
February 13, 2024 07:19 by
Peter
Keyed services are a major improvement to the built-in dependency injection (DI) framework of.NET 8. This functionality, which has long been a part of third-party DI frameworks like Autofac and StructureMap, gives developers more freedom and control over how dependencies are delivered to their applications. Now let's explore keyed services in more detail: Understanding Keyed Services
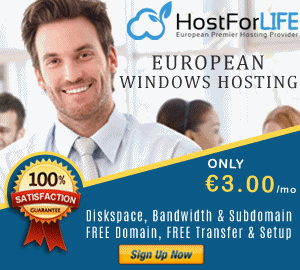
In the past,.NET DI registered services exclusively on the basis of their kind. Although this worked well in most cases, it became less successful when there were several implementations of the same interface. This is mitigated by keyed services, which enable us to link a special "key" to every service registration. An enum, string, or any other item that specifically defines the intended implementation can be used as this key.
Advantages of Services with Keys
- Flexibility: You can inject particular implementations according to runtime circumstances, configuration settings, or dynamic conditions.
- Decoupling: Implement different versions of the same interface for distinct uses to keep concerns apart.
- Maintainability: Clearly name and reference desired dependencies in your code to improve readability.
- Configurability: Apply several implementations according to configuration files or environment variables.
Consider a scenario where an application requires data storage because of configuration settings or user preferences. We have two implementations of the IDataStore interface: LocalStorage and CloudStorage.
1. Explain interfaces
public interface IDataStore
{
void SaveData(string data);
}
public class LocalStorage : IDataStore
{
public void SaveData(string data)
{
// Implementation to save data locally
Console.WriteLine($"Saving data locally: {data}");
}
}
public class CloudStorage : IDataStore
{
public void SaveData(string data)
{
// Implementation to save data in the cloud
Console.WriteLine($"Saving data in the cloud: {data}");
}
}
2. Register Services with Keys
// In Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDataStore, LocalStorage>(key: "local");
services.AddSingleton<IDataStore, CloudStorage>(key: "cloud");
}
3. Inject and Use Keyed Service
public class MyService
{
private readonly IKeyedServiceProvider _provider;
private readonly IConfiguration _configuration;
public MyService(IKeyedServiceProvider provider, IConfiguration configuration)
{
_provider = provider;
_configuration = configuration;
}
public void DoSomething()
{
string storageType = _configuration["StorageType"]; // e.g., "local" or "cloud"
IDataStore store = _provider.GetKeyedService<IDataStore>(storageType);
store.SaveData("This data will be saved based on the configuration.");
}
}
4. Run the Application
// Program.cs
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
// Configure services and app...
var app = builder.Build();
// Run the app...
}
With their more sophisticated and adaptable approach to dependency injection, keyed services are a great addition to the.NET 8 DI architecture. You can gain more authority and clarity over the design of your program by comprehending their advantages, usage trends, and sophisticated applications. Learn about, play with, and take use of keyed services to create more flexible, dynamic, and organized.NET applications!