package into your project as follows,
Install-Package TanvirArjel.Extensions.Microsoft.DependencyInjection
Using Marker Interface
Now let your services inherit any of the ITransientService, IScoperService, and ISingletonService marker interfaces as follows,
using TanvirArjel.Extensions.Microsoft.DependencyInjection
// Inherit `IScopedService` interface if you want to register `IEmployeeService` as scoped service.
public class IEmployeeService : IScopedService
{
Task CreateEmployeeAsync(Employee employee);
}
internal class EmployeeService : IEmployeeService
{
public async Task CreateEmployeeAsync(Employee employee)
{
// Implementation here
};
}
ITransientService, IScoperService, and ISingletonService are available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Using Attribute
Now mark your services with any of the ScopedServiceAttribute, TransientServiceAttribute, and SingletonServiceAttribute attributes as follows,
using TanvirArjel.Extensions.Microsoft.DependencyInjection
// Mark with ScopedServiceAttribute if you want to register `IEmployeeService` as scoped service.
[ScopedService]
public class IEmployeeService
{
Task CreateEmployeeAsync(Employee employee);
}
internal class EmployeeService : IEmployeeService
{
public async Task CreateEmployeeAsync(Employee employee)
{
// Implementation here
};
}
ScopedServiceAttribute, TransientServiceAttribute, and SingletonServiceAttribute are available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Now in your ConfigureServices method of the Startup class,
public void ConfigureServices(IServiceCollection services)
{
services.AddServicesOfType<IScopedService>();
services.AddServicesWithAttributeOfType<ScopedServiceAttribute>();
}
AddServicesOfType<T> is available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Moreover, if you want only specific assemblies to be scanned during type scanning,
public static void ConfigureServices(IServiceCollection services)
{
// Assemblies start with "TanvirArjel.Web", "TanvirArjel.Application" will only be scanned.
string[] assembliesToBeScanned = new string[] { "TanvirArjel.Web", "TanvirArjel.Application" };
services.AddServicesOfType<IScopedService>(assembliesToBeScanned);
services.AddServicesWithAttributeOfType<ScopedServiceAttribute>(assembliesToBeScanned);
}
That's it! The job is done! It is as simple as above to dynamically register all your services into ASP.NET Core Dependency Injection container at once. If you have any issues, you can submit it to the Github Repository of this library. You will be helped as soon as possible.

October 8, 2020 08:45 by
Peter
Validating user input is a basic function in a web application. For production systems, developers usually spend a lot of time writing a lot of code to complete this function. If we use Fluent Validation to build the ASP.NET Core Web API, the task of input validation will be much easier than before. Fluent Validation is a very popular. NET library for building strong type validation rules.
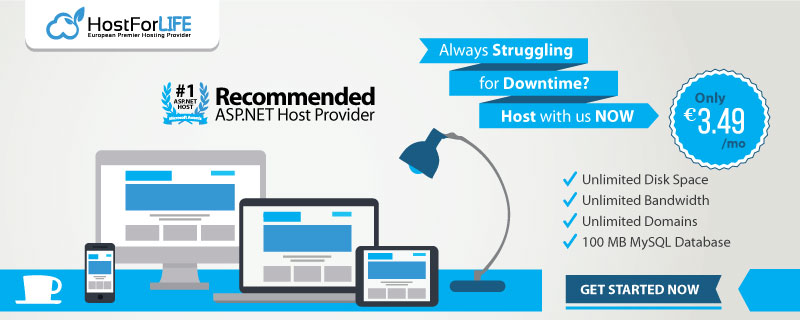
Configuration project
Step 1: Download fluent validation
We can use nuget to download the latestFluentValidationlibrary
PM> Install-Package FluentValidation.AspNetCore
Step 2: Add the Fluent Validation service
We need to be in the ____________Startup.csAdd Fluent Validation Service to File
public void ConfigureServices(IServiceCollection services)
{
// mvc + validating
services.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1)
.AddFluentValidation();
}
Adding Checker
FluentValidationA variety of built-in calibrators are provided. In the following examples, we can see two of them.
NotNull Checker
NotEmpty Checker
Step 1: Add a data model that needs to be validated
Now let’s add oneUserClass.
public class User
{
public string Gender { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string SIN { get; set; }
}
Step 2: add verifier class
UseFluentValidationTo create a validator class, the validator class needs to inherit from an abstract classAbstractValidator
public class UserValidator : AbstractValidator<User>
{
public UserValidator()
{
//Add rules here
}
}
Step 3: Add validation rules
In this example, we need to verify that FirstName, LastName, SIN can’t be null, can’t be empty. We also need to verify that the SIN (Social Insurance Number) number is legitimate.
public static class Utilities
{
public static bool IsValidSIN(int sin)
{
if (sin < 0 || sin > 999999998) return false;
int checksum = 0;
for (int i = 4; i != 0; i--)
{
checksum += sin % 10;
sin /= 10;
int addend = 2 * (sin % 10);
if (addend >= 10) addend -= 9;
checksum += addend;
sin /= 10;
}
return (checksum + sin) % 10 == 0;
}
}
Here we areUserValidatorClass, add validation rules
public class UserValidator : AbstractValidator<User>
{
public UserValidator()
{
RuleFor(x => x.FirstName)
.NotEmpty()
.WithMessage("FirstName is mandatory.");
RuleFor(x => x.LastName)
.NotEmpty()
.WithMessage("LastName is mandatory.");
RuleFor(x => x.SIN)
.NotEmpty()
.WithMessage("SIN is mandatory.")
.Must((o, list, context) =>
{
if (null != o.SIN)
{
context.MessageFormatter.AppendArgument("SIN", o.SIN);
return Utilities.IsValidSIN(int.Parse(o.SIN));
}
return true;
})
.WithMessage("SIN ({SIN}) is not valid.");
}
}
Step 4: Injecting authentication services
public void ConfigureServices(IServiceCollection services)
{
// Add validator
services.AddSingleton<IValidator<User>, UserValidator>();
// mvc + validating
services
.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1)
.AddFluentValidation();
}
Step 5:Startup.csManage your validation errors
In ASP.NET Core 2.1 and above, you can override the default behavior (ApiBehavior Options) managed by ModelState.
public void ConfigureServices(IServiceCollection services)
{
// Validators
services.AddSingleton<IValidator<User>, UserValidator>();
// mvc + validating
services
.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1)
.AddFluentValidation();
// override modelstate
services.Configure<ApiBehaviorOptions>(options =>
{
options.InvalidModelStateResponseFactory = (context) =>
{
var errors = context.ModelState
.Values
.SelectMany(x => x.Errors
.Select(p => p.ErrorMessage))
.ToList();
var result = new
{
Code = "00009",
Message = "Validation errors",
Errors = errors
};
return new BadRequestObjectResult(result);
};
});
}
When data model validation fails, the program executes this code.
In this example, I set up how to display errors to the client. In the returned result here, I just include an error code, error message and error object list.
Let’s take a look at the final results.
Using Verifier
Verifier is very easy to use here.
You just need to create an action and put the data model that needs to be validated into the action parameters.
Since the authentication service has been added to the configuration, when this action is requested,FluentValidationYour data model will be validated automatically!
Step 1: Create an action using the data model to be validated
[Route("api/[controller]")]
[ApiController]
public class DemoValidationController : ControllerBase
{
[HttpPost]
public IActionResult Post(User user)
{
return NoContent();
}
}