
March 6, 2015 06:22 by
Peter
Today, In this article I will tell you about Create Web API with ASP.NET 5, run outside IIS application. First step, open visual studio and then make a console application as you can see on the picture below:
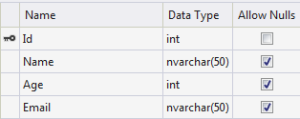
Create the Web API & OWIN Packages
Go to Tools menu, choose Library Package Manager, then select Package Manager Console. In the Package Manager Console window, enter the following code:
Install-Package Microsoft.AspNet.WebApi.OwinSelfHost
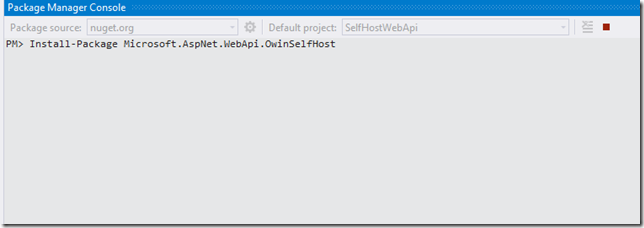
Then, right click the project. Create new class named Startup.cs and add the following code:
public class Startup
{
// This code configures Web API. The Startup class is specified as a type
// parameter in the WebApp.Start method.
public void Configuration(IAppBuilder appBuilder)
{
// Configure Web API for self-host.
HttpConfiguration config = new HttpConfiguration();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
appBuilder.UseWebApi(config);
}
}
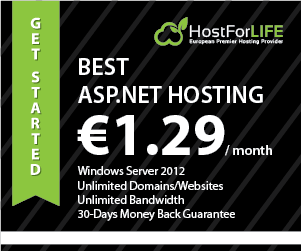
Now create a Web API controller class. In Solution Explorer, right click the project & choose Add / Class to add a new class. I named it: HelloController. Then write the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
namespace SelfHostWebApi
{
public class HelloController : ApiController
{
// GET api/values/5
public string Get(int id)
{
return "Hello API";
}
}
}
Open Program.cs and add write the code below, inside main method:
using Microsoft.Owin.Hosting;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
namespace SelfHostWebApi
{
class Program
{
static void Main(string[] args)
{
string baseAddress = "http://localhost:9000/";
// Start OWIN host
using (WebApp.Start<Startup>(url: baseAddress))
{
// Create HttpCient and make a request to api/values
HttpClient client = new HttpClient();
var response = client.GetAsync(baseAddress + "api/hello").Result;
Console.WriteLine(response);
Console.WriteLine(response.Content.ReadAsStringAsync().Result);
}
Console.ReadLine();
} }
}
Finally, your self hosted Web API is ready. You will see the output like the picture below when you Run it.
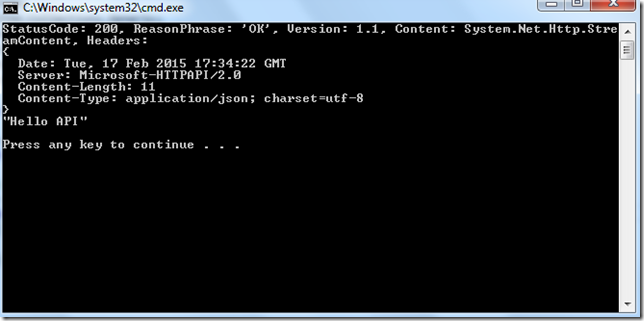
HostForLIFE.eu ASP.NET 5 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
