
February 20, 2024 08:29 by
Peter
Prompt engineering
For conversational AI systems to be effective, prompt engineering is essential. We'll examine how to apply rapid engineering with.NET Core, a flexible framework for creating cross-platform apps, in this practical course. In order to demonstrate several prompt engineering strategies, such as contextual prompts, error management, and response variation, we'll develop a basic chatbot application.
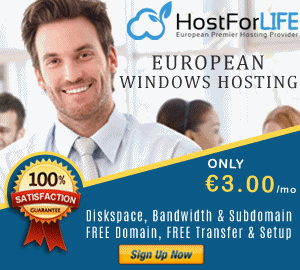
Setting up your development environment
Before we begin, ensure you have the .NET Core SDK installed on your system. You can download it from the official .NET website
Once installed, open your terminal or command prompt and run the following command to verify the installation.
dotnet --version
If the installation was successful, you should see the version of .NET Core.
Creating a new .NET core console application
Let's start by creating a new .NET Core console application. Open your terminal or command prompt and navigate to the directory where you want to create the project. Then, run the following command.
dotnet new console -n ChatbotApp
This command creates a new console application named "ChatbotApp."
Building the Chatbot
Now that we have our project set up, let's build the chatbot functionality. Open the Program.cs file in your preferred code editor and replace the existing code with the following.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to the Chatbot!");
Console.WriteLine("What's your name?");
string userName = Console.ReadLine();
Console.WriteLine($"Hello, {userName}! How can I assist you today?"); // Your prompt engineering logic goes here
}
}
This code prompts the user for their name and greets them accordingly. Let's add some prompt engineering logic to provide a more interactive experience.
Implementing prompt engineering
- Contextual Prompts: Based on the user's input, we can tailor the prompts to provide relevant responses. For example, if the user asks for help, we can provide assistance prompts.
- Error Handling: Handle user input errors gracefully. If the user provides invalid input, prompt them to try again.
- Response Variation: Introduce a variety of responses to make the conversation feel more natural and engaging.
Here's an example of how you can enhance the chatbot with these prompt engineering techniques.
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Welcome to the Chatbot!");
Console.WriteLine("What's your name?");
string userName = Console.ReadLine();
Console.WriteLine($"Hello, {userName}! How can I assist you today?");
// Continuously prompt the user for input
while (true)
{
string userInput = Console.ReadLine().ToLower();
// Handle specific user inputs
if (userInput.Contains("help"))
{
Console.WriteLine("Sure, I can help you with that!");
continue;
}
else if (userInput.Contains("quit") || userInput.Contains("exit"))
{
Console.WriteLine("Goodbye! Have a great day!");
break;
}
// Default response for other inputs
Console.WriteLine("I'm sorry, I didn't understand. Can you please rephrase?");
}
}
}
Running the Chatbot
To run the chatbot, navigate to the project directory in your terminal or command prompt and execute the following command.
dotnet run
You'll see the chatbot welcome message and prompt for the user's name. Then, you can interact with the chatbot by entering different inputs and observing the prompt engineering logic in action.
In this hands-on tutorial, we explored prompt engineering using .NET Core by building a simple chatbot application. We implemented various prompt engineering techniques, including contextual prompts, error handling, and response variation, to create an engaging conversational experience. By mastering prompt engineering with .NET Core, you can develop conversational interfaces that provide seamless interactions and enhance user engagement.
Start experimenting with prompt engineering in your .NET Core projects today and unleash the full potential of conversational AI systems.