Values that cannot be changed at runtime in C# are declared using the readonly and const keywords. However, there are several notable distinctions between these two words.
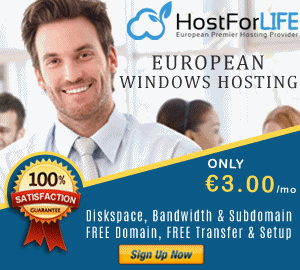
Constant: Describe It
Const is a compile-time constant, meaning that its value can only be set at compile time and cannot be modified by runtime. Usually, it is used for declaring global constants, such as mathematical constants or other values that aren't expected to change throughout program execution. Const values are intrinsically static and always belong to the class; they are not particular to any one instance of the class. Since they are by nature public, they can also be accessed from anywhere in the code.
public class Constants
{
public const string CLIENT_ID = "ClientId";
}
ReadOnly: What Is It?
Conversely, readonly fields are runtime constants, which means that only the class's constructor has the ability to modify them. Their value is predetermined at runtime. Since the readonly fields are instance-level variables, a duplicate of each field exists in each class instance. Additionally, they can be marked as static, indicating that they are part of the class itself rather than a specific class instance. You have to specifically declare the access level because the readonly fields are not inherently public.
public class TestService
{
private readonly ISomeService _someService;
public TestService(ISomeService someService)
{
_someService = someService;
}
}
Key distinctions in C# between readonly and constant:
Constants in C# are defined using the readonly and const keywords, although their applications and capabilities differ.
When their values are set is one of the biggest distinctions between readonly and const. Values defined with the const keyword must be set at compile time and will not change at runtime. It implies that const values are decided upon during code compilation rather than execution. Conversely, readonly values are set at runtime and can only have their values assigned within the class constructor.
Const values are also fundamentally different since they are always implicitly static—that is, they are part of the class and not an instance of it. On the other hand, readonly values need to specifically state the access level and can be either instance-level or static.
The following example demonstrates the distinctions between readonly and const:
public class TestClass
{
public const double PI = 3.14;
public readonly string Name;
public TestClass(string name)
{
Name = name;
}
}
In this case, the const value PI was set to 3.14 at compile time. However, Name is a read-only variable that is set in the class's constructor and can only be changed during runtime.
The way that const and readonly are accessible is another distinction between them. Readonly values require the access level to be explicitly specified, whereas const variables are always implicitly public.
While read-only variables are determined at runtime, const values are computed at compile time.
While read-only fields might be instance-level or static and require the access level to be explicitly specified, const values are always static and automatically public.
Readonly fields can only be modified within the class constructor, but const values cannot be altered at runtime.
ReadOnly vs Constant
ReadOnly keyword |
Const keyword |
The readonly keyword in C# can be used to create readonly fields. |
The const keyword in C# is used to construct constant fields. |
The runtime constant ReadOnly. |
A compile-time constant is called const. |
It is possible to modify the readonly field's value. |
It is not possible to modify the value of the const field. |
It can't be declared within the procedure. |
It may be specified within the procedure. |
We can set values in the constructor and declaration sections of read-only fields. |
We can only assign values to the declaration part of const fields. |
The use of static modifiers is possible. |
Static modifiers are incompatible with its use. |
When should you use CONSTANTS versus READONLY?
Utilize constants when the values are absolute, fixed, and do not vary, such as DaysOfWeek, MonthsOfYear, mathematical PI value, and so on. Use readonly when the variables are obtained via user input, configuration files, or any other variable that does not have the same value, such as a database connection string, a secret key, and so on.
We learned the new technique and evolved together.