In this post we are going to talk about techniques for displaying data contained in the SQL Server database with ASP.NET. There are at least three ways commonly used to perform the data binding to server conrol in ASP.NET 4.5
Here are three ways:
- Data Source Control (Declarative)
but in this time I will explain how to create a database with the Data Source Control in ASP.NET 4.5
Web Config
Before going into the main discussion helps me informed beforehand that the connection string that is used to communicate with the database in ASP.NET contained in the configuration file "web.config". Here are the contents
file: Web.config
<?xml version="1.0"?>
<configuration>
<connectionStrings> <add name="learn_webConnectionString" connectionString="Data Source=SOFT-ENGINEER;Initial Catalog=learning_web;Integrated Security=True" providerName="System.Data.SqlClient" />
</connectionStrings>
<system.web>
<compilation debug="true" targetFramework="4.5" />
<httpRuntime targetFramework="4.5" />
</system.web>
</configuration>
Database Design
The database used in this post only use one of the table is very simple with the name "Category"
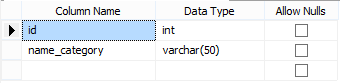
Data Source Control
Data Source Control in ASP.NET 4.5 used as a link between the database with controls for displaying data. Using this approach means that we do is get the data in a declarative rather than programmatic. To be more explicit, the following is a sample code
file: default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="TryingWebForm.Default" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>Data Source Control</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<p>The Declarative Way</p>
<asp:SqlDataSource ID="sdsCategory" runat="server" ConnectionString="<%$ ConnectionStrings:trying_webConnectionString %>"
SelectCommand="SELECT [id], [name_category] FROM [Category]"/>
<asp:GridView ID="gvCategoryDeclarative" runat="server" DataSourceID="sdsCategory" />
</div>
</form>
</body>
</html>
As seen in the above code, control "SqlDataSource" requires a connection string and a query command to be executed. In magic this control will connect to the database and execute commands query and the result was thrown into "GridView" by filling property "DataSourceID" with the Id of control SqlDataSource concerned. The image below is the result
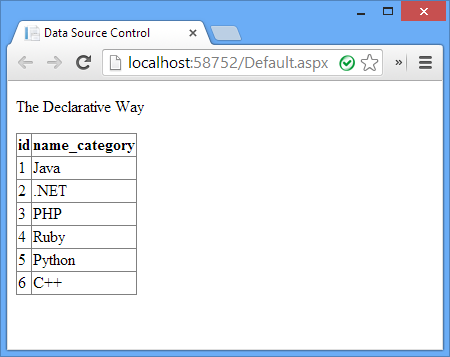
HostForLIFE.eu ASP.NET 4.5 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
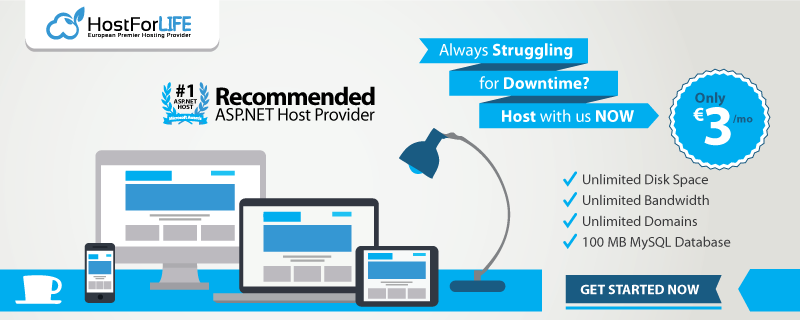