In this short tutorial, I will tell you about submit form without page refresh in ASP.NET with Ajax and jQuery. First step, make a Employee table as shown on the picture below:
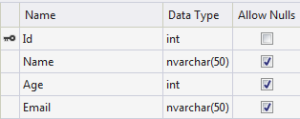
Now, make a new WebSite then add a new WebForm name as Index.aspx. Write the following code to the page.
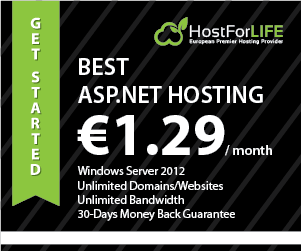
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Index.aspx.cs" Inherits="Index" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script src="Script/jquery-2.1.1.min.js"></script>
<script src="Script/Myjs.js"></script>
</head>
<body>
<form id="form1" runat="server">
<div>
<table>
<tr>
<td>Name</td>
<td>
<input type="text" id="txtName" />
</td>
</tr>
<tr>
<td>Age</td>
<td>
<input type="text" id="txtAge" />
</td>
</tr>
<tr>
<td>Email</td>
<td>
<input type="text" id="txtEmail" />
</td>
</tr>
<tr>
<td>
<input type="button" id="btnSubmit" value="Save" />
</td>
</tr>
</table>
<label id="lblResult" ></label>
</div>
</form>
</body>
</html>
Now, Open file Index.aspx.cs and write the following code:
using System;
using System.Web.Services;
using System.Data.SqlClient;
public partial class Index : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static string SaveRecord(string Name,int Age, string Email)
{
string messageResult = string.Empty;
string strConnection = @"Data Source=(LocalDB)\v11.0;AttachDbFilename=C:\Users\Peter\TestDB.mdf;Integrated Security=True;Connect Timeout=30";
SqlConnection con = new SqlConnection(strConnection);
con.Open();
SqlCommand cmd = new SqlCommand("insert into Employee (Name,Age,Email) values (@name,@age,@email)",con);
cmd.Parameters.AddWithValue("@name", Name);
cmd.Parameters.AddWithValue("@age",Age);
cmd.Parameters.AddWithValue("@email", Email);
int result = cmd.ExecuteNonQuery();
con.Close();
if(result == 1)
{
messageResult = "Record Inserted";
}
else
{
messageResult="Unable to Save Record";
}
return messageResult;
}
}
Above you can see, I have included a attribute [WebMethod]. What is WebMethod ? A WebMethod Attribute Enable a system to be called through the WebServices. Now, include another JS record. Compose the accompanying code in your JS document.
$(document).ready(function () {
$('#btnSubmit').on('click', function () {
var getName = $('#txtName').val();
var getAge = parseInt($('#txtAge').val());
var getEmail = $('#txtEmail').val();
$.ajax(
{
type: "POST",
contentType: "application/json; charset=utf-8",
url: "Index.aspx/SaveRecord",
data: "{'Name':'" + getName + "','Age':" + getAge + ",'Email':'" + getEmail + "'}",
dataType: "json",
success:function(data)
{
$('#lblResult').text(data.d);
$('#txtName').val('');
$('#txtAge').val('');
$('#txtEmail').val('');
},
error:function(data)
{
alert(data);
}
});
});
});
And here is the output:
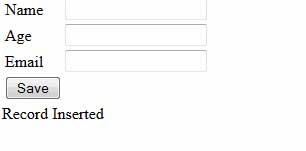
HostForLIFE.eu ASP.NET 5 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
