I'm building a tutorial (hopefully soon to be a post) and in that tutorial I needed to use Session for some quick-and-dirty data storage. Unfortunately when I tried to use Session in my default project, it was nowhere to be found, and I was sent down a small rabbit hole trying to find it. This post will walk through a reminder of what Session is, where to find it in ASP.NET Core 1.0, an overview of the new extension methods available, and building our own custom extension method. Let's get started!
What is Session?
If you're just starting to develop in ASP.NET, you may not have encountered Session before. Session is a serialized collection of objects that are related to the current user's session. The values are usually stored on the local server memory, but there are alternate architectures where the values can be stored in a SQL database or other distributed storage solutions, especially when your servers are part of a server farm.
You can store any data you like in Session, however any data you store will only be available to the current user as long as the session is active. This means that if that user logs out, the Session data is lost; if you need to keep this data you have to find another way to store it.
Finding the Session
ASP.NET Core 1.0 has been written from the ground up to be a modular, choose-what-you-need framework. What this means is that you must explicitly include any packages you want to use in your project.
This allows us developers to maintain tight control over what functionality our ASP.NET Core projects actually need, and exclude anything that is not necessary.
In our case, Session is considered to be one of these "additional" packages. In order to include that package we need to add a reference to Microsoft.AspNet.Session in the project.json file. If we wanted to use memory as our caching backend, we would also include Microsoft.Extensions.Caching.Memory.
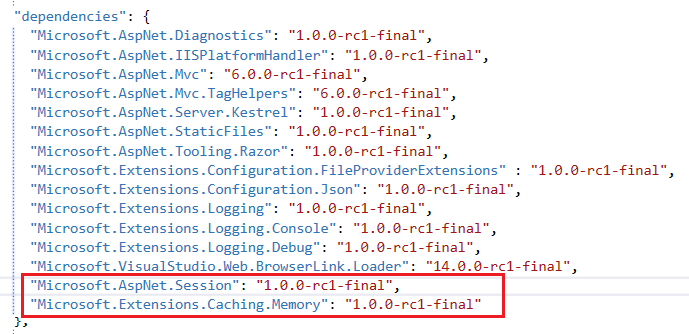
Once we've got the package included in our project, we need to make it available to the Services layer by modifying the ConfigureServices()method in the Startup file, like so:
public void ConfigureServices(IServiceCollection services)
{
...
services.AddMemoryCache();
services.AddSession(options =>
{
options.IdleTimeout = TimeSpan.FromMinutes(60);
options.CookieName = ".MyCoreApp";
});
...
}
With all of these steps completed, you can now use Session in your projects just like in any other ASP.NET application. If you wanted to use a different cache backend (rather than memory) you could grab a different NuGet package like Redis or SqlServer. Don't forget to check NuGet if you can't find the functionality you need; it is probably there and you just need to download it.
How to Use Session
ASP.NET Core 1.0 has introduced some new extension methods that we can use for accessing and storing Session values. The odd thing is that these extensions are not in Microsoft.AspNet.Session; rather, they are in Microsoft.AspNet.Http, and so we will need to add that package.
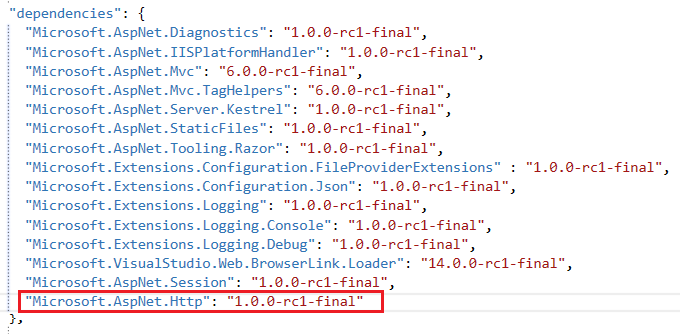
Once we've got that package included, we can start using the extension methods:
[HttpGet]
public IActionResult Index()
{
var userID = Context.Session.GetInt("UserID");
var userName = Context.Session.GetString("UserName");
return View();
}
[HttpGet]
public IActionResult Default()
{
Context.Session.SetInt("UserID", 5);
Context.Session.SetString("UserName", "John Smith");
return View();
}
The new extension methods are:
- Get: Returns a byte array for the specified Session object.
- GetInt: Returns an integer value for the specified Session object.
- GetString: Returns a string value for the specified Session object.
- Set: Sets a byte array for the specified Session object.
- SetInt: Sets an integer value for the specified Session object.
- SetString: Sets a string value for the specified Session object.
Why do only these extensions exist, and not GetDouble, GetDateTime, etc? I'm really not sure. If I had to guess I'd say it is to ensure that the values are serializable, but don't quote me on that. If anybody knows the real reason, I'd love to hear it!
Creating Extension Methods
I'm not completely satisfied with these extensions; they don't have enough functionality for my tastes, and so I'm gonna build some more. Specifically, I want to build extensions that will store a DateTime in session and retrieve it.
Here's the method signatures for these extensions:
public static DateTime? GetDateTime(this ISessionCollection collection, string key)
{
}
public static void SetDateTime(this ISessionCollection collection, string key, DateTime value)
{
}
The ISessionCollection interface is exactly what it sounds like: a collection of items stored in Session.
Let's tackle the SetDateTime() method first. DateTimes are weird because they are not inherently serializable, but they can be converted to a serializable type: long. So, we must convert the given DateTime value to a long before it can be stored.
public static void SetDateTime(this ISessionCollection collection, string key, DateTime value)
{
collection.Set(key, BitConverter.GetBytes(value.Ticks));
}
The BitConverter class allows us to convert byte arrays into other types easily.
Now we can tackle the GetDateTime() method. There are two things we need to keep in mind when building this extension. First, it is entirely possible that there will be no value in Session for the specified key; if this happens, we should return null. Second, we are storing the DateTime as a long, and therefore we need to serialize it back into a DateTime type; luckily the DateTime constructor makes this really easy. The final code for the method looks like this:
public static DateTime? GetDateTime(this ISessionCollection collection, string key)
{
var data = collection.Get(key);
if(data == null)
{
return null;
}
long dateInt = BitConverter.ToInt64(data, 0);
return new DateTime(dateInt);
}
Now we can use these extensions in addition to the ones already defined.
Now we've seen Session in action, including what package to use from NuGet, what extension methods are available, and even how to build our own extension method. Let me know if this helped you out in the comments!
Happy Coding!
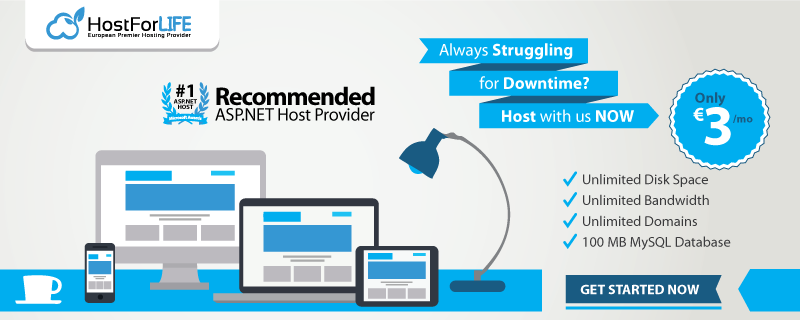