
December 15, 2014 06:01 by
Peter
Today, I will show you how to ValidationSummary Control in ASP.NET 5. ValidationSummary control allow us to display summary of all validation errors. We can display validation errors summary inline of a web page or a message box or both by using ShowMessageBox and ShowSummary property value true or false.
ValidationSummary will display validation messages as bulleted list, 1 paragraph or only list based on DisplayMode. we can set a header text for validation summar. ASP.NET validationsummary control have many properties to design the error messages text as like fore color, back color, border color, border style, border width, theme, skin and after all CSS class.
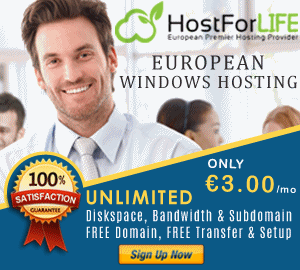
This ValidationSummary, allow to summarize of all validation error messages from all validators in a single location. This code below will show you how can we display all validation error messages as summary using ValidationSummary control. In this example, I used three text box and make them required field using requiredfieldvalidator control. When user submit form without entering textboxes value, they got a validation error messages summary in one location as bulleted list.
And here is the example ValidationSummaryExample.aspx code:
<%@ Page Language="C#" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e) {
Label1.Text = "Your Country: " +
TextBox1.Text.ToString() +
"<br />Region: " +
TextBox2.Text.ToString() +
"<br />City: " +
TextBox3.Text.ToString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>ValidationSummary example: how to use ValidationSummary control in asp.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server" Font-Size="Large" ForeColor="BlueViolet"></asp:Label>
<br /><br />
<asp:Label ID="Label2" runat="server" Text="Country Name" AssociatedControlID="TextBox1"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator
ID="RequiredFieldValidator1"
runat="server"
ControlToValidate="TextBox1"
ErrorMessage='Input Country!'
EnableClientScript="true"
SetFocusOnError="true"
Text="*"
>
</asp:RequiredFieldValidator>
<br />
<asp:Label ID="Label3" runat="server" Text="Region Name" AssociatedControlID="TextBox2"></asp:Label>
<asp:TextBox ID="TextBox2" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator
ID="RequiredFieldValidator2"
runat="server"
ControlToValidate="TextBox2"
ErrorMessage='Input Region!'
EnableClientScript="true"
SetFocusOnError="true"
Text="*"
>
</asp:RequiredFieldValidator>
<br />
<asp:Label ID="Label4" runat="server" Text="City Name" AssociatedControlID="TextBox3"></asp:Label>
<asp:TextBox ID="TextBox3" runat="server"></asp:TextBox>
<asp:RequiredFieldValidator
ID="RequiredFieldValidator3"
runat="server"
ControlToValidate="TextBox3"
ErrorMessage='Input Region!'
EnableClientScript="true"
SetFocusOnError="true"
Text="*"
>
</asp:RequiredFieldValidator>
<br />
<asp:Button ID="Button1" runat="server" Text="Submit" OnClick="Button1_Click" />
<br /><br />
<asp:ValidationSummary ID="ValidationSummary1" runat="server" HeaderText="Following error occurs:" ShowMessageBox="false" DisplayMode="BulletList" ShowSummary="true" />
</div>
</form>
</body>
</html>