
November 28, 2023 06:29 by
Peter
DateTime Fundamentals in C#
Before we go into formatting, let's go over the fundamentals of working with DateTime in C#. The DateTime structure represents dates and times and provides methods for modifying and comparing dates. DateTime can be used to get the current date and time.DateTime can now be used to denote a specific date.DateTime or Parse.ParseExact.
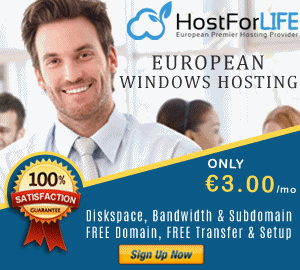
DateTime Object Formatting
When it comes to turning DateTime objects into human-readable strings, the ToString method is the major player. To produce the desired output, you can use standard format specifiers or custom format strings.
Specifiers of Standard Format
You can use the ToString function with a set of standard format specifiers provided by C#.
C# provides a set of standard format specifiers that you can use with the ToString method.
"d": Short date pattern
"t": Short time pattern
"f": Full date/time pattern (short time)
"g": General date/time pattern (short time)
"s": Sortable date/time pattern (ISO 8601)
"u": Universal sortable date/time pattern (UTC)
DateTime now = DateTime.Now; string shortDate = now.ToString("d"); string fullDateTime = now.ToString("f");
Custom Format Strings
For more control over the formatting, you can use custom format strings.
"yyyy": Four-digit year
"MM": Two-digit month
"dd": Two-digit day
"HH": Two-digit hour (24-hour clock)
"mm": Two-digit minute
"ss": Two-digit second
DateTime now = DateTime.Now; string customFormat = now.ToString("yyyy-MM-dd HH:mm:ss");
Handling Time Zones
Dealing with time zones is a common challenge in programming. You can use the DateTime methods ToUniversalTime and ToLocalTime to convert between UTC and local time.
DateTime utcNow = DateTime.UtcNow; DateTime localTime = utcNow.ToLocalTime();
Example 1. Using Standard Format Specifiers
using System;
class Program
{
static void Main()
{
DateTime now = DateTime.Now;
// Short date pattern
string shortDate = now.ToString("d");
Console.WriteLine("Short Date: " + shortDate);
// Full date/time pattern (short time)
string fullDateTime = now.ToString("f");
Console.WriteLine("Full Date/Time: " + fullDateTime);
// Sortable date/time pattern (ISO 8601)
string sortableDateTime = now.ToString("s");
Console.WriteLine("Sortable Date/Time: " + sortableDateTime);
}
}
Output
Short Date: 11/25/2023 Full Date/Time: Sunday, November 25, 2023 3:23 PM Sortable Date/Time: 2023-11-25T15:23:45
Example 2. Using Custom Format Strings
using System;
class Program
{
static void Main()
{
DateTime now = DateTime.Now;
// Custom format: yyyy-MM-dd HH:mm:ss
string customFormat = now.ToString("yyyy-MM-dd HH:mm:ss");
Console.WriteLine("Custom Format: " + customFormat);
// Custom format: ddd, MMM dd yyyy
string customFormat2 = now.ToString("ddd, MMM dd yyyy");
Console.WriteLine("Custom Format 2: " + customFormat2);
}
}
Output
Custom Format: 2023-11-25 15:23:45
Custom Format 2: Sun, Nov 25 2023
Experiment with various format strings and tailor them to your specific needs and tastes. Customizing the format enables you to display dates and times in the manner that is most appropriate for your application or user interface.
Conclusion
Date and time formatting in C# is an essential skill for any developer. Understanding the subtleties of DateTime formatting is critical whether you're presenting dates in a user interface, logging events, or dealing with time-sensitive data. Experiment with alternative format specifiers, develop custom formats, and take time zone differences into account to guarantee your apps handle dates and times correctly and logically. You're well-equipped to tackle the problems of managing temporal data in C# with these skills in your toolbox. Have fun coding!