
March 25, 2024 10:23 by
Peter
ASP.NET is a reliable platform for web development, allowing developers to easily create dynamic and resilient web applications. At the heart of ASP.NET is Razor, a powerful templating engine that transforms the way developers create server-side code. Razor syntax provides a concise and easy way to combine server-side code and HTML, increasing productivity and maintainability. Let's go deeper into Razor syntax and see how it can improve your ASP.NET programming experience.
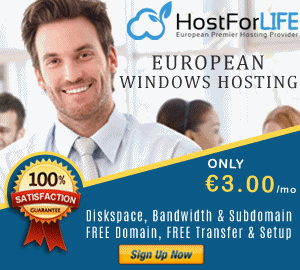
Understanding Razor syntax
Razor syntax seamlessly integrates server-side code into HTML markup, resulting in cleaner and more understandable code than typical ASP.NET Web Forms. Its lightweight and fluid design allows developers to seamlessly switch between HTML and server-side code, resulting in a more efficient development process.
Razor syntax uses a set of special characters (@) to represent server-side code blocks in HTML markup. These code blocks can include C# or VB.NET code, allowing developers to execute logic, get data from databases, modify objects, and conduct a variety of other operations right within the markup.
Benefits of Razor Syntax
- Readable and Maintainable Code: Razor syntax promotes code readability by minimizing the clutter typically associated with embedding server-side logic within HTML. The clean and concise syntax enhances code maintainability, making it easier for developers to understand and modify code segments as needed.
- Seamless Integration: Unlike traditional ASP.NET Web Forms, which often necessitate a clear demarcation between server-side and client-side code, Razor syntax seamlessly integrates server-side logic with HTML markup. This cohesive approach simplifies development and fosters a more fluid coding experience.
- Enhanced Productivity: By streamlining the process of writing server-side code within HTML, Razor syntax boosts developer productivity significantly. Its intuitive nature reduces cognitive overhead, allowing developers to focus on implementing business logic rather than wrestling with cumbersome syntax.
- Code Reusability: Razor syntax facilitates code reusability by enabling developers to encapsulate common functionality within reusable components known as partial views. These partial views can be invoked from multiple pages, promoting a modular and DRY (Don't Repeat Yourself) coding approach.
- IntelliSense Support: Integrated development environments (IDEs) such as Visual Studio provide robust IntelliSense support for Razor syntax, offering real-time suggestions and auto-completion for server-side code blocks. This feature enhances code efficiency and reduces the likelihood of syntax errors.
Example of Razor Syntax
<!DOCTYPE html>
<html>
<head>
<title>ASP.NET Razor Syntax</title>
</head>
<body>
<div>
<h1>Welcome, @Model.Name!</h1>
<p>Your account balance is: [email protected]</p>
@if (Model.IsPremium)
{
<p>Congratulations! You are a premium member.</p>
}
else
{
<p>Upgrade to premium for exclusive benefits.</p>
}
</div>
</body>
</html>
In this example, Razor syntax is used to embed server-side code within an HTML document. The @Model directive accesses properties of the model passed to the view, allowing dynamic content to be rendered based on the provided data.