Writing integration tests for ASP.NET Core controller actions used for file uploads is not a rare need. It is fully supported by ASP.NET Core integration tests system. This post shows how to write integration tests for single and multiple file uploads.
Getting started
Suppose we have controller action for file upload that supports multiple files. It uses complex composite command for image file analysis and saving. Command is injected to action by framework-level dependency injection using controller action injection.
[HttpPost]
[Authorize(Roles = "Admin")]
public async Task<IActionResult> Upload(IList<IFormFile> files, int? parentFolderId,
[FromServices]SavePhotoCommand savePhotoCommand)
{
foreach(var file in files)
{
var model = new PhotoEditModel();
model.FileName = Path.GetFileName(file.FileName);
model.Thumbnail = Path.GetFileName(file.FileName);
model.ParentFolderId = parentFolderId;
model.File = file;
list.AddRange(savePhotoCommand.Validate(model));
await savePhotoCommand.Execute(model);
}
ViewBag.Messages = savePhotoCommand.Messages;
return View();
}
We want to write integration tests for this action but we need to upload at least one file to make sure that command doesn’t fail.
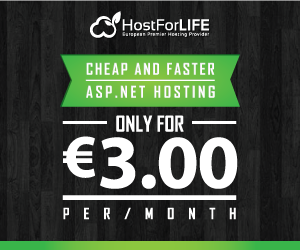
Making files available for integration tests
It’s good practice to have files for testing available no matter where tests are run. It’s specially true when writing code in team or using continuous integration server to run integration tests. If we don’t have many files and the files are not large then we can include those files in project.
Important thing is to specify in Visual Studio that these files are copied to output folder.
Same way it’s possible to use also other types of files and nobody stops us creating multiple folders or folder trees if we want to organize files better.
Uploading files in integration tests
Here is integration tests class for controller mentioned above. Right now there’s only one test and it is testing Upload action. Notice how image files are loaded from TestPhotos folder to file streams and how form data object is built using the file streams.
public class PhotosControllerTests : IClassFixture<WebApplicationFactory<Startup>>
{
private readonly WebApplicationFactory<Startup> _factory;
public PhotosControllerTests(WebApplicationFactory<Startup> factory)
{
_factory = factory;
}
[Fact]
public async Task Upload_SavesPhotoAndReturnSuccess()
{
// Arrange
var expectedContentType = "text/html; charset=utf-8";
var url = "Photos/Upload";
var options = new WebApplicationFactoryClientOptions { AllowAutoRedirect = false };
var client = _factory.CreateClient(options);
// Act
HttpResponseMessage response;
using (var file1 = File.OpenRead(@"TestPhotos\rt-n66u.jpg.webp"))
using (var content1 = new StreamContent(file1))
using (var file2 = File.OpenRead(@"TestPhotos\speedtest.png.webp"))
using (var content2 = new StreamContent(file2))
using (var formData = new MultipartFormDataContent())
{
// Add file (file, field name, file name)
formData.Add(content1, "files", "rt-n66u.jpg.webp");
formData.Add(content2, "files", "speedtest.png.webp");
response = await client.PostAsync(url, formData);
}
// Assert
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
Assert.NotEmpty(responseString);
Assert.Equal(expectedContentType, response.Content.Headers.ContentType.ToString());
response.Dispose();
client.Dispose();
}
}
For actions that accept only one file we need only one call to Add() method of formData.
Wrapping up
Integration tests mechanism in ASP.NET Core is flexible enough to support also more advanced scenarios like file uploads in tests. It’s not very straightforward and we can’t just call few methods of HTTP client to do it but it’s still easy enough once we know the tricks. If we keep test files in integration tests project then we don’t have to worry about getting files to machine where integration tests are running.