The differences between.NET 7 and.NET 8 can be better understood by contrasting their compatibility, integration powers, performance, and diagnostic features. A thorough grasp of these frameworks can also be attained by exploring the new features added in.NET 8 and comparing them with those of.NET 7. Examining these specifics can help identify the best version of the Microsoft.NET framework.
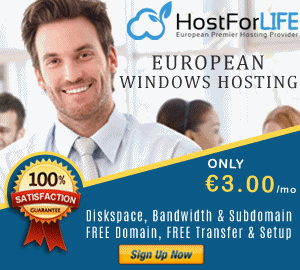
The improvements in.NET 8 constitute a noteworthy achievement for Microsoft, offering an extensive feature set with the goals of strengthening security, enhancing performance, enabling smooth integration, and streamlining maintenance. The question of whether the new features in.NET 8 are superior to those in.NET 7 is still up for dispute.
Examining the main distinctions between.NET 7 and.NET 8 is essential to obtain understanding and make wise choices about a possible upgrade. With the clarity this article will offer, it will be possible to comprehend the advantages and improvements brought forth by.NET 8.
Despite being created by Microsoft,.NET 8 and.NET 7 differ significantly. We've divided up their differences into categories based on a number of important factors, such as integration capabilities, cross-platform compatibility, performance, diagnostics, observability, and support services. It will enable you to fully comprehend how the two differ from one another.
1. Enhancements in Performance
Performance is one of the most important factors that developers consider when assessing technology for building applications. Notably,.NET 8 exhibits better optimization than.NET 7 in terms of speed and efficiency. A JIT (Just-In-Time) compiler is used by the Microsoft.NET framework in both versions, but in.NET 8, substantial improvements have been made to speed up code processing, leading to faster process execution and shorter loading times.
Furthermore, significant improvements to the runtime environment and garbage collector in.NET 8 also add to increased efficiency. With these improvements, companies can save a great deal of resources and maintain high performance even during busy hours.
2. Development Across Platforms
When it comes to facilitating the development of cross-platform compatible applications,.NET 8 outperforms.NET 7. .NET 8 is notably more capable than.NET 7 of facilitating the creation of programs customized for different hardware intrinsics.
- AVX-512
- Vector 512
- Optimized support for ARM64 and WebAssembly
These enhancements expand the accessibility of your program, making it available to a larger range of users on desktop and mobile devices, covering a variety of operating systems such as Windows, Linux, macOS, and other platforms.
3. Assistance for Platforms
For every.NET version, Microsoft offers different support durations based on two main policies: LTS (Long Term Support) and STS (Short Term Support). Long Term Support (LTS) for the.NET 8 version ensures Microsoft's assistance from November 14, 2023, to November 10, 2026. On the other hand, May 14th, 2024 is when support for the Dotnet 7 version is supposed to end. Selecting.NET 8 guarantees improved upkeep and durability for the well-being of your program.
Version |
Latest patch version |
Patch release date |
Release type |
Support phase |
End of support |
.NET 8 |
8.0.0 |
November 14, 2023 |
LTS |
Active |
November 10, 2026 |
.NET 7 |
7.0.14 |
November 14, 2023 |
STS |
Active |
May 14, 2024 |
4. Consolidation
In contrast to.NET 7, which necessitates that developers create unique code in order to integrate with APIs and other components,.NET 8 provides more convenience. Major integrated development environments, APIs, and services are all pre-supported by default. Additionally, the debugging features have been much enhanced, enabling better process execution and problem correction with less time commitment.
New Features in.NET 8
Now that we know why.NET 8 is better than.NET 7, it's imperative that you be familiar with all of its key characteristics. Comprehending these fundamental features of.NET 8 is essential as it confirms its place as a reliable technology for application development, outperforming.NET 7.
1. An improved source generator
When it comes to earlier iterations, the source generator included in.NET 8 is a major improvement. Microsoft has made significant improvements to System.Text.Json, bringing it closer to parity with the reflection-based serializer. Among the improvements included in.NET 8 are:
- Support for the "init" and "required" properties when using the source generator to serialize types.
- source-generated code formatted more neatly and systematically.
- Two new diagnoses, SYSLIB1034 and SYSLIB1039, have been added.
- Support for JsonStringEnumConverter<TEnum> is integrated into.NET 8.
2. Original AOT
By compiling only the essential parts rather than the complete codebase,.NET 8 apps improve code use with native AOT (Ahead-of-Time) compilation.
For instance, just the code specifically responsible for the login feature will be used when a user enters in with a.NET 8 application; the rest of the codebase stays in its current configuration. Thus, there are several benefits to this strategy.
- Elimination of the need for a Just-In-Time compiler.
- It increased the loading speed.
- Conservation of network and memory resources.
- Reduced execution time and resource costs.
- Improved user experience and satisfaction.
3. Enhanced Artificial Intelligence Skills
AI integration is now more stable, quick, and smooth with.NET 8. The AI components in.NET 8 are the outcome of multiple technologies working together, including Qdrant, Microsoft Teams, Azure OpenAI, Milvus, and Azure Cognitive Search. Together, these many technological advances enable.NET 8 applications to leverage sophisticated artificial intelligence (AI) capabilities, producing outputs that are accurate and exact.
4. Elevated .NET MAUI
.NET 8 brings significant enhancements to .NET MAUI. Now, a single codebase caters to API 34 and XCode 15, enabling seamless operation of your .NET MAUI application across WinUI, iOS, Android, and Mac Catalyst systems.
5. SHA-3 Hashing Primitives
Compared to .NET 7, the security enhancements in .NET 8 are notably robust. It introduces support for configuring the SHA-3 hashing algorithm, ensuring compatibility with APIs that complement SHA-3 within your .NET 8 applications. The SHA-3 cryptography algorithms available in .NET 8 include.
- SHA3_256, SHA3_384, and SHA3_512 (for Hashing purposes)
- HMACSHA3_256, HMACSHA3_384, and HMACSHA3_512 (for HMAC usage)
- HashAlgorithmName.SHA3_256, HashAlgorithmName.SHA3_384, and HashAlgorithmName.SHA3_512 (for developer-configurable algorithm settings)
- RSAEncryptionPadding.OaepSHA3_256, RSAEncryptionPadding.OaepSHA3_384, and RSAEncryptionPadding.OaepSHA3_512 (for RSA OAEP encryption)
6. HTTPS Proxy
Support: Man-in-the-middle attacks are prevented with HTTPS proxy support, which.NET 8 brings to improve the security of client connections. By creating encrypted communication channels, this function protects the confidentiality and integrity of data.
Should you upgrade from .NET 7 to .NET 8?
Considering the analysis of various factors, .NET 8 is more advanced and proficient than .NET 7. So, you should upgrade your application to .NET 8.
Conclusion
.NET 8 stands out as a remarkable software development technology by Microsoft, surpassing .NET 7 in every aspect. From performance and integration to C# code compilation, observability, and support, .NET 8 excels in every aspect when compared. Moreover, .NET experts and professionals widely endorse .NET 8 for their projects.