
June 20, 2024 13:52 by
Peter
In software development, the introduction of new features often involves a period of testing and feedback. During this period, developers need a way to indicate that certain features are not fully mature and may be subject to change. The Experimental attribute in C# serves this purpose by marking classes, methods, or other members as experimental. This blog will delve into what the Experimental attribute is, why it's useful, how to implement it, and provide detailed code snippets to illustrate its usage.
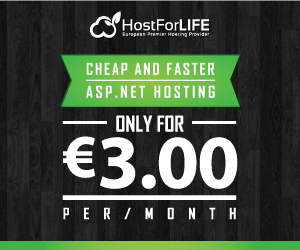
What is the Experimental Attribute?
The Experimental attribute is a custom attribute that can be applied to various members of your codebase (e.g., classes, methods, properties). It indicates that the marked feature is experimental, meaning it's in a testing phase and may undergo significant changes or might even be removed in future releases.
Why Do We Need It?
- Communication: It communicates to other developers that the marked feature is not yet stable and should be used with caution.
- Encapsulation: It helps encapsulate features that are still under development, making it easier to manage their lifecycle.
- Documentation: It serves as a form of documentation, indicating the experimental nature directly in the code.
Implementing the Experimental Attribute
Step 1. Define the Experimental Attribute
First, you need to define the Experimental attribute. This is a simple custom attribute class.
using System;
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method | AttributeTargets.Property)]
public class ExperimentalAttribute : Attribute
{
public string Message { get; }
public ExperimentalAttribute(string message)
{
Message = message;
}
}
Step 2. Applying the Experimental Attribute
You can apply the Experimental attribute to various parts of your codebase to indicate that they are experimental.
using System;
[Experimental("This class is experimental and may change in the future.")]
public class ExperimentalFeature
{
[Experimental("This method is experimental and may change in the future.")]
public void ExperimentalMethod()
{
Console.WriteLine("This is an experimental method.");
}
[Experimental("This property is experimental and may change in the future.")]
public string ExperimentalProperty { get; set; }
}
Step 3. Using the Experimental Features
When using the experimental features, it’s important to handle them with care, knowing that their behavior might change.
public class Program
{
public static void Main()
{
ExperimentalFeature feature = new ExperimentalFeature();
feature.ExperimentalMethod();
feature.ExperimentalProperty = "Testing";
Console.WriteLine(feature.ExperimentalProperty);
}
}
Detailed Explanation of Code Snippets
Defining the Attribute
[AttributeUsage(AttributeTargets.Class | AttributeTargets.Method | AttributeTargets.Property)]
public class ExperimentalAttribute : Attribute
{
public string Message { get; }
public ExperimentalAttribute(string message)
{
Message = message;
}
}
- [AttributeUsage]: Specifies the program elements to which the attribute can be applied. Here, it can be applied to classes, methods, and properties.
- Constructor: Initializes the attribute with a message that provides additional context or warnings about the experimental feature.
Applying the Attribute
[Experimental("This class is experimental and may change in the future.")]
public class ExperimentalFeature
{
[Experimental("This method is experimental and may change in the future.")]
public void ExperimentalMethod()
{
Console.WriteLine("This is an experimental method.");
}
[Experimental("This property is experimental and may change in the future.")]
public string ExperimentalProperty { get; set; }
}
- Class Level: Marks the entire class as experimental.
- Method Level: Marks a specific method within the class as experimental.
- Property Level: Marks a property within the class as experimental.
Using the Experimental Features
public class Program
{
public static void Main()
{
ExperimentalFeature feature = new ExperimentalFeature();
feature.ExperimentalMethod();
feature.ExperimentalProperty = "Testing";
Console.WriteLine(feature.ExperimentalProperty);
}
}
Creating an Instance: An instance of the ExperimentalFeature class is created and its experimental method and property are used. This is straightforward, but developers should be aware of the experimental status.
Conclusion
The Experimental attribute in C# is a powerful tool for marking features as experimental, providing clear communication to other developers about the stability and maturity of the code. By defining and applying this custom attribute, you can better manage the development and evolution of new features, ensuring that everyone involved in the project is aware of their experimental nature.
Using the Experimental attribute helps in documenting and encapsulating the experimental features effectively, making your codebase more maintainable and understandable. While the examples provided are simple, the approach can be scaled to larger and more complex projects, enhancing the overall development process.