
January 8, 2024 05:58 by
Peter
An essential feature to the asynchronous programming paradigm in.NET is channels. They offer a thread-safe method of data transfer between producers and consumers, improving application performance and scalability. The System is the foundation of channels.Threading.Namespaces and channels provide an adaptable and effective way to communicate.
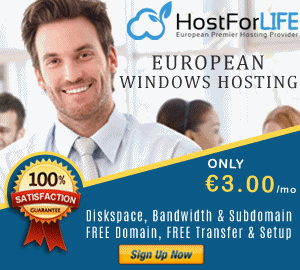
How to Make a Channel in.NET?
To get started, let's develop a basic example that shows how to use and create a channel in.NET 8.0.
using System.Threading.Channels;
Console.WriteLine("Channels In .NET");
// Create an unbounded channel
var channel = Channel.CreateUnbounded<int>();
// Producer writing data to the channel
async Task ProduceAsync()
{
for (int i = 0; i < 5; i++)
{
await channel.Writer.WriteAsync(i);
Console.WriteLine($"Produced: {i}");
}
channel.Writer.Complete();
}
// Consumer reading data from the channel
async Task ConsumeAsync()
{
while (await channel.Reader.WaitToReadAsync())
{
while (channel.Reader.TryRead(out var item))
{
Console.WriteLine($"Consumed: {item}");
}
}
}
// Run producer and consumer asynchronously
var producerTask = ProduceAsync();
var consumerTask = ConsumeAsync();
// Wait for both tasks to complete
await Task.WhenAll(producerTask, consumerTask);
Console.ReadLine();
An explanation of the example:
- Using Channel, we construct an infinite channel of integers.ConstructUnbounded<int>().
- As the producer, the ProduceAsync() method uses a channel to write data to the channel.Author.Use WriteAsync().
- As the consumer, the ConsumeAsync() method uses the channel to read data from it.Peruser.TryRead().
- Task allows both producer and consumer tasks to run asynchronously.WhenAll() to await their finish
Channel Properties in.NET
There are various options available with.NET Channels to manage data flow:
- Boundary and Non-Boundary Channels: You can decide whether to let an infinite amount of objects through or restrict the capacity of the channel.
- Completion: Use the channel to indicate that the channel is finished.Author.To indicate that the data stream has ended, use Complete().
- Cancellation: To manage the channel activities lifetime, use cancellation tokens.
- numerous Writers/Readers: Use channels to properly support numerous writers or readers.