
December 11, 2023 06:52 by
Peter
A more complete and reliable substitute for the built-in DateTime and DateTimeOffset classes in the.NET Framework is the date and time management library NodaTime. It is intended to handle typical problems and intricacies related to changing dates and times.
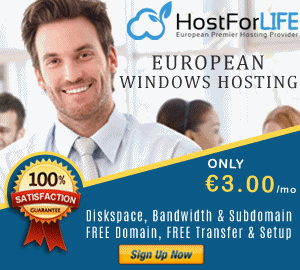
Important aspects of NodaTime
- Immutable Types: NodaTime represents dates, times, and durations using immutable types. This encourages the development of a more consistent and thread-safe programming model and aids in the prevention of accidental object change.
- Rich number of Types: NodaTime offers a rich number of types, such as ZonedDateTime (a date and time in a particular time zone), LocalDate (a date without a time), Instant (a point on the timeline), and LocalTime (a time without a date).
- Time Zones: NodaTime provides extensive time zone support, enabling developers to operate across time zones, carry out conversions, and manage changes in daylight saving time.
- Duration and Period: To handle time spans and calendar discrepancies, respectively, more expressively and precisely, NodaTime provides the Duration and Period types.
- Compatibility with.NET Platforms: NodaTime is made to function with the.NET Framework,.NET Core, and.NET 5/6, among other.NET platforms.
- Improved Thread Safety: Reasoning about concurrent programs requiring date and time operations is made easier by the usage of immutable types.
- NodaTime's extensibility enables developers to specify their own calendar systems and, if necessary, provide more time zone data.
- Testing Support: NodaTime comes with features to make testing easier, like a FakeClock to manage time in unit tests.
NodaTime was developed to provide a more reliable and developer-friendly method of handling date and time in.NET programs by addressing some of the shortcomings and difficulties related to the DateTime and DateTimeOffset classes. When handling situations where exact control over time and time zones is necessary, it is quite helpful.
Noda Time aims for.NET Standard 1.3 and.NET 4.5. We don't utilize dynamic typing in the distributable libraries for optimal compatibility, although we do use it periodically in testing. Noda Time users do not require a current C# compiler, but we usually make advantage of language features as soon as they are made available in stable beta and general release. Although we make every effort to avoid adding external dependencies, using C# 7 tuples is currently not possible since System.ValueTuple would add another dependence.
How Do I Begin Using NodaTime?
1) Use the package manager console to perform the following command or install the NodaTime package/library from the Manage NuGet package. This is NodaTime's core library.
Install-Package NodaTime
2) Install the NodaTime Serialization package/library as below in the package manager console. This library is useful when serializing the NodaTime type.
Install-Package NodaTime.Serialization.JsonNet
3) Install the NodaTime Testing library for building the Unit test project.
Install-Package NodaTime.Testing
NodaTime properties
Instant
In NodaTime, Instant is a fundamental type representing an instantaneous point on the timeline. It is similar to DateTimeOffset in the .NET Framework but provides a more precise representation of time, particularly in scenarios where high precision is required.Install-Package NodaTime
2) Install the NodaTime Serialization package/library as below in the package manager console. This library is useful when serializing the NodaTime type.
Install-Package NodaTime.Serialization.JsonNet
3) Install the NodaTime Testing library for building the Unit test project.
Install-Package NodaTime.Testing
NodaTime properties
Instant
In NodaTime, Instant is a fundamental type representing an instantaneous point on the timeline. It is similar to DateTimeOffset in the .NET Framework but provides a more precise representation of time, particularly in scenarios where high precision is required.
Instant instant = SystemClock.Instance.GetCurrentInstant();
Console.WriteLine($"NodaTime Instant : {instant}");
Output. NodaTime Instant: 2023-12-08T05:22:05Z
Converting Instant type into UTC time.
Instant convertedToUtc = instant.InUtc().ToInstant();
Console.WriteLine($"NodaTime in UTC : {convertedToUtc}");
Output. NodaTime in UTC: 2023-12-08T05:22:05Z
Getting Instant type with TimeZone specified.
Instant convertedToEastern = instant.InZone(DateTimeZoneProviders.Tzdb["America/New_York"]).ToInstant();
Console.WriteLine($"NodaTime in Zone : {convertedToEastern}");
Output. NodaTime in Zone: 2023-12-08T05:22:05Z
ZonedDateTime
'ZonedDateTime in NodaTime is a type that represents a specific date and time with an associated time zone. This is particularly useful for handling time-related information in a way that considers different time zones and daylight saving time changes.
ZonedDateTime zonedDateTime = instant.InZone(DateTimeZoneProviders.Tzdb["Europe/Berlin"]); //US/Pacific
Console.WriteLine($"NodaTime ZonedDateTime : {zonedDateTime}");
Output. NodaTime ZonedDateTime : 2023-12-08T06:22:05 Europe/Berlin (+01)
OffsetDateTime
'OffsetDateTime in NodaTime represents a date and time along with an offset from UTC (Coordinated Universal Time). This type is useful when you want to work with an absolute point in time while considering the offset from UTC.
OffsetDateTime offsetDateTime = OffsetDateTime.FromDateTimeOffset(dateTimeOffset);
Console.WriteLine($"NodaTime offsetDateTime : {offsetDateTime}");
Output. NodaTime offsetDateTime : 2023-12-08T10:52:05+05:30
LocalDateTime
'LocalDateTime in NodaTime represents a date and time without any specific time zone or offset from UTC. It's a combination of a LocalDate and a LocalTime. This type is suitable for situations where you want to work with a date and time without considering time zone-related adjustments.
LocalDateTime localDateTime = zonedDateTime.LocalDateTime;
Console.WriteLine($"NodaTime LocalDateTime : {localDateTime}");
Output. NodaTime LocalDateTime : 12/8/2023 6:22:05 AM
LocalDate
'LocalDate in NodaTime represents a date without considering any time zone or offset from UTC. It only consists of the year, month, and day components. This type is suitable for situations where you need to work with dates independently of time zones or daylight-saving time changes.
LocalDate LD = new LocalDate(1992, 05, 08);
Console.WriteLine($"LocalDate : {LD}");
Output. LocalDate : Friday, May 8, 1992
LocalTime
'LocalTime in NodaTime represents a time of day without any association with a specific time zone or offset from UTC. It captures the hour, minute, second, and fractional seconds of a given time, allowing you to work with time-related operations without considering time zone changes.
LocalTime LT = new LocalTime(8, 0, 0);
Console.WriteLine($"LocalTime : {LT}");
Output. LocalTime : 8:00:00 AM
Summary
NodaTime offers a robust and flexible solution for handling date and time in .NET applications, addressing many of the limitations and ambiguities present in the standard DateTime types.