Assume you run an online store and, every month, you send a marketing email to consumers who have registered on your site highlighting the latest products from your store in order to increase sales. Your CEO, on the other hand, wants to know how many customers actually open these emails. If you can't pay third-party email tracking solutions, you can utilize the following way to see if your emails have been opened. Create an API first. If our customers open our emails, this API will record the messages they send back.
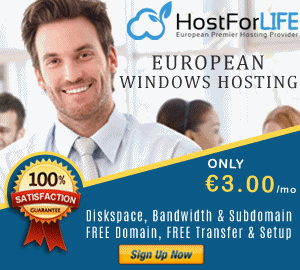
[ApiController]
[Route("api/[controller]")]
public class EmailTrackingController : Controller
{
[HttpGet("EmailOpener")]
public ActionResult EmailOpener(string email, string eventId)
{
//Your logic here to capture email & eventId
//maybe save email and eventID to DB
byte[] pixel = Convert.FromBase64String("R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7");
return new FileContentResult(pixel, "image/gif");
}
}
As you can see, I've built an EmailTracking controller with a GET method called EmailOpener that takes two parameters: email and eventId.
When a customer opens your marketing email, this API is invoked, and their email address, along with the eventId, is provided to it. The email and eventId can then be captured and saved in your database. You might have noticed that I returned the encoded value "R0lGODlhAQABAIAAAAAAAP///yH5BAEAAAAALAAAAAABAAEAAAIBRAA7."
This isn't just any dummy value; it's a legitimate base64-encoded representation of a tiny transparent GIF image. Assume the short HTML text below is your marketing email for the forthcoming Black Friday Sales.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Black Friday Sales</title>
</head>
<body>
<h1>Hi, check out our Black Friday Sales!!!</h1>
<img alt="" src="https://www.mysite.com/EmailTracking/EmailOpener?email=ENCODED_EMAIL_ADDRESS&eventId=BlackFridaySales2023" width="1" height="1" style="border: none; display: block;" />
<!-- Main content images -->
<img src="https://www.mysite.com/contents/image/BFD2023.PNG" alt="Black Friday Deals" />
<!-- Dummy messages -->
<p>Dummy Message....</p>
<p>Dummy Message....</p>
<p>Dummy Message....</p>
</body>
</html>
As you can see in the email, I have included the following html snippet
<img alt="" src="@Email&eventId=BlackFridaySales2023">https://www.mysite.com/EmailTracking/EmailOpener?email=@Email&eventId=BlackFridaySales2023" width="1" height="1" style="border-width: 0px; border-style: solid;" />
This is an HTML image tag with a 1x1 pixel size that contains a link to our EmailOpener API. Because of its small size, the buyer will overlook it when they read our marketing email. As you can see, I used @Email for the email field; this is a variable that will be substituted with the email address of the real recipient.
When a customer reads your email, the 1x1 pixel picture loads and the URL (in this case, "https://www.mysite.com/EmailTracking/EmailOpener?email=@Email&eventId=BlackFridaySales2023") is triggered. This enables us to determine who opened the email and for what event.
Redirect Image Clickable
Some email providers, however, automatically prevent photos from loading and only display the images if the user chooses to view or download them. If this occurs, our 1x1 pixel image will not work because our consumers will not see it in the email, let alone download it.
To avoid this problem, we can include an appealing image in the email along with a call-to-action such as 'Click the image to discover more amazing deals!' We can redirect them to our API and store their email address in our database whenever they click the image.
To help with this, I've included a new API method called RedirectCustomer.
public ActionResult RedirectCustomer(string email, string eventId)
{
//Your logic here to capture email & eventId
//maybe save email and eventID to DB
if (eventId == "BlackFridaySales2023")
{
return Redirect("https://www.mysite.com/BFD2023LandingPage");
}
else {
return Redirect("https://www.mysite.com");
}
}
This function will record the customer's incoming email and route them to the relevant page based on the incoming eventID. If we notice an incoming client with the eventID 'BlackFridaySales2023,' for example, we'll redirect them to our Black Friday Promotions landing page.
Include the following html image element in the email you send to the customer.
<a href="https://www.mysite.com/EmailTracking/RedirectCustomer?email=@email&eventId=BlackFridaySales2023">
<img alt="Black Friday Promotion 2023" class="fullWidth" src="https://www.mysite.com/Content/images/BFD2023.png" style="display: block; height: auto; border: 0px; max-width: 600px; width: 100%;" title="Black Friday Promotion 2023!" width="600" />
</a>
An image entitled 'BFD2023.png' will be prominently shown in the email sent to our consumers. When the customer clicks on this image, they will be taken to our API at https://www.mysite.com/EmailTracking/RedirectCustomer?email=@Email&eventId=BlackFridaySales2023. At this point, the customer's email address will be saved in our database. This information allows us to track the email open rate, which can then be examined after the offer is over to determine the campaign's reach and effectiveness.
Some Things to Think About
If you intend to service a large number of customers, you must address potential concerns such as database locks, concurrency, and server overload. It is critical to guarantee that your infrastructure can manage a high amount of incoming requests. Furthermore, further coding steps are required to manage concurrency and prevent database locking from a programming standpoint.