
October 16, 2020 09:45 by
Peter
In ASP.NET Core, whenever we inject a service as a dependency, we must register this service to ASP.NET Core Dependency Injection container. However, registering services one by one is not only tedious and time-consuming, but it is also error-prone. So here, we will discuss how we can register all the services at once dynamically. To register all of the services dynamically, we will use TanvirArjel.Extensions.Microsoft.DependencyInjection library. This is a small but extremely useful library that enables you to register all your services into ASP.NET Core Dependency Injection container at once without exposing the service implementation.
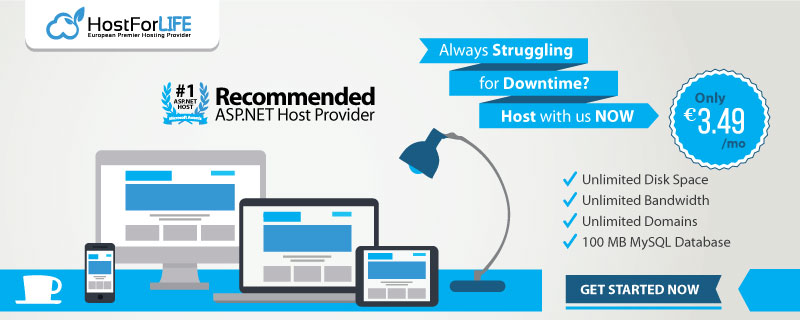
First, install the latest version of TanvirArjel.Extensions.Microsoft.DependencyInjection NuGet package into your project as follows,
Install-Package TanvirArjel.Extensions.Microsoft.DependencyInjection
Using Marker Interface
Now let your services inherit any of the ITransientService, IScoperService, and ISingletonService marker interfaces as follows,
using TanvirArjel.Extensions.Microsoft.DependencyInjection
// Inherit `IScopedService` interface if you want to register `IEmployeeService` as scoped service.
public class IEmployeeService : IScopedService
{
Task CreateEmployeeAsync(Employee employee);
}
internal class EmployeeService : IEmployeeService
{
public async Task CreateEmployeeAsync(Employee employee)
{
// Implementation here
};
}
ITransientService, IScoperService, and ISingletonService are available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Using Attribute
Now mark your services with any of the ScopedServiceAttribute, TransientServiceAttribute, and SingletonServiceAttribute attributes as follows,
using TanvirArjel.Extensions.Microsoft.DependencyInjection
// Mark with ScopedServiceAttribute if you want to register `IEmployeeService` as scoped service.
[ScopedService]
public class IEmployeeService
{
Task CreateEmployeeAsync(Employee employee);
}
internal class EmployeeService : IEmployeeService
{
public async Task CreateEmployeeAsync(Employee employee)
{
// Implementation here
};
}
ScopedServiceAttribute, TransientServiceAttribute, and SingletonServiceAttribute are available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Now in your ConfigureServices method of the Startup class,
public void ConfigureServices(IServiceCollection services)
{
services.AddServicesOfType<IScopedService>();
services.AddServicesWithAttributeOfType<ScopedServiceAttribute>();
}
AddServicesOfType<T> is available in TanvirArjel.Extensions.Microsoft.DependencyInjection namespace.
Moreover, if you want only specific assemblies to be scanned during type scanning,
public static void ConfigureServices(IServiceCollection services)
{
// Assemblies start with "TanvirArjel.Web", "TanvirArjel.Application" will only be scanned.
string[] assembliesToBeScanned = new string[] { "TanvirArjel.Web", "TanvirArjel.Application" };
services.AddServicesOfType<IScopedService>(assembliesToBeScanned);
services.AddServicesWithAttributeOfType<ScopedServiceAttribute>(assembliesToBeScanned);
}
That's it! The job is done! It is as simple as above to dynamically register all your services into ASP.NET Core Dependency Injection container at once. If you have any issues, you can submit it to the Github Repository of this library. You will be helped as soon as possible.