European leading web hosting provider, HostForLIFE.eu announces the launch of ASP.NET 5 support on the recently released Windows Server 2012 R2.
HostForLIFE.eu was established to cater to an under served market in the hosting industry; web hosting for customers who want excellent service. HostForLIFE.eu – a cheap, constant uptime, excellent customer service, quality, and also reliable hosting provider in advanced Windows and ASP.NET technology. HostForLIFE.eu proudly announces the availability of the ASP.NET 5 hosting in our entire servers environment.
ASP.NET is Microsoft's dynamic website technology, enabling developers to create data-driven websites using the .NET platform and the latest version is 5 with lots of awesome features. ASP.NET 5 is a lean .NET stack for building modern web apps. Microsoft built it from the ground up to provide an optimized development framework for apps that are either deployed to the cloud or run on-premises. It consists of modular components with minimal overhead.
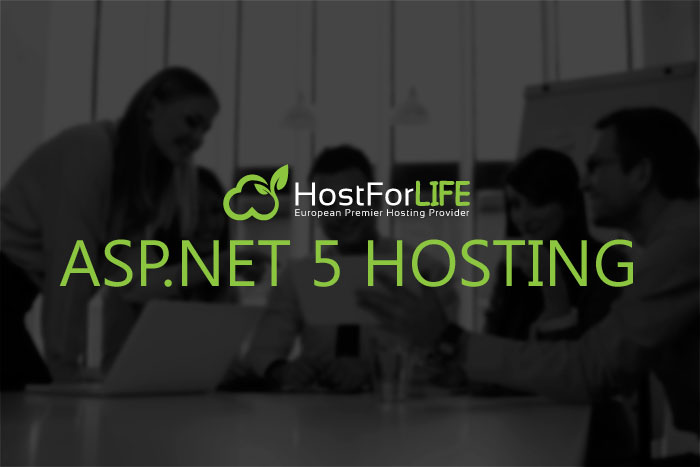
According to Microsoft officials, much of the functionality in the ASP.NET 5 release is a new flexible and cross-platform runtime, a new modular HTTP request pipeline, Cloud-ready environment configuration, Unified programming model that combines MVC, Web API, and Web Pages, Ability to see changes without re-building the project, etc.
HostForLIFE.eu hosts its servers in top class data centers that is located in Amsterdam (NL), London (UK), Paris (FR) and Seattle (US) to guarantee 99.9% network uptime. All data center feature redundancies in network connectivity, power, HVAC, security, and fire suppression. All hosting plans from HostForLIFE.eu include 24×7 support and 30 days money back guarantee. The customers can start hosting our ASP.NET 5 site on our environment from as just low €3.00/month only.
HostForLIFE.eu is a popular online ASP.NET based hosting service provider catering to those people who face such issues. The company has managed to build a strong client base in a very short period of time. It is known for offering ultra-fast, fully-managed and secured services in the competitive market.
HostForLIFE.eu offers the latest European ASP.NET 5 hosting installation to all our new and existing customers. The customers can simply deploy our ASP.NET 5 website via our world-class Control Panel or conventional FTP tool. HostForLIFE.eu is happy to be offering the most up to date Microsoft services and always had a great appreciation for the products that Microsoft offers.
Further information and the full range of features ASP.NET 5 Hosting can be viewed here http://hostforlife.eu/European-ASPNET-5-Hosting
About Company
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. HostForLIFE.eu deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
HostForLIFE.eu is awarded Top No#1 SPOTLIGHT Recommended Hosting Partner by Microsoft (see http://www.asp.net/hosting/hostingprovider/details/953). Their service is ranked the highest top #1 spot in several European countries, such as: Germany, Italy, Netherlands, France, Belgium, United Kingdom, Sweden, Finland, Switzerland and other European countries. Besides this award, we have also won several awards from reputable organizations in the hosting industry and the detail can be found on our official website.