What is Authentication in the.NET Core API?
Authentication is one of the most fundamental aspects of software development. It secures our APIs and refuses API requests if an unauthorized user attempts to access secure endpoints.
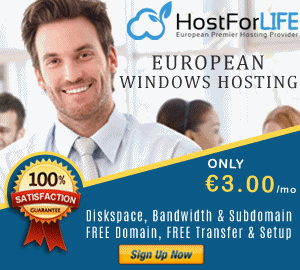
Why do we need Authentication in .Net Core API?
- Data Protection: APIs frequently expose access to data or services that could be abused if they fall into the wrong hands. Authentication ensures that only authorized people or systems can access this information or service.
- Access Control: Not all users should have equal access rights. Some users may have complete access, while others may have limited permissions. Authentication is the initial step in building such an access control mechanism.
- Non-repudiation: Using proper authentication, actions may be traced back to the user who conducted them. This ensures accountability for activities taken, which is necessary in many applications.
- Compliance: Many sectors have standards and obligations in place to protect data and privacy. Authentication, together with other security procedures, helps to meet these regulatory compliance requirements.
- User Experience: By authenticating users, APIs can also provide personalized experiences, as they know who is making the request and can tailor responses accordingly.
Let's Begin
Let's create a new project
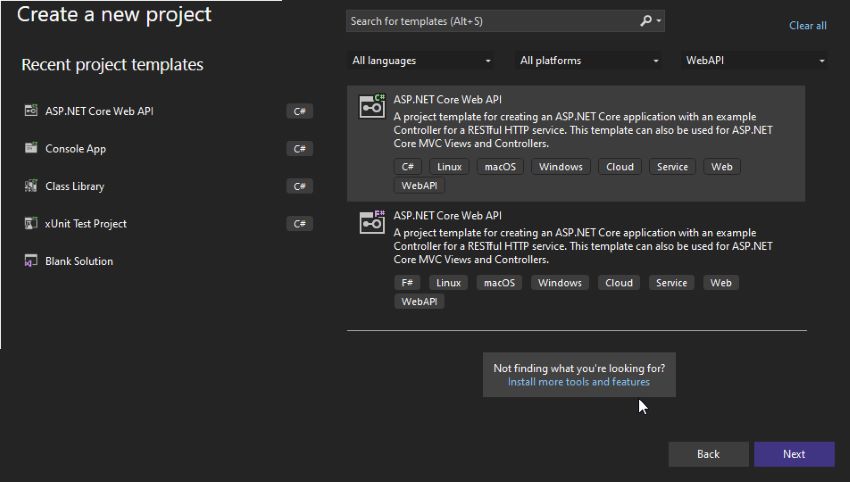
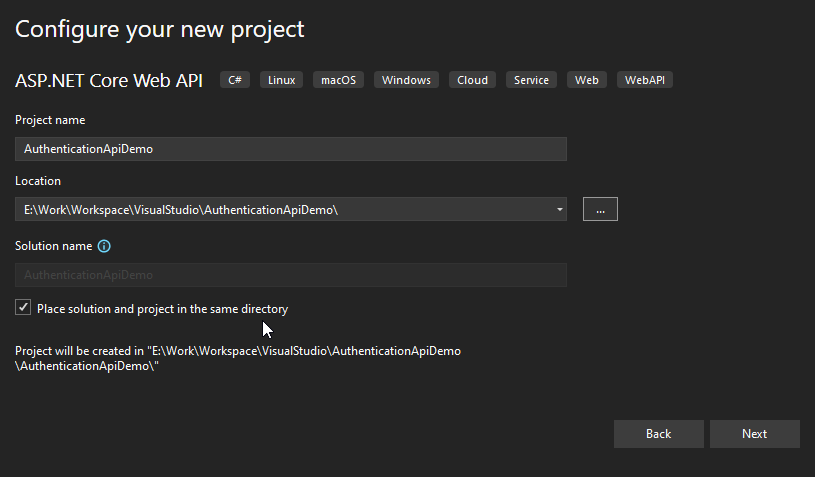
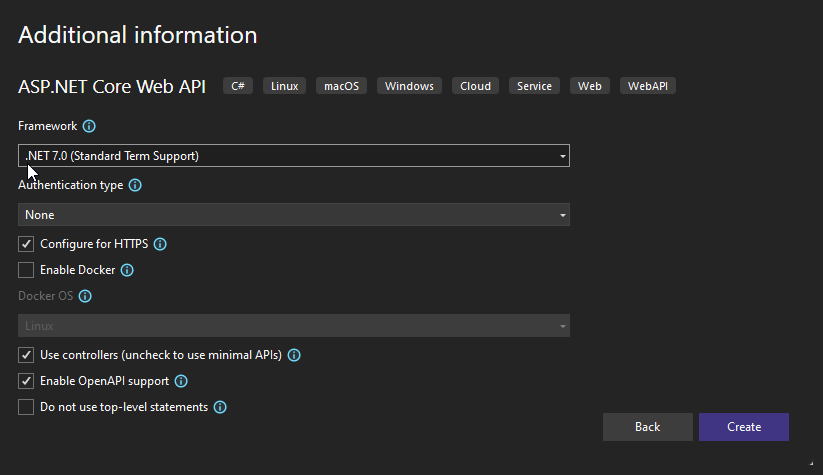
Following the API construction, we will install the nuget package "Microsoft.AspNetCore.Authentication.JwtBearer".
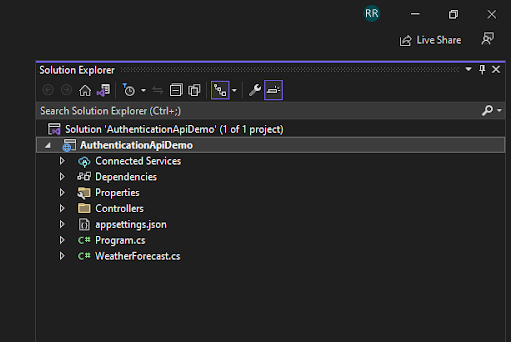
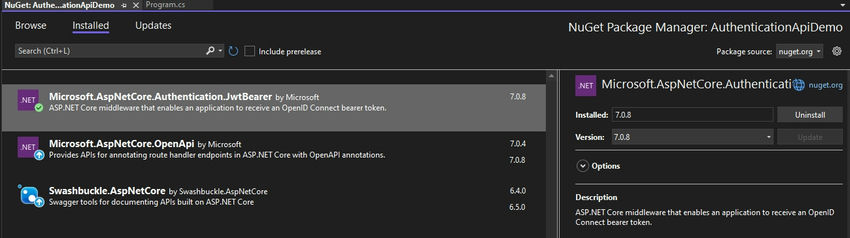
For Authentication, we will be using Okta; Okta provides a way to manage and provide access to users and gives its developer platform to try out authentication stuff.
Sign up on the below link
https://developer.okta.com/signup/
Use authO options, and pick up the region of your choice.
After that, we will create a new API, and In the identifier, we usually give out the hosted app URL, but here for testing purposes. We will give our localhost url of our API.
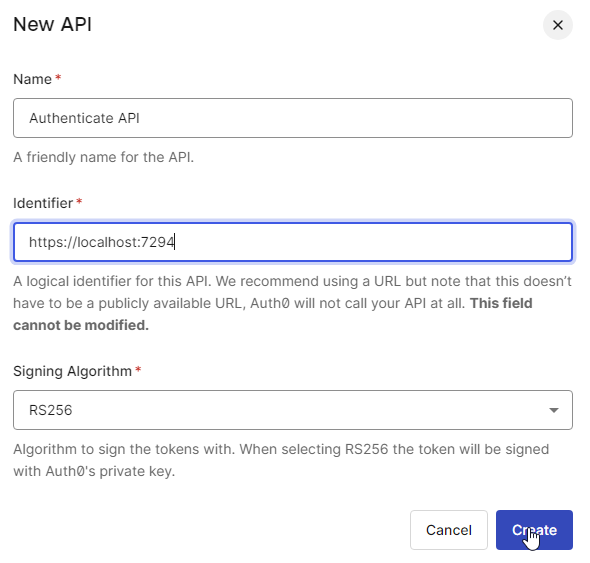
Now click on the APIs on the left side and click on the Test section; you will get the curl command to generate the Okta auth token.
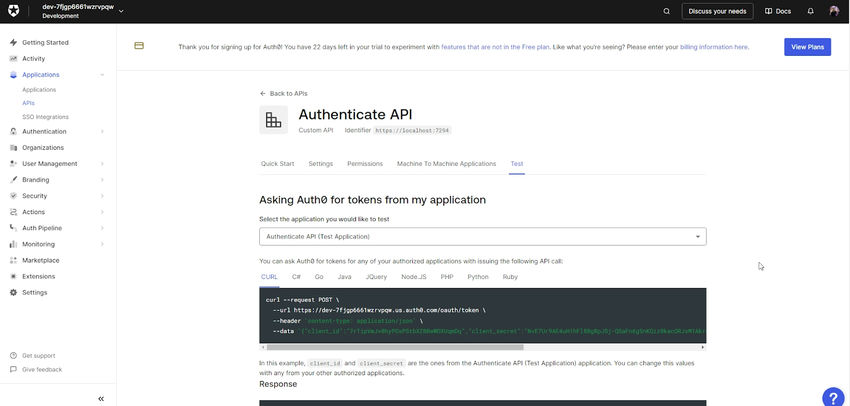
Now let's open our Program.cs, and we will add the following code.
builder.Services.AddAuthentication(options =>
{
options.DefaultAuthenticateScheme = JwtBearerDefaults.AuthenticationScheme;
options.DefaultChallengeScheme = JwtBearerDefaults.AuthenticationScheme;
}).AddJwtBearer(options =>
{
options.Authority = "https://dev-7fjgp6661wzrvpqw.us.auth0.com/";
options.Audience = "https://localhost:7294";
});
From the values in Okta portal, we can use it in the Postman to get the token response.
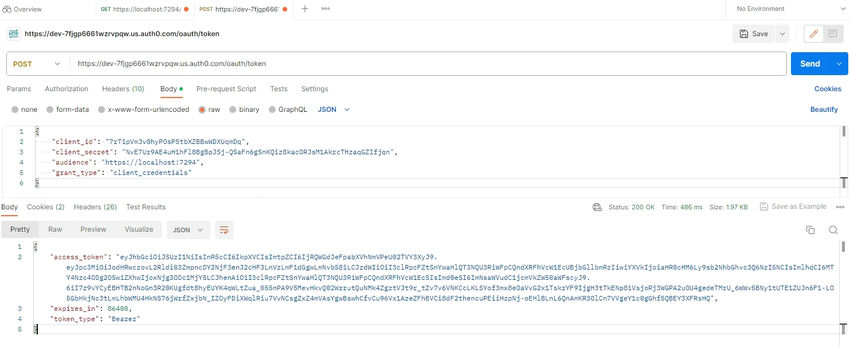
Now we will add a new controller in our API called "AllowAllController".
[Route("api/[controller]")]
[ApiController]
public class AllowAllController: ControllerBase {
[HttpGet]
public string Get() {
return "Working okay";
}
}
Once we run the API and hit this endpoint, we will get the response.
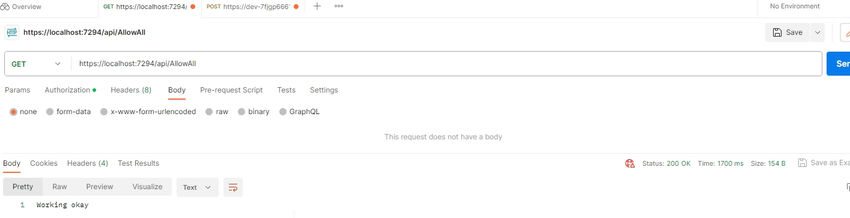
Now to test authorization, we will add a [Authorize] keyboard at the top of the controller.
So now our modified controller looks like this.
[Route("api/[controller]")]
[ApiController]
[Authorize]
public class AllowAllController: ControllerBase
{
[HttpGet]
public string Get()
{
return "Working okay";
}
}
Now when we hit our API, it will get the Unauthorized response.
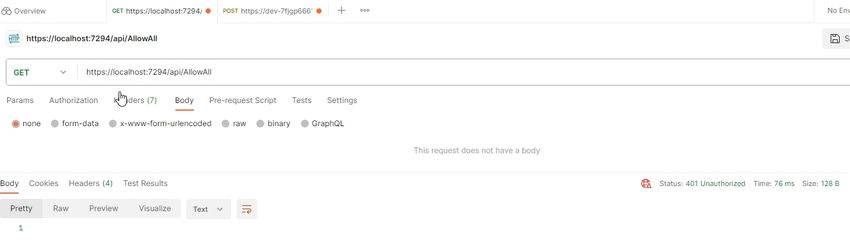
Now we will pass the token we get from the token endpoint in the Authorization Header of the request.
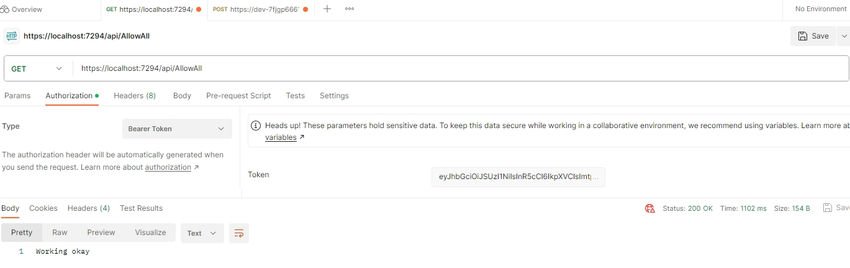