
January 25, 2024 07:05 by
Peter
The following describes how to incorporate health checks into a.NET Core application:
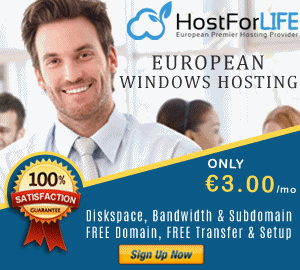
1. Set up the NuGet package for Health Checks
You must install Microsoft.Extensions.Diagnostics in your project.The NuGet package HealthChecks. You can accomplish this by include the package reference in your project file or by using the Package Manager Console:
dotnet add package Microsoft.Extensions.Diagnostics.HealthChecks
2. Configure Health Checks
In your Startup.cs file, configure the health checks in the ConfigureServices method:
using Microsoft.AspNetCore.Builder;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Diagnostics.HealthChecks;
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks();
// Add other services...
}
public void Configure(IApplicationBuilder app)
{
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapHealthChecks("/health");
// Map other endpoints...
});
}
}
A health check endpoint at /health is configured in this example.
3. Explain Health Examinations
By including checks for other components, you can define health checks. You may wish to verify the state of an external API, a database connection, or any other crucial element. These checks can be included in the ConfigureServices method:
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks()
.AddSqlServer("YourConnectionString", name: "DatabaseHealthCheck")
.AddUrlGroup(new Uri("https://api.example.com"), name: "ApiHealthCheck");
// Add other services...
}
4. Execute and Verify
Launch your application at this point, and go to the health check endpoint (http://localhost:5000/health, for example). A JSON response with the status of each health check ought to appear.
In summary
- The status of each check and any further information you supply are usually included in health check responses.
- Health checks can be modified and expanded to meet your unique needs.
It's a good idea to include health checks if you want to make sure that your.NET Core application is reliable, particularly in production scenarios where you want to keep an eye on any problems and take proactive measures to address them.