
April 2, 2024 08:18 by
Peter
Creating reliable and secure online apps requires careful consideration of authentication and authorization. Microsoft Identity offers a strong foundation for implementing authorization and authentication features with ease in the.NET ecosystem. In this post, we'll look at how to use Microsoft Identity in conjunction with controllers to efficiently handle user registration and login functions in a.NET API.
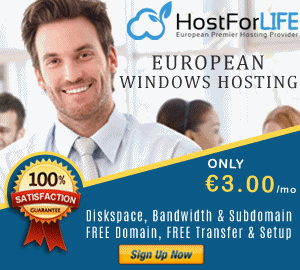
Required conditions
Make sure you have installed the following prerequisites before continuing:
- The.NET SDK (at least version 5)
- Visual Studio Code (optional) or Visual Studio
Establishing the Project
Let's start by making a new.NET Web API project:
dotnet new webapi -n YourProjectName
cd YourProjectName
Adding Identity to the Project
To add Identity to your project, run the following commands:
dotnet add package Microsoft.AspNetCore.Identity.EntityFrameworkCore
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
This adds the necessary packages to your project for using Identity and Entity Framework Core with SQL Server.
Scaffold Identity
Next, scaffold Identity into your project:
dotnet aspnet-codegenerator identity -dc YourDbContext
Replace YourDbContext with the name of your application's DbContext.
Implementing Registration and Login Controllers:
Now, let's implement controllers for user registration and login.
1. Registration Controller
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
using YourProjectName.Models;
namespace YourProjectName.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class RegisterController : ControllerBase
{
private readonly UserManager<ApplicationUser> _userManager;
public RegisterController(UserManager<ApplicationUser> userManager)
{
_userManager = userManager;
}
[HttpPost]
public async Task<IActionResult> Register(RegisterModel model)
{
var user = new ApplicationUser { UserName = model.Email, Email = model.Email };
var result = await _userManager.CreateAsync(user, model.Password);
if (result.Succeeded)
{
return Ok("Registration successful");
}
return BadRequest(result.Errors);
}
}
}
2. Login Controller
using Microsoft.AspNetCore.Identity;
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
using YourProjectName.Models;
namespace YourProjectName.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class LoginController : ControllerBase
{
private readonly SignInManager<ApplicationUser> _signInManager;
public LoginController(SignInManager<ApplicationUser> signInManager)
{
_signInManager = signInManager;
}
[HttpPost]
public async Task<IActionResult> Login(LoginModel model)
{
var result = await _signInManager.PasswordSignInAsync(model.Email, model.Password, false, false);
if (result.Succeeded)
{
return Ok("Login successful");
}
return Unauthorized("Invalid email or password");
}
}
}
Models
Ensure you have the necessary models for registration and login:
namespace YourProjectName.Models
{
public class RegisterModel
{
public string Email { get; set; }
public string Password { get; set; }
}
public class LoginModel
{
public string Email { get; set; }
public string Password { get; set; }
}
}
Configuring Startup
Finally, add Identity services to the ConfigureServices method in Startup.cs:
services.AddIdentity<ApplicationUser, IdentityRole>()
.AddEntityFrameworkStores<YourDbContext>()
.AddDefaultTokenProviders();
Happy coding!