
April 16, 2024 08:45 by
Peter
You can add the Experimental property to your types, methods, or assemblies to designate that a feature is experimental using the new Experimental attribute in C# 12. When someone tries to use the method or the type after you've done this, the compiler will throw an error. I describe how to use this functionality in this article.
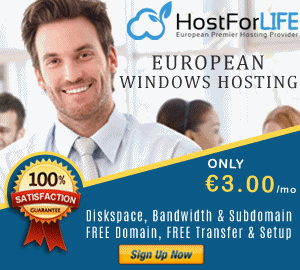
I used Visual Studio 2022 Preview to construct a console application with.NET 8 and run it for demonstration purposes. Use of the Preview version is required until the article's date. This feature will not function otherwise.
The System.Diagnostics.CodeAnalysis namespace is where the Experimental attribute originates. You can see an example of a class with this attribute in the code below:
using System.Diagnostics.CodeAnalysis;
namespace DotNet8Examples
{
[Experimental(diagnosticId: "Test001")]
public class ExperimentalAttributeDemo
{
public void Print()
{
Console.WriteLine("Hello Experimental Attribute");
}
}
}
On line 1, there is the namespace for the Experimental attribute.
On line 5, there is the Experimental attribute, with the diagnosticId. For the diagnosticId, you can specify an Id, which will be used by the compiler, to report a usage of the API the attribute applies to.
On line 8, there is a method for this class.
Now, if you try to create an instance of this class, the compiler will complain about it:
var experimentalAttributeDemo = new ExperimentalAttributeDemo();

It should be noted that the Experimental property can be used in both the method and the class. You can use the NoWarn property in the.csproj file to use this class and ignore this error; this will work on a global level, so each time you try to use the class or method, you won't have to suppress the error; alternatively, you can suppress it directly on the code, which will require you to do so each time you need to use the class or method.
I'm directly suppressing the error Test001 in the code in the example below. In order to do it, you must add your code in between the #pragma warning disable Test001 and the #pragma warning restore Test001: