
January 6, 2016 21:10 by
Peter
The errors and exceptions are going to be written to a text file because it is simpler to search out the reason for the error because the Error Log text file are often easily opened using the basic notepad application in Windows.
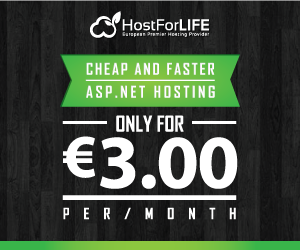
The following HTML Markup consists of an ASP.NET Button control which will raise an Exception and the exception (error) Message details will be displayed.
<asp:Button Text="Click to Raise Exception" runat="server" OnClick="RaiseException"/>
Namespaces
You will need to import the following namespace.
C#
using System.IO;
VB.Net
Imports System.IO
Creating simple Error Log Text File in ASP.NET
The following event handler is raised when the Button is clicked. An exception is raised by converting a string value to integer inside a Try-Catch block.
The raised Exception is caught in the Catch block and the LogError function is called.
Inside the LogError function, the details of the exception (error) are written to an Error Log Text file along with current date and time.
C#
protected void RaiseException(object sender, EventArgs e)
{
try
{
int i = int.Parse("Mudassar");
}
catch (Exception ex)
{
this.LogError(ex);
}
}
private void LogError(Exception ex)
{
string message = string.Format("Time: {0}", DateTime.Now.ToString("dd/MM/yyyy hh:mm:ss tt"));
message += Environment.NewLine;
message += "-----------------------------------------------------------";
message += Environment.NewLine;
message += string.Format("Message: {0}", ex.Message);
message += Environment.NewLine;
message += string.Format("StackTrace: {0}", ex.StackTrace);
message += Environment.NewLine;
message += string.Format("Source: {0}", ex.Source);
message += Environment.NewLine;
message += string.Format("TargetSite: {0}", ex.TargetSite.ToString());
message += Environment.NewLine;
message += "-----------------------------------------------------------";
message += Environment.NewLine;
string path = Server.MapPath("~/ErrorLog/ErrorLog.txt");
using (StreamWriter writer = new StreamWriter(path, true))
{
writer.WriteLine(message);
writer.Close();
}
}
VB.Net
Protected Sub RaiseException(sender As Object, e As EventArgs)
Try
Dim i As Integer = Integer.Parse("Mudassar")
Catch ex As Exception
Me.LogError(ex)
End Try
End Sub
Private Sub LogError(ex As Exception)
Dim message As String = String.Format("Time: {0}", DateTime.Now.ToString("dd/MM/yyyy hh:mm:ss tt"))
message += Environment.NewLine
message += "-----------------------------------------------------------"
message += Environment.NewLine
message += String.Format("Message: {0}", ex.Message)
message += Environment.NewLine
message += String.Format("StackTrace: {0}", ex.StackTrace)
message += Environment.NewLine
message += String.Format("Source: {0}", ex.Source)
message += Environment.NewLine
message += String.Format("TargetSite: {0}", ex.TargetSite.ToString())
message += Environment.NewLine
message += "-----------------------------------------------------------"
message += Environment.NewLine
Dim path As String = Server.MapPath("~/ErrorLog/ErrorLog.txt")
Using writer As New StreamWriter(path, True)
writer.WriteLine(message)
writer.Close()
End Using
End Sub
And here is the output:
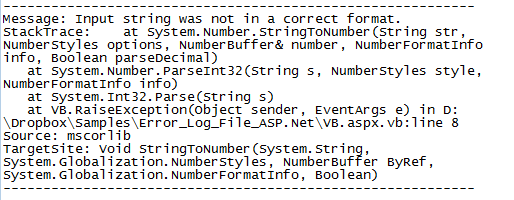
HostForLIFE.eu ASP.NET 4.6 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
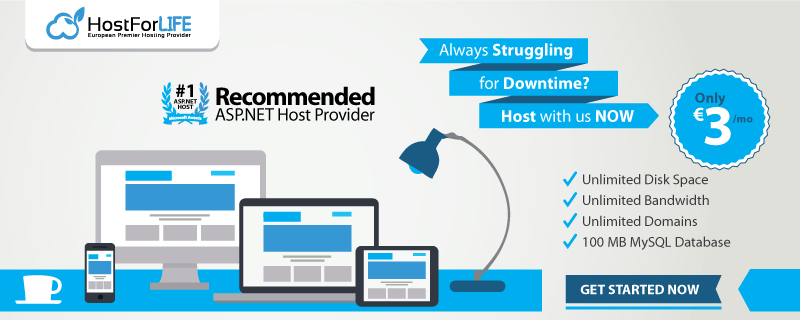