
March 19, 2024 08:58 by
Peter
Deadlocks are a prevalent problem in concurrent programs, and they can be especially troublesome for programs written in C# and running on.NET Core. A deadlock arises when two or more threads are waiting for each other to release resources that are required to proceed. This can lead to all threads becoming stuck and unable to progress, causing the program to freeze. Many distinct conditions can result in deadlocks, and developers must be aware of them in order to avoid or reduce the danger of deadlocks.
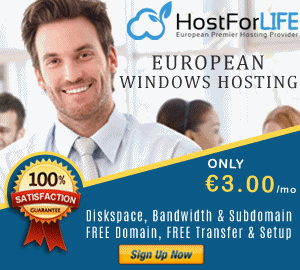
For example, one common circumstance is that two threads each have a resource that the other thread requires. Another instance is when one thread is waiting for a lock to be released while another thread holds that lock and waits for the first thread to release a different lock. These circumstances can be difficult to identify and resolve, but with careful analysis and development techniques, deadlocks can be avoided or reduced.
What is a deadlock?
A deadlock occurs when two or more threads are stalled indefinitely, each waiting for the other to relinquish a resource that it requires to move forward. Deadlocks are characterized by a circular waiting pattern in which no thread can continue, resulting in a stalemate.
using System;
using System.Threading;
class Program
{
static object ResourceA = new object();
static object ResourceB = new object();
static void Thread1()
{
lock (ResourceA)
{
Console.WriteLine("Thread1 acquired ResourceA");
Thread.Sleep(100); // Simulating some work
lock (ResourceB)
{
Console.WriteLine("Thread1 acquired ResourceB");
}
}
}
static void Thread2()
{
lock (ResourceB)
{
Console.WriteLine("Thread2 acquired ResourceB");
Thread.Sleep(100); // Simulating some work
lock (ResourceA)
{
Console.WriteLine("Thread2 acquired ResourceA");
}
}
}
static void Main(string[] args)
{
Thread t1 = new Thread(Thread1);
Thread t2 = new Thread(Thread2);
t1.Start();
t2.Start();
t1.Join();
t2.Join();
Console.WriteLine("Program completed successfully.");
}
}
Understanding The Deadlock
In this case, Thread1 locks ResourceA first and then attempts to lock ResourceB, whereas Thread2 locks ResourceB first and then attempts to lock ResourceA. If Thread1 obtains ResourceA while Thread2 obtains ResourceB, they will both be waiting for the other resource, resulting in a stalemate.
Preventing Deadlocks
To prevent deadlocks, you can employ various strategies:
- Lock Ordering: Always acquire locks in a consistent order to prevent circular dependencies.
- Lock Timeout: Use Monitor.TryEnter or Mutex.WaitOne with a timeout to avoid indefinite blocking.
- Lock Hierarchy: Establish a lock hierarchy and always acquire locks in the same order within that hierarchy.
- Avoid Nested Locks: Minimize the use of nested locks to reduce the risk of deadlocks.
Conclusion
Deadlocks can be challenging to debug and resolve, but understanding their causes and employing preventive measures can help mitigate their occurrence. In C# and .NET Core, careful design and coding practices, along with thorough testing, are essential for creating robust and reliable concurrent applications. By following best practices and being mindful of potential deadlock scenarios, developers can ensure the smooth execution of their multithreaded code.