The Visitor Pattern is used in an ASP.NET Core Web API with a 3-tier design for efficient data manipulation in the C# Article model. The model, CSharpArticle, contains critical features. A Data Access Layer with a repository manages database interactions, a Business Layer with the Visitor interface and Article Service, and a Presentation Layer with the API controllers comprise the architecture. In the Business Layer, the Visitor Pattern is used to conduct operations on articles, allowing for clean separation of responsibilities and enabling reusable, structured, and scalable code. This architecture ensures that CRUD activities benefit from the flexibility of the Visitor Pattern while preserving a clear division of responsibilities across the application layers.
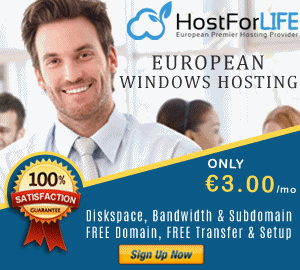
Overview of Three-Tier Architecture
The Presentation Layer manages the API controllers and interacts with the client.
The business logic, data validation, and any special business rules are all contained in the business layer.
Data Access Layer: Manages data retrieval and storage, as well as database interaction.
Article Model in C#
Let's start with the Article model.
public class CSharpArticle
{
public int Id { get; set; }
public string Title { get; set; }
public string Content { get; set; }
}
Data Access Layer
Repository Interface:
public interface IArticleRepository
{
CSharpArticle GetArticleById(int id);
void AddArticle(CSharpArticle article);
void UpdateArticle(CSharpArticle article);
void DeleteArticle(int id);
}
Repository Implementation:
public class ArticleRepository: IArticleRepository
{
private readonly CSharpCornerArticleProject _context;
public ArticleRepository(YourDbContext context)
{
_context = context;
}
public CSharpArticle GetArticleById(int id)
{
return _context.Articles.FirstOrDefault(a => a.Id == id);
}
public void AddArticle(CSharpArticle article)
{
_context.Articles.Add(article);
_context.SaveChanges();
}
public void UpdateArticle(CSharpArticle article)
{
_context.Articles.Update(article);
_context.SaveChanges();
}
public void DeleteArticle(int id)
{
var article = _context.Articles.FirstOrDefault(a => a.Id == id);
if (article != null)
{
_context.Articles.Remove(article);
_context.SaveChanges();
}
}
public void Accept(IArticleVisitor visitor, int articleId)
{
var article = GetArticleById(articleId);
visitor.Visit(article);
UpdateArticle(article);
}
}
Business Layer
Visitor Interface
public interface IArticleVisitor
{
void Visit(CSharpArticle article);
}
public class ContentAnalyzerVisitor: IArticleVisitor
{
public void Visit(CSharpArticle article)
{
if (article != null)
{
int wordCount = CountWords(article.Content);
bool hasKeywords = CheckForKeywords(article.Content);
article.WordCount = wordCount;
article.HasKeywords = hasKeywords;
}
}
private int CountWords(string content)
{
if (string.IsNullOrWhiteSpace(content))
return 0;
string[] words = content.Split(new char[] { ' ', '.', ',', ';', '!', '?' }, StringSplitOptions.RemoveEmptyEntries);
return words.Length;
}
private bool CheckForKeywords(string content)
{
string[] keywordsToCheck = { "C#", "ASP.NET", "Entity Framework" }; if needed
foreach (string keyword in keywordsToCheck)
{
if (content.Contains(keyword, StringComparison.OrdinalIgnoreCase))
{
return true;
}
}
return false;
}
}
Article Service:
public class ArticleService
{
private readonly IArticleRepository _repository;
public ArticleService(IArticleRepository repository)
{
_repository = repository;
}
public void Accept(IArticleVisitor visitor, int articleId)
{
CSharpArticle article = _repository.GetArticleById(articleId);
visitor.Visit(article);
_repository.UpdateArticle(article);
}
public void Publish(int articleId)
{
CSharpArticle article = _repository.GetArticleById(articleId);
article.Publish();
_repository.UpdateArticle(article);
}
public void Archive(int articleId)
{
CSharpArticle article = _repository.GetArticleById(articleId);
article.Archive();
_repository.UpdateArticle(article);
}
}
Presentation Layer (Controller)
Controller:
[Route("api/articles")]
[ApiController]
public class CSharpArticleController : ControllerBase
{
private readonly ArticleService _articleService;
public CSharpArticleController(ArticleService articleService)
{
_articleService = articleService;
}
[HttpGet("{id}")]
public IActionResult Get(int id)
{
var article = _articleService.GetArticle(id);
if (article == null)
{
return NotFound();
}
return Ok(article);
}
[HttpPost]
public IActionResult Post([FromBody] CSharpArticle article)
{
if (article == null)
{
return BadRequest();
}
_articleService.CreateArticle(article);
return CreatedAtAction("Get", new { id = article.Id }, article);
}
[HttpPut("{id}")]
public IActionResult Put(int id, [FromBody] CSharpArticle article)
{
if (id != article.Id)
{
return BadRequest();
}
_articleService.UpdateArticle(article);
return NoContent();
}
[HttpDelete("{id}")]
public IActionResult Delete(int id)
{
var existingArticle = _articleService.GetArticle(id);
if (existingArticle == null)
{
return NotFound();
}
_articleService.DeleteArticle(id);
return NoContent();
}
}
Conclusion
This structure separates concerns and enables the Visitor Pattern to conduct actions on the Article model across levels, maintaining logical separation and reusability. The repository, service, and controller implementations may alter depending on the database, ORM, or particular requirements of your application. While the Visitor Pattern can be useful in some situations, it may not be required for every CRUD action. Always evaluate the pattern's true need in the context of your application's complexity and requirements.
The implementation of the Visitor Pattern for C# Article management in an ASP.NET Core Web API following a 3-tier design provides a strong structure for managing CRUD activities. The CSharpArticle model is at the heart of data operations, which are governed by the Data Access Layer via the ArticleRepository. This repository communicates with the database and supports CRUD operations. The Business Layer, represented by the ArticleService, orchestrates operations on articles by utilizing the Visitor Pattern to perform specified tasks via visitors such as content analysis, publishing, archiving, and other appropriate actions.The Presentation Layer's CSharpArticleController serves as the interface for external interactions. It interfaces with the ArticleService to handle HTTP requests, allowing it to get, create, update, and delete articles. Each method correlates to an HTTP verb, allowing for smooth communication with the underlying layers and, ultimately, efficient and controlled article administration.
This hierarchical architecture enables code that is modular, scalable, and maintainable. It allows the program to handle additional features or changes without interfering with core functionality. The application of the Visitor Pattern within the three-tier architecture increases the system's flexibility by fostering a clear separation of responsibilities and improving the API's overall performance and maintainability.