Asynchronous programming has become an essential aspect of modern software development, and.NET Core (now known as.NET 5,.NET 6,.NET 7, and later.NET 8) provides strong capabilities to make asynchronous processes easier. When working with tasks, you have two fundamental methods at your disposal: WaitAll and WhenAll. These methods enable you to efficiently manage and coordinate several asynchronous operations. In this blog post, we will look at WaitAll and WhenAll in.NET Core and learn about their differences and applications.
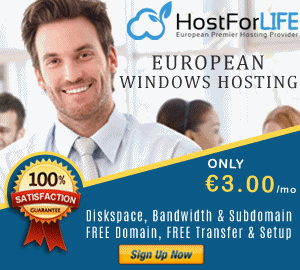
The Fundamentals of.NET Core Asynchronous Programming
Before diving into WaitAll and WhenAll, it's critical to understand the principles of.NET Core asynchronous programming. Asynchronous operations allow your application to run numerous tasks at the same time without interrupting the main thread, which improves responsiveness and speed. You deal with tasks in.NET Core, which represent units of work that can run concurrently.
public async Task DoSomeWorkAsync()
{
// Perform asynchronous operations
await Task.Delay(1000); // Simulate work
// Continue with other operations
}
WaitAll - Waiting for All Tasks to Complete
Task.WaitAll is a synchronous method that blocks the current thread until all the provided tasks have completed. It ensures that all tasks finish their execution before allowing the program to proceed further. This is particularly useful when you need to perform actions only when all tasks are done.
async Task PerformMultipleOperationFromWaitllAsync()
{
WaitAllSample waitAllSample = new WaitAllSample();
Task task1 = waitAllSample.DoSomeWorkAsync();
Task task2 = waitAllSample.DoSomeOtherWorkAsync();
Task.WaitAll(task1, task2);
Console.WriteLine("All tasks in WaitAll are complete!");
}
Using Task, on the other hand.WaitAll can cause thread blocking and may not be appropriate in all cases, particularly in UI applications where blocking the main thread can result in a frozen interface.
WhenAll - Concurrently Awaiting All Tasks
WhenAll is an asynchronous method that returns a new task that finishes when all of the specified tasks have completed. Rather of blocking the thread, it lets the computer to continue processing other activities or managing user input, which is essential for responsive apps.
async Task PerformMultipleOperationFromWhenAllAsync()
{
WhenAllExample whenallexample = new WhenAllExample();
Task task3 = whenallexample.DoSomethingAsync();
Task task4 = whenallexample.DoSomethingElseAsync();
await Task.WhenAll(task3, task4);
Console.WriteLine("All tasks in WhenAll are complete!");
}
Task.WhenAll is better suited for cases in which numerous asynchronous operations must be performed concurrently without blocking the main thread. It is essential for developing responsive and efficient applications.
Important distinctions between WaitAll and WhenAll
Task.WaitAll is a synchronous method that causes the current thread to be blocked, whereas Task.Because WhenAll is asynchronous, additional processes can execute concurrently.
When you wish to wait for all tasks to finish before proceeding, Task.WaitAll is useful, whereas Task.WhenAll allows you to continue performing other tasks while waiting for tasks to complete.
Task.WaitAll may cause thread-blocking, rendering it inappropriate for UI applications, whereas Task.WhenAll is better suited for responsive interfaces.
Task.WaitAll is older and is frequently used in legacy programs, whereas Task.WhenAll is the ideal and modern method of managing many tasks in.NET Core.
Conclusion
Asynchronous programming is a useful technique in.NET Core for improving application responsiveness and performance. Task.Task and WaitAll.WhenAll methods are critical for managing and coordinating several asynchronous tasks. You may make informed selections when selecting the correct strategy for your specific circumstance if you understand their distinctions and use cases. .NET Core delivers the tools you need to build efficient and responsive apps, whether you need to wait for all actions to complete synchronously or proceed with other processes simultaneously.