Optimization of performance is an important part of software development. As developers, we frequently attempt to write code that is efficient and speedy. However, precisely assessing code performance can be difficult. This is where BenchmarkDotNet, a powerful.NET benchmarking library, comes in. In this post, we'll look at the capabilities of BenchmarkDotNet and show you how to use it to test your.NET programs.
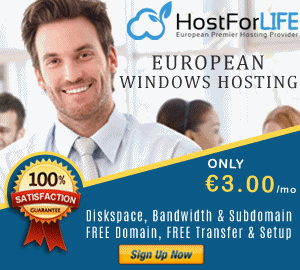
What exactly is BenchmarkDotNet?
BenchmarkDotNet is an open-source.NET benchmarking library. It enables developers to create, execute, and evaluate benchmarks in order to assess the performance of their programs. BenchmarkDotNet is a vital tool for optimizing.NET applications since it provides precise measurements, many run modes, and statistical analysis.
Starting Over
Before we begin benchmarking, please confirm that BenchmarkDotNet is installed in your project. You may install it with the following command in the NuGet Package Manager Console:
dotnet add package BenchmarkDotNet
Creating Your First BenchmarkLet's create a simple benchmark to compare the performance of two different implementations of a function. Consider the following example where we want to find the factorial of a number. Now you create the ConsoleApp.
Create a Class name FactorialCalculator
public class FactorialCalculator
{
public int CalculateRecursive(int n)
{
if (n == 0 || n == 1)
{
return 1;
}
return n * CalculateRecursive(n - 1);
}
public int CalculateIterative(int n)
{
int result = 1;
for (int i = 1; i <= n; i++)
{
result *= i;
}
return result;
}
}
Let's use BenchmarkDotNet to create benchmarks for these methods and measure their performance.
using BenchmarkDotNet.Attributes;
using BenchmarkDotNet.Running;
using ConsoleApp2;
class Program
{
static void Main(string[] args)
{
var summary = BenchmarkRunner.Run<FactorialBenchmarks>();
}
}
public class FactorialBenchmarks
{
private readonly FactorialCalculator _calculator = new FactorialCalculator();
[Benchmark]
public void RecursiveBenchmark()
{
_calculator.CalculateRecursive(10);
}
[Benchmark]
public void IterativeBenchmark()
{
_calculator.CalculateIterative(10);
}
}
In this example, we've created two benchmarks, RecursiveBenchmark and IterativeBenchmark, using the Benchmark attribute. The BenchmarkRunner.Run method triggers the benchmarks and generates a detailed report.
Running Benchmarks
Build in RELEASE Mode
- Open a terminal or command prompt.
- Navigate to the project directory using the cd command.
Run the following command to build the project in RELEASE mode:
Run Benchmarks
After building the project in RELEASE mode, you can run your benchmarks using the dotnet run command.
When building your project, it is crucial to do so in RELEASE mode in order to guarantee that the compiler applies optimizations. This will lead to more precise benchmark results. By following this suggestion, you can avoid receiving warning messages and anticipate dependable performance measurements for your benchmarks.
When you have run it then you see

It may take some time for the results to display after running the application.
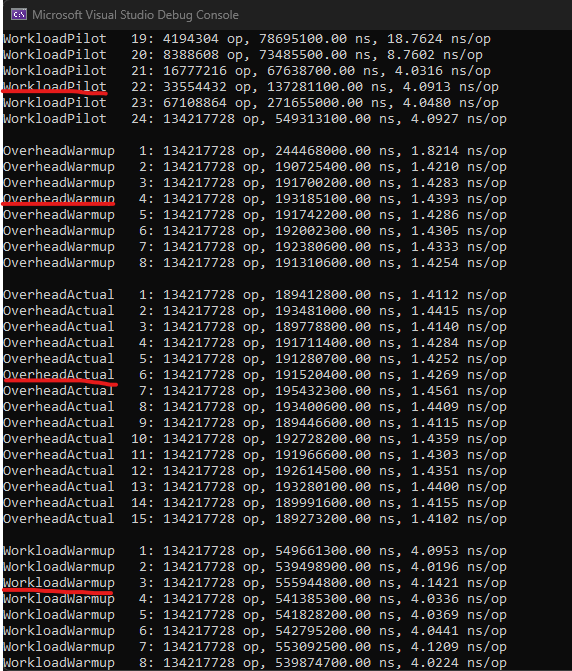
It may take some time for the results to display after running the application.
- WorkloadPilot is the code you want to measure.
- OverheadWarmup and OverheadActual account for the time and resources consumed by BenchmarkDotNet itself.
- WorkloadWarmup measures the time it takes for your code to stabilize during the warm-up phase.
- WorkloadActual measures the actual performance of your code once it has reached a stable state.
Result Summary Section
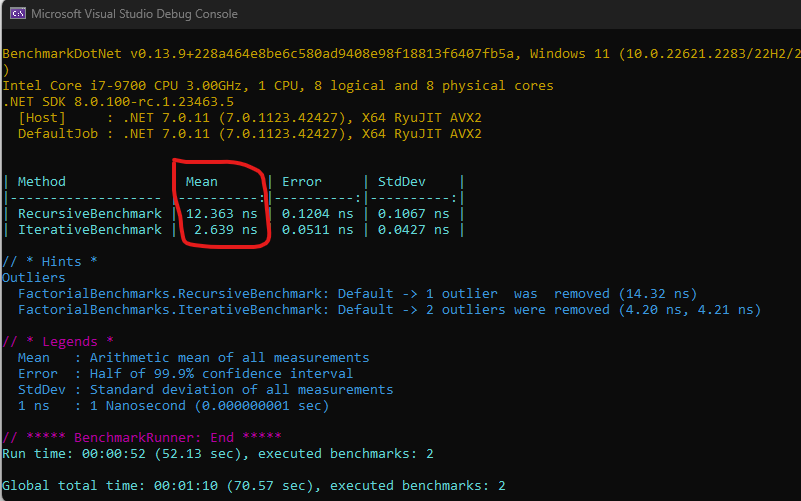
The two methods we're evaluating are listed in the overview section, along with their performance metrics. The mean measure, which is the average time it takes to run each procedure, is the most essential metric.
Conclusion
BenchmarkDotNet streamlines the process of measuring performance in.NET applications. It enables developers to find bottlenecks and improve their code effectively by delivering reliable data and statistical analysis. Consider incorporating BenchmarkDotNet into your workflow as you continue to develop high-performance applications to guarantee your code runs as efficiently as possible.