Today, let me show you how to select data tree view in ASP.NET. The ASP.NET TreeView control is a powerful server-control for rendering TreeView UI, as shown in the figure below. It supports a variety of programming models, from statically-defined trees, to dynamically constructed trees, to databound trees. The TreeView's rendering is fully customizable, allowing for a wide-range of look-and-feels for the control. The TreeView supports both postback-style events and simple hyperlink navigation, as well as a unique event handling model that allows data to be retrieved directly from a client without requiring a server postback. It also supports rendering on a variety of browsers, and can take advantage of up-level capabilities, such as client-script on later desktop browser versions.
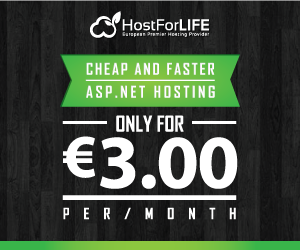
Now, it's time to write the following code:
public void fill()
{
con.Open();
SqlCommand cmd = new SqlCommand("select sr_no,rank_no,emp_name,emp_code,rank_name,doj from empregistration where emp_code='" + TextBox4.Text + "' ", con);
SqlDataReader dr = cmd.ExecuteReader();
if (dr.Read())
{
no = Convert.ToInt32(dr["rank_no"].ToString());
Label20.Text = dr["emp_name"].ToString();
Label21.Text = dr["emp_code"].ToString();
Label22.Text = dr["rank_name"].ToString();
Label23.Text = dr["doj"].ToString();
no13 = Convert.ToInt32(dr["sr_no"].ToString());
dr.Read();
} else
{
Response.Write("<script>alert('Employee Code Is Not Correct')</script>");
}
for (int i = no; i >= 1; i--)
{
if (i == 12)
{
no12 = Convert.ToInt32(i.ToString());
}
if (i == 11)
{
no11 = Convert.ToInt32(i.ToString());
}
if (i == 10)
{
no10 = Convert.ToInt32(i.ToString());
}
if (i == 9)
{
no9 = Convert.ToInt32(i.ToString());
}
if (i == 8)
{
no8 = Convert.ToInt32(i.ToString());
}
if (i == 7)
{
no7 = Convert.ToInt32(i.ToString());
}
if (i == 6)
{
no6 = Convert.ToInt32(i.ToString());
}
if (i == 5)
{
no5 = Convert.ToInt32(i.ToString());
}
if (i == 4)
{
no4 = Convert.ToInt32(i.ToString());
}
if (i == 3)
{
no3 = Convert.ToInt32(i.ToString());
}
if (i == 2)
{
no2 = Convert.ToInt32(i.ToString());
}
if (i == 1)
{
no1 = Convert.ToInt32(i.ToString());
}
}
con.Close();
// SqlCommand cmd1 = new SqlCommand("select rank_no from empregistration where ", con);
}
protected void Button1_Click(object sender, EventArgs e)
{
Label14.Visible = true;
Label8.Visible = true;
Label15.Visible = false;
Label7.Visible = false;
fill();
con.Open();
SqlCommand cmd = new SqlCommand("select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no12 + "'and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no11 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no10 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no9 + "'and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no8 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no7 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no6 + "'and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no5 + "'and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no4 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no3 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no2 + "' and sr_no >'" + no13.ToString() + "' union select rank_no,rank_name,emp_code,emp_name,doj,int_code,int_name,int_rank from empregistration where rank_no <='" + no1 + "' and sr_no >'" + no13.ToString() + "' order by emp_code asc ", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
DataSet ds = new DataSet();
da.Fill(ds);
if (ds.Tables[0].Rows.Count > 0)
{
gvProducts.DataSource = ds;
gvProducts.DataBind();
}
con.Close();
}
I hope this code is work for you. Good luck!
HostForLIFE.eu ASP.NET Core 1.0 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
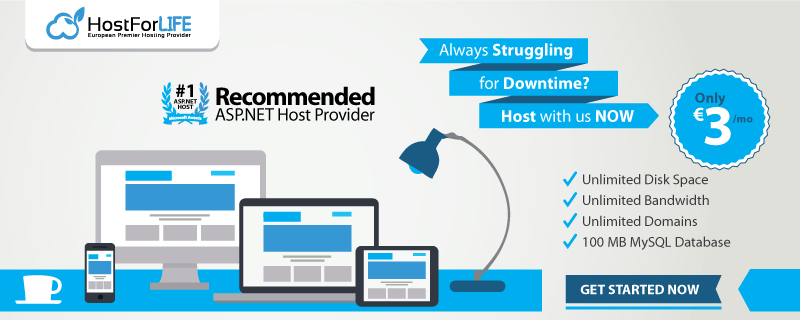