
July 18, 2018 09:09 by
Peter
In this article, we are going to learn how to cancel or interrupt the Long Running Task using the Cancellationtokensource method in ASP.NET Core. So, here is the task: My web application will send POST request by making web service API call and get the data via a third-party library. For example, consider, the application is going to send details about the students through the API and will get the response once the POST data is successfully received and saved on another side. Consider that the application is going to send 5000 bits of data through the API. So, by simply using for-each loop by calling third-party API, we can POST 5000 data.
It will take too long to finish this job. Until this job is done the application will not allow other operations to perform as the job is running in the main thread. To make it possible, we will move to “TASK”, to run this job in a separate thread so that the application can do other operations. To understand this, have a look at the code below.
Var task=Task.Factory.StartNew(()=> CallWebServiceandGetResponceData());
The code is creating the task which is making API webservice call inside CallWebServiceandGetResponceData function to send a request and get the response data. So by doing this, the application allows this task operation in a separate thread and we can do other operations in the main thread, which will not affect the task operation. Everything is going fine.
If you are using .NET 4.5 Framework you can use Async and wait for keywords to do this. But in .NET 4.0 we can’t use async and await keywords, so I'm going to use “TASK” to achieve this operation. So, now I need to cancel or stop the task whenever I need to. For example, 2673rd data is currently running inside the CallWebServiceandGetResponceData function, when I hit the stop button I need to cancel or stop calling third-party API and exit the loop. To do this I'm going to use CancellationToken.
.NET 4.0 introduces a lightweight struct called the cancellationToken that provide a mechanism to cancel synchronous and asynchronous operations. CancellationToken consist is a CancellationRequested method, it is a Boolean property that can be polled by code to find out if it has been canceled or the cancellation has been requested.
By using instance of CancellationTokenSource class to get a cancellation token, in cancellation model the cancellation token can be passed freely to the listeners, so that canceling token is cleanly separted from the ability to consume a request for cancellation. Here is the sample code to cancel a task,
CancellationTokenSource mCancellationTokenSource = new CancellationTokenSource();
CancellationToken token = mCancellationTokenSource.Token;
Task < string > ObjTask = Task.Factory.StartNew(() => {
while (!token.IsCancellationRequested) {
try {
For(i = 0; i < totalrecordscount; i++) {
If(i == 2341) {
Token.Cancecl();
}
// Sending data to other end by api call
}
} catch () {
return;
}
}
return;
}, token);
The above code will run continuously in while loop until it gets the cancel request. Once the token gets a cancellation request the control will terminate the entire Task and the control will get back to the main thread. Here, the cancel request is made inside the task based on the condition.
Instead we can also stop the task whenever we want, like after the task started execution, when I hit the stop button from front end the token get cancellation request and terminate the execution. Here, is the sample code to cancel a task by clicking stop button:
Bool istasktostop = false; // declare one global bool variable
CancellationTokenSource mCancellationTokenSource = new CancellationTokenSource();
CancellationToken token = mCancellationTokenSource.Token;
Task < string > ObjTask = Task.Factory.StartNew(() => {
while (!token.IsCancellationRequested) {
try {
For(i = 0; i < totalrecordscount; i++) {
//for each loop its checking weather “istasktostop” is
If(istasktostop) {
Token.Cancecl();
}
// Sending data to other end by api call
}
} catch () {
return;
}
}
return;
}, token);
protected void Stop_Sending_Click(object sender, ImageClickEventArgs e) {
istasktostop = true;
}
Normally, if we did the same operation without task and a cancellationtoken, we can't send stop request when the process is in running mode because the current process occupies the main thread and we can't interrupt the main thread while it's running. When we did the same process inside the task, it is separated and run at a separate thread, so we can do other processes in the main thread.
HostForLIFE.eu ASP.NET Core 2.2.1 Hosting
European best, cheap and reliable ASP.NET hosting with instant activation. HostForLIFE.eu is #1 Recommended Windows and ASP.NET hosting in European Continent. With 99.99% Uptime Guaranteed of Relibility, Stability and Performace. HostForLIFE.eu security team is constantly monitoring the entire network for unusual behaviour. We deliver hosting solution including Shared hosting, Cloud hosting, Reseller hosting, Dedicated Servers, and IT as Service for companies of all size.
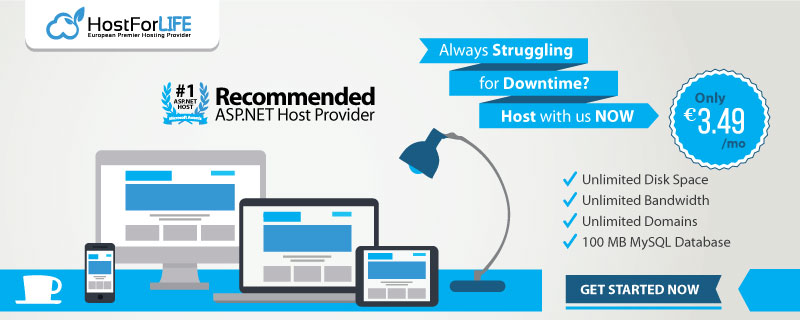