Although users today have faster Internet connection, light web pages and fast load are still popular. If your ASP.NET page is heavy, it will take some time to load and you risk that user will close browser window or go to some faster web site. Also, fast page load is respected by Google and other search engines. Pages that load fast are ranked higher in search results and because of that get more visits. With reduced page size, bandwidth costs area also reduced. So, if small page size is so important, let's take a look on how is possible to reduce size of page without removing useful content.
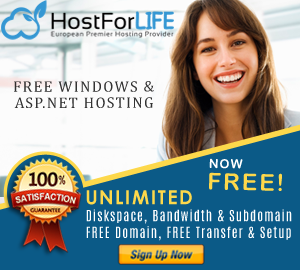
1. Optimize Images for Web
Let start with basic things. If you are placed image from digital camera and just set width and height of IMG tag to smaller values that action will not resize an image (although will appear smaller on page). So, use Photoshop, Paint Shop Pro or Resize image automatically with .Net code. Photoshop has feature named "Save For Web & Devices..." which helps to optimize images, especially if you are not professional graphic designer.
Another important issue with images on web page is using of backgrund image in page layout. Try to avoid large image in background, use few smaller images or if possible one small image with background-repeat parameter. Example code that repeat small background image inside a TABLE could look like this:
<table style="background-image:url('Sunflowers.jpg');background-repeat:repeat;" width="500" height="500">
<tr>
<td>here is a table content</td>
</tr>
</table>
Notice that using a lot of small images will increase number of http requests to server that can increase time to load. The solution is using of CSS sprites. Place all background images on one image, then use CSS background-image and background-position property to display only wanted image part.
2. Disable, Minimize or Move ViewState
ViewState can grow large if you have many controls on page, especially if data controls like GridView used. The best option is to turn off ViewState or at least not use it for every control. To disable ViewState use EnableViewState="false" in Page directive, with code like this:
<%@ Page EnableViewState="false" Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
If you must use ViewState for some controls, then turn it off for other controls. Every control has a property named EnableViewState. Set this property to false for every control that not require ViewState. On this way ViewState hidden field will be smaller and page will load faster.
Another option is to move ViewState from page to Session object. This approach will reduce page size, but ViewState will take space in memory on server. If you have a lot of concurrent visitors this could be performance problem. Take care that visitor can look at the two or more pages at the same time, so ViewState of every page must be stored to separated Session object. You can try this method with code like this:
[ C# ]
protected override object LoadPageStateFromPersistenceMedium()
{
// Returns page's ViewState from Session object
return Session["ViewState-" + Request.Url.AbsolutePath];
}
protected override void SavePageStateToPersistenceMedium(object state)
{
// Saves page's ViewState to Session object
Session["ViewState-" + Request.Url.AbsolutePath] = state;
// Removes content of ViewState on page
RegisterHiddenField("__VIEWSTATE", "");
}
[ VB.NET ]
Protected Overrides Function LoadPageStateFromPersistenceMedium() As Object
' Returns page's ViewState from Session object
Return Session("ViewState-" & Request.Url.AbsolutePath)
End Function
Protected Overrides Sub SavePageStateToPersistenceMedium(ByVal state As Object)
' Saves page's ViewState to Session object
Session("ViewState-" & Request.Url.AbsolutePath) = state
' Removes content of ViewState on page
RegisterHiddenField("__VIEWSTATE", "")
End Sub
If web site has performance problems because of extensive use of Session object, notice that with LoadPageStateFromPersistenceMedium() and SavePageStateToPersistenceMedium functions you can load and save ViewState from any other source, like file system, database etc. Strategy like this will for sure reduce page size, but in the same time add additional processing to load and save ViewState from other medium so it should be used only when we can't disable ViewState or reduce it enough.
3. Remove Inline Styles to external .css File
If inline styles are moved from HTML to external .css file, pages will have smaller size. CSS file is loaded only first time when user visits web site. After that, it is stored in browser's cache and used to format every next page. Not only pages will load faster but you'll also have less maintenance work if styles are changed.
To link CSS file to HTML page use code like this in HEAD section:
<head runat="server">
<title>Page with external CSS file</title>
<link rel="stylesheet" type="text/css" href="Styles.css" />
</head>
4. Remove any inline JavaScript to external .js File
Like CSS styling, you can move any inline JavaScript to external .js file. On this way, JavaScript will be loaded only first time, and then cached by web browser for future use. To relate .js file to web page, add one more line in HEAD section:
<head runat="server">
<title>Page with external CSS and JS files</title>
<link rel="stylesheet" type="text/css" href="Styles.css" />
<script language="JavaScript" src="scripts/common.js"></script>
</head>
5. Use Light Page Layout
A lot of nested tables will probably make your page unnecessary large. Try use DIV tags instead of tables where possible and define DIVs appearance with CSS. On this way, HTML code will be smaller. Although many designers recommend using of DIV tags only and without any table used for layout, I am not DIV purist. On my opinion, tables are still good for columns even yooyut use table for that part. I created twelve hybrid CSS/Table/DIV Page Layouts Examples. This page layout examples use both TABLE and DIVs to get light but still robust page layout.
6. Use HTTP Compression
HTTP compression can be used to reduce number of bytes sent from server to client. If web browser supports this feature, IIS will send data compressed using GZIP.
Free ASP.NET 5 Hosting
Try our Free ASP.NET 5 Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc.
