Email address are the means of communication with people around the world. While processing forms email address validation plays an important. Proper email validation strengthen our contact list, ban spamming and protect us from robot form filling (Form AutoFill).
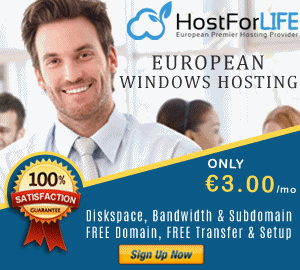
Here we are going to design a regular expression pattern to validate email address which will check to make sure an e-mail address is a valid address, and in proper format means containing an username, at sign (@), and valid hostname. For example, [email protected] is valid, but SPAM@badhost is invalid. Most of email service provides limit the use of literals for email address creation. Only letters (a-z,A-Z), numbers (0-9), hyphens (-), underscore (_) and periods (.) are allowed and no special characters are accepted. You can add or remove any literals to your regular expression.
Regular Expression Pattern
^([a-zA-Z0-9_\-\.]+)@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$
email with IP -
^([a-zA-Z0-9_\-\.]+)@(([a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3}))|(([01]?\d\d?|2[0-4]\d|25[0-5])\.){3}([01]?\d\d?|25[0-5]|2[0-4]\d))$
A description of the regular expression:
1]: A numbered capture group. [[a-zA-Z0-9_\-\.]+]
Any character in this class: [a-zA-Z0-9_\-\.], one or more repetitions
@
Any character in this class: [a-z0-9-], one or more repetitions
[2]: A numbered capture group. [\.[a-z0-9-]+], any number of repetitions
\.[a-z0-9-]+
Literal .
Any character in this class: [a-z0-9-], one or more repetitions
[3]: A numbered capture group. [\.[a-z]{2,3}]
\.[a-z]{2,3}
Literal .
Any character in this class: [a-z], between 2 and 3 repetitions
Sucessful Matches:
[email protected]
[email protected]
[email protected]
email with IP
[email protected]
[email protected]
How It Works:
This regular expression will check for valid email address in which Only letters (a-z,A-Z), numbers (0-9), hyphens (-), underscore (_) and periods (.) are allowed and no special characters are accepted. Here we are going to search three group and @ sign.
First first group will check valid conbination of characters (a-z,A-Z), numbers (0-9), hyphens (-), underscore (_) and periods (.) followed by "@". Then we check for host name(tipsntracks,yahoo, google etc) and host type(.com, .biz, .co.in etc)
ASP.NET
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="Default.aspx.vb" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>U.S. Social Security Numbers</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<span>Valid Format: 123-45-6789</span><br />
<asp:TextBox id="txtInput" runat="server"></asp:TextBox><br />
<asp:RegularExpressionValidator Id="vldRejex" RunAt="server" ControlToValidate="txtInput" ErrorMessage="Please enter a valid email address" ValidationExpression="^([a-zA-Z0-9_\-\.]+)@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$">
</asp:RegularExpressionValidator><br />
<asp:Button Id="btnSubmit" RunAt="server" CausesValidation="True" Text="Submit"></asp:Button>
</div>
</form>
</body>
</html>
C#.NET
//use System.Text.RegularExpressions befour using this function
public bool vldEmail(string emlAddress)
{
//create Regular Expression Match pattern object
Regex myRegex = new Regex("^([a-zA-Z0-9_\\-\\.]+)@[a-z0-9-]+(\\.[a-z0-9-]+)*(\\.[a-z]{2,3})$");
//boolean variable to hold the status
bool isValid = false;
if (string.IsNullOrEmpty(emlAddress))
{
isValid = false;
}
else
{
isValid = myRegex.IsMatch(emlAddress);
}
//return the results
return isValid;
}
VB.NET
‘Imports System.Text.RegularExpressions befour using this function
Public Function vldEmail(ByVal emlAddress As String) As Boolean
‘create Regular Expression Match pattern object
Dim myRegex As New Regex("^([a-zA-Z0-9_\-\.]+)@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$")
‘boolean variable to hold the status
Dim isValid As Boolean = False
If emlAddress = "" Then
isValid = False
Else
isValid = myRegex.IsMatch(emlAddress)
End If
‘return the results
Return isValid
End Function
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
