In web development, response compression is an essential technique used to maximize the speed and responsiveness of HTTP applications. Response compression improves user experience, speeds up page loads, and uses less bandwidth by lowering the size of HTTP responses.
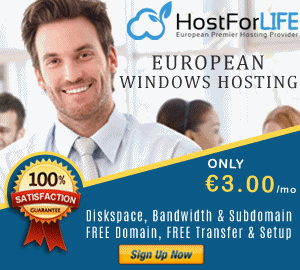
Advantages of Compression Response:
- Enhanced Performance: Compression speeds up page loads by reducing the quantity of data sent over the network.
- Decreased Bandwidth Usage: Less data transfer from compressed answers results in lower hosting and bandwidth expenses.
- Improved SEO: Page load times are taken into account by search engines, which could result in a website that loads faster ranking better in search results.
Response Compression Implementation in.NET
Middleware can be used to implement response compression in a.NET web application. This is an example that makes use of AddResponseCompression, a.NET middleware.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddResponseCompression(options =>
{
options.EnableForHttps = true;
});
var app = builder.Build();
app.UseResponseCompression();
app.MapGet("/", () => "Hello World!");
app.Run();
In this example, EnableForHttps is set to true, but caution is advised due to potential security vulnerabilities like CRIME and BREACH attacks. It's recommended to use anti-forgery tokens to mitigate these risks.
builder.Services.AddResponseCompression(options =>
{
options.EnableForHttps = true;
options.Providers.Add<BrotliCompressProvider>();
options.Providers.Add<GzipCompressProvider>();
options.MimeTypes = ResponseCompressionDefaults.MimeTypes;
});
Compression Levels
Each compression provider has different compression levels.
builder.Services.Configure<BrotliCompressionProviderOptions>(options =>
{
options.Level = CompressionLevel.SmallestSize;
});
builder.Services.Configure<GzipCompressProviderOptions>(options =>
{
options.Level = CompressionLevel.Optimal;
});
These levels include Optimal, Fastest, No Compression, and Smallest Size.
Custom Providers
You can also create custom compression providers by implementing the ICompressionProvider interface.
builder.Services.AddResponseCompression(options =>
{
options.Providers.Add<BrotliCompressionProvider>();
options.Providers.Add<GzipCompressionProvider>();
options.Providers.Add<CustomCompressionProvider>();
});
When to Compress and When not?
Compress responses that are not natively compressed (e.g., CSS, JS, HTML, XML, JSON). Avoid compressing natively compressed assets (e.g., PNG) and smaller files (150-1000 bytes).
Verifying Compression
To ensure compression is working, use middleware, set compression levels, and send requests from tools like Postman with different values of Accept-Encoding in the header.
Default MIME Types Supported
Common MIME types supported by default include text/CSS, text/XML, text/JSON, text/HTML, text/plain, application/XML, application/JSON, application/WASM, and application/javascript.
One useful method for improving the performance and user experience of web applications is to add response compression. Smaller HTTP response sizes result in quicker page loads, less bandwidth used, and possibly higher search engine ranks. A strong framework for effectively handling response compression is provided by the AddResponseCompression middleware in the.NET environment, in conjunction with providers such as Brotli and Gzip compression. It is imperative to take security concerns into account, particularly when enabling HTTPS compression. It is recommended to take steps to address potential vulnerabilities such as CRIME and BREACH attacks, such as implementing anti-forgery tokens.
Developers can adapt compression algorithms to individual application requirements thanks to the flexibility of bespoke compression providers. It is possible to adjust compression levels according to optimization, speed, or file size considerations. It's important to know when to apply compression, focusing on replies that aren't already compressed and avoiding it on smaller or previously compressed data. It is important to confirm that the compression settings you have put in place are working as intended by using tools such as Postman to verify the efficiency of the compression with different Accept-Encoding header variants.
Developers can further improve the effectiveness of response compression by covering a variety of frequently used content kinds by utilizing default MIME types for compression. To put it simply, response compression is a crucial component of web development that, when used carefully, greatly improves an application's overall functionality, responsiveness, and cost-effectiveness..