
February 18, 2020 10:54 by
Peter
Web APIs have some common parameters in a project, mabybe those paramters should be passed via header or query, etc.For example, there is a Web API url, https://yourdomain.com/api/values, and when we access this Web API, we should add timestamp, nonce and sign three parameters in the query.
It means that the url must be https://yourdomain.com/api/values?timestamp=xxx&nonce=yyy&sign=zzz
Most of the time, we will use Swagger as our document. How can we document those parameters in Swagger without adding them in each action?
Here I will use ASP.NET Core 3.1 to introduce the concept.
How to do this?
Create a new Web API project, and edit the csproj file, add the following content in it.
<ItemGroup>
<PackageReference Include="Swashbuckle.AspNetCore" Version="5.0.0" />
</ItemGroup>
<PropertyGroup>
<GenerateDocumentationFile>true</GenerateDocumentationFile>
<NoWarn>$(NoWarn);1591</NoWarn>
</PropertyGroup>
Swashbuckle provides a feature named operation filter that can help us to do that job.
We can add those three additional parameters in our custom operation filter, so that we do not need to add them in each action.
Here is the sample code demonstration.
using Microsoft.AspNetCore.Mvc.Controllers;
using Microsoft.OpenApi.Models;
using Swashbuckle.AspNetCore.SwaggerGen;
using System.Collections.Generic;
public class AddCommonParameOperationFilter : IOperationFilter
{
public void Apply(OpenApiOperation operation, OperationFilterContext context)
{
if (operation.Parameters == null) operation.Parameters = new List<OpenApiParameter>();
var descriptor = context.ApiDescription.ActionDescriptor as ControllerActionDescriptor;
if (descriptor != null && !descriptor.ControllerName.StartsWith("Weather"))
{
operation.Parameters.Add(new OpenApiParameter()
{
Name = "timestamp",
In = ParameterLocation.Query,
Description = "The timestamp of now",
Required = true
});
operation.Parameters.Add(new OpenApiParameter()
{
Name = "nonce",
In = ParameterLocation.Query,
Description = "The random value",
Required = true
});
operation.Parameters.Add(new OpenApiParameter()
{
Name = "sign",
In = ParameterLocation.Query,
Description = "The signature",
Required = true
});
}
}
}
NOTE
For showing the difference, we only add those parameters whose controller name does not start with Weather.
By the way, if you have any other parameters or conditions, add them yourself.
Then, we will configure Swagger in Startup class.
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddSwaggerGen(c =>
{
// sepcify our operation filter here.
c.OperationFilter<AddCommonParameOperationFilter>();
c.SwaggerDoc("v1", new OpenApiInfo
{
Version = "v1.0.0",
Title = $"v1 API",
Description = "v1 API",
TermsOfService = new Uri("https://www.c-sharpcorner.com/members/catcher-wong"),
Contact = new OpenApiContact
{
Name = "Catcher Wong",
Email = "[email protected]",
},
License = new OpenApiLicense
{
Name = "Apache-2.0",
Url = new Uri("https://www.apache.org/licenses/LICENSE-2.0.html")
}
});
});
// other....
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseSwagger(c =>
{
});
app.UseSwaggerUI(c =>
{
c.SwaggerEndpoint("/swagger/v1/swagger.json", "v1 API");
});
// other....
}
}
Here is the result.
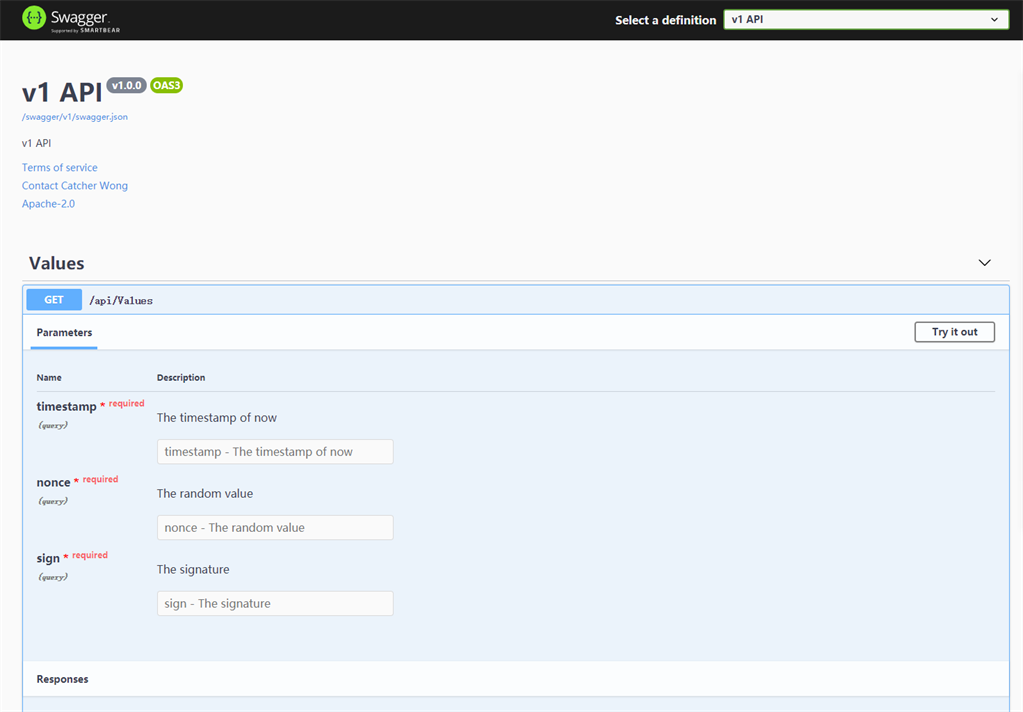
The controller name that doesn't start with `Weather` will contain those three parameters.
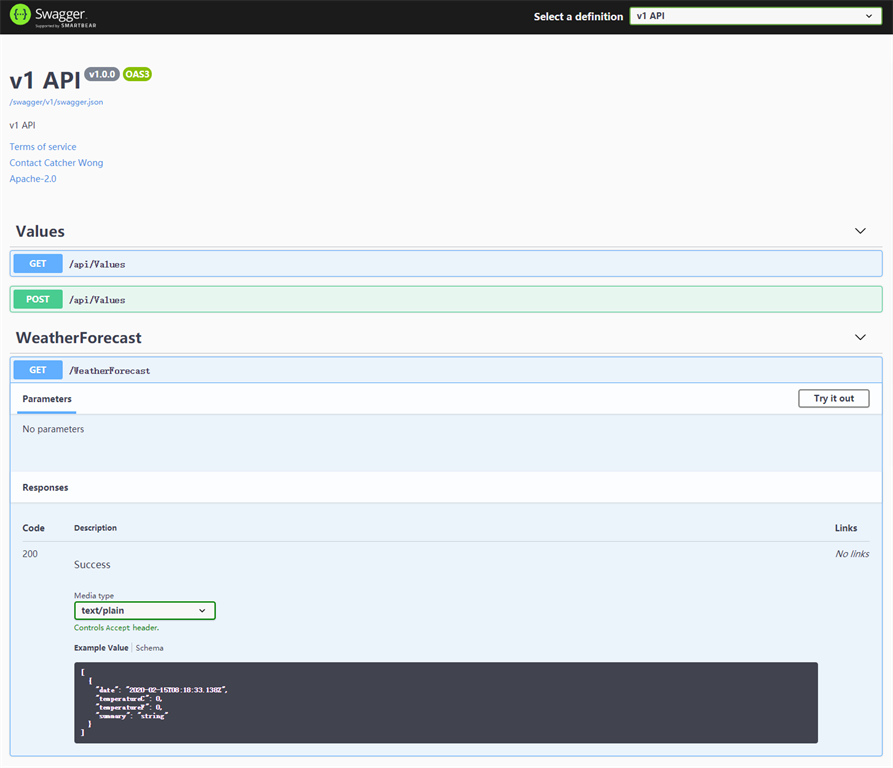
This article showed you a sample of how to add custom request parameters in Swagger using ASP.NET Core 3.1 and Swashbuckle.AspNetCore 5.0.0.
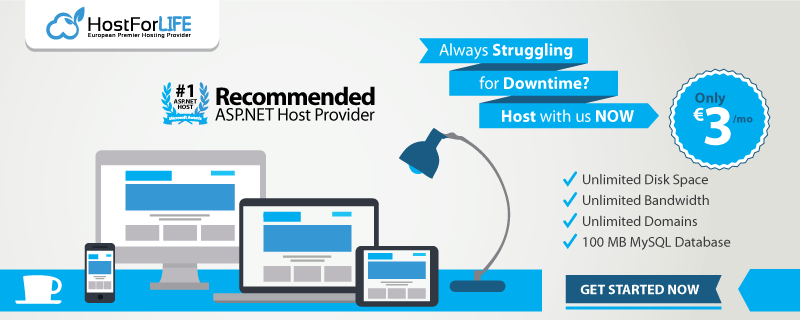